[Python #5] Learning Python with Selenium - Class [KR]
지난번 함수에 이어 클래스를 알아본다. 'class 클래스명:' 으로 시작 후 함수를 들여쓰기하여 넣어주면 되고 필요할 때 이 클래스 객체를 생성 후 함수 호출을 하면 된다.
class Steemit:
def login(self):
... ...
... ...
그런데 파이썬은 java와 달리 클래스가 필수는 아니다. 클래스를 없이 함수만으로도 충분히 사용이 가능하다. 실제로 만들어보니 그냥 함수를 만들때보다 먼가 많이 복잡하다.
음? 그럼 왜 클래스를 사용하는가?
좀 찾아보니 여러가지 이유가 있는데 그 중 내 실력으로 이해 가능한 애들만 우선 정리해 본다.
- 클래스는 요술 메서드라고 하는 고유 메서드가 많다. 예를 들면 java의 생성자 역할을 하는
__init__
메서드가 있겠다. 메서드명 앞뒤로 언더스코어가 두개씩 있는데 이를 '던더'라고 한다. 이런 요술 메서드를 오버라이딩해서 더 많은 기능을 확장할 수 있다. - with 키워드와 같이 사용할 수 있다. 파일 처리하는 스크립트를 작성할 때 항상 파일을 열고, 편집하고, 파일을 닫아야 하는데 제일 중요한 스텝인 파일 닫는 작업을 까먹는 경우가 있다. with 키워드로 이런 실수를 막을 수 있다. 요술 메서드중
__enter__
,__exit__
두개를 클래스내에 정의하면 with 키워드와 연동이 가능하다.
그럼 지금까지 작성한 코드를 클래스화 해보자.
Scenario
- Module.py 라는 파일에 Steemit 이라는 클래스와 SteemCoinpan이라는 클래스 생성
- 각각의 클래스에 지금까지 만들어 놓은 login(), vote() 등 함수 입력
- Scenario.py 라는 파일 새로 생성 후 steemit, SteemCoinpan 객체 생성, 초기화한 chrome driver를 인자로 넣어준다.
- steemit의 login() 함수 호출, 또 SteemCoinpan의 login() 함수 호출
Source
Module.py
from selenium import webdriver
import time
from selenium.common.exceptions import NoSuchElementException
class Steemit:
def __init__(self, driver: webdriver) -> None:
self.driver = driver
def login(self):
"""
log in to steemit
"""
self.driver.get('https://steemit.com')
self.driver.find_element_by_xpath('//Header/descendant::a[text()="로그인"]').click()
self.driver.find_element_by_xpath('//input[contains(@name,"username")]').send_keys('june0620')
with open('C:/Users/USER/Desktop/python/june0620.txt') as pw:
for postingKey in pw:
self.driver.find_element_by_xpath('//input[contains(@name,"password")]').send_keys(postingKey)
self.driver.find_element_by_xpath('//button[text()="로그인"]').click()
time.sleep(3)
def vote(self):
pass
def is_post_valid(self, post: webdriver) -> bool:
"""
Check if the post is valid
"""
whitelist = ['xxx']
username = post.find_element_by_xpath('descendant::span[@class="author"]/strong').text
if username in whitelist:
print('white List: ' + username)
return True
if self.is_nsfw(post):
return False
def is_nsfw(self, post: webdriver) -> bool:
"""
Check if the post is nsfw.
"""
try:
post.find_element_by_xpath('descendant::span[text()="nsfw"]')
return True
except NoSuchElementException as err:
return False
class SteemCoinpan:
def login(self):
"""
log in to steemcoinpan
"""
pass
def vote(self):
pass
def is_post_valid(self, post: webdriver) -> bool:
"""
Check if the post is valid
"""
pass
def is_nsfw(self, post: webdriver) -> bool:
"""
Check if the post is nsfw.
"""
pass
Scenario.py:
from selenium import webdriver
from Module import Steemit, SteemCoinpan
# Set chromedriver
chrome = webdriver.Chrome('C:/Users/USER/Desktop/python/chromedriver/chromedriver.exe')
# Set window maximum
chrome.maximize_window()
# Create a steemit object
steemit = Steemit(chrome)
# Call function 'login'
steemit.login()
# Create a steemcoinpan object
span = SteemCoinpan(chrome)
span.login()
chrome.quit()
오늘의 내용 정리
Python:
- 클래스에서 메서드를 만들 때 첫번째 인자는 self라는 키워드를 붙이는게 관례이다. self는 다른 언어의 this와 같은 개념이다.
- 하나의 모듈(파일)에 여러개의 클래스를 만들 수 있다.
- 함수를 명명할 때 소문자에 언더스코어로 강조, 클랙스를 명명할 때 캐멀케이스로 명명한다. 이유는 함수를 호출한 것인지 객체를 생성한 것인지 구분하기 위함이다.
- 클래스에는
__init__
메서드로 객체를 초기화한다. Java의 생성자 개념인 것 같다. - with 문은 클래스와 연결할 수 있고, 파일 처리할 때 사용한다.
Reference:
[Python #4] Learning Python with Selenium - Function [KR]
@tipu curate 1
Posted using Partiko Android
Upvoted 👌 (Mana: 15/20)
!tipu curate 1
Posted using Partiko Android
Thank you so much for participating in the Partiko Delegation Plan Round 1! We really appreciate your support! As part of the delegation benefits, we just gave you a 3.00% upvote! Together, let’s change the world!
Hi帅锅😉
tipu不知道怎么呼叫不了了 刚呼叫几次都没来😅🤦♀️
给你呼叫!shop
系统出问题了吧 😄
Posted using Partiko Android
谢谢
!shop
Posted using Partiko Android
Hi~ 萍萍!
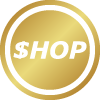
@june0620 has gifted you 1 SHOP!
Currently you have: 104.001 SHOP
View or Exchange
Are you bored? Play Rock,Paper,Scissors game with me!SHOP
Please go to steem-engine.com.Hi~ june0620!
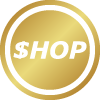
@annepink has gifted you 1 SHOP!
Currently you have: 87 SHOP
View or Exchange
Are you bored? Play Rock,Paper,Scissors game with me!SHOP
Please go to steem-engine.com.june0620, thanks for your kindness to gift SHOP to 1 steemians, you have been received a 29.41% upvote from me~
@tipu curate