[Tutorial]: Ionic App Development: Building the buisness app part 4
Repository: Ionic Framework Github Repository
Software Requirements: Visual Studio Code(Or any preferred editor)
A browser(Preferably Chrome)
What you will learn:
Passing Data through pages using
Events
andmodals
Making Dynamic Angular components
Styling and design methods
Difficulty: Intermediate
Tutorial
In the last post we did quite a lot for our development by theming our app and adding a few lists and functionalities. Today we will be binding more elements to our inputs using Angular and learning how to pass useful data through pages. In ionic they are four major methods of passing data through pages. They are:
- Pushing using
navCtrl
- Using
Modals
- Using
Providers
- and using
events
In this tutorial we will be using two which are the events and modals.
Modals are usually used for editing components. For example if you're on a list and want to edit the content of one of the list items, a modal would be the best way to pass data to the editing page and pass the edited data back to your original page.
Note: Immediately the modal is dismissed, the modal is destroyed.
Before we get into that lets go to our start page and bind the balance to our updated balance using angular.
Ionic has angular inbuilt so unlike a normal angular project you can simply define the variables in the .ts
file for that page and directly refer to it using the ngModel
directive.
Our code should look like this
home.ts
import { Component } from '@angular/core';
import { NavController, Events } from 'ionic-angular';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
public balance: number= 0;//Simply define a preset value for your balance using typescript
home.html
<ion-header>
<ion-toolbar>
<ion-title>
<div class="pageheader">The Buisness App</div>
</ion-title>
</ion-toolbar>
</ion-header>
<ion-content padding:10px class="background">
<ion-card>
<ion-card-header>
<div text-center class="balanceheading">
<h1>
BALANCE
</h1>
</div>
</ion-card-header>
<hr>
<ion-card-content>
<div text-center class="balancecontent">
Your current account balance is
</div><br>
<div text-center class="balance_value">
{{ balance | currency : "N" }}//Simply refer to your variable like this using our angular directive.
</div>
So now we can progress to our products page. With the knowledge of styling from the last tutorial you should understand the styling the .html
file below.
products.html
<ion-header>
<ion-navbar>
<ion-title>Products</ion-title>
</ion-navbar>
</ion-header>
<ion-content class="background" padding:10px>
<ion-card>
<ion-card-header>
Manage your products
</ion-card-header>
<ion-card-content>
<p>The total selling price of your good is:<br> {{ balance | currency : "N" }}</p>
Here you can manage all the products in your store and keep accurate count of everything bought to maximize profits
</ion-card-content>
</ion-card>
<button ion-button full (click)="createNewProduct()">
Add a new product
</button>
<ion-card>
<ion-list inset>
<ion-list-header>
Your products
</ion-list-header>
<button ion-item *ngFor="let item of items" (click)="itemSelected(item)">
<ion-icon name="{{ item.type }}"> {{ item.name }}</ion-icon><div text-right>{{ item.sellingprice | currency : "N" }}</div>
<hr>
</button>
</ion-list>
</ion-card>
</ion-content>
But what i mainly want you to take note of is the button
with *ngFor
. This is the method used for iterating through arrays and even for creating infinite content read from a server. The *ngFor directive iterates through an array and repeats the .html
tag its within for every element in the array. In infinite scroll media a server just keep serving data into this array which will continuously displays this in the view.
So with that we can see form this line *ngFor="let item of items"
that the process iterates through an array called items
which is not yet available in our .ts
file. So we will have to head there and create this.
products.ts
import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams, ModalController, Events } from 'ionic-angular';
import { NewproductPage } from '../newproduct/newproduct';
@IonicPage()
@Component({
selector: 'page-products',
templateUrl: 'products.html',
})
export class ProductsPage {
public items:any=[{name: "Hello", type="cafe", price="500"},{name: "Hello", type="cafe", price="500"}]//Use this simple example to test and see that this is working.
Another thing youll notice in the .html
file is that we added a <button (click)="createNewProduct">
which calls a function called create new product. This is where the modal comes in. We are going to create a modal which will add to this items
array. So lets get on to that.
First you create a new page called the newproductPage
using
ionic g page newproduct
Then import it into your app.module.ts
as taught in the last tutorial
Beneath your constructor add this
product.ts
createNewProduct(){
let newItemModal = this.modalCtrl.create(NewproductPage);//This will create new modal with the content of the new product page.
newItemModal.present();//This will present the modal to the viewer
So this will display a blank page for now because we havent added any .html
content.
Head to your newproductPage.html
and style it using this template to gather input
<ion-header>
<ion-navbar>
<ion-title>Add a New Product</ion-title>
</ion-navbar>
</ion-header>
<ion-content class="background" padding>
<ion-list margin:50px>
<ion-list-header primary>What is the name of your product</ion-list-header>
<ion-item>
<ion-label floating>Product Name</ion-label>
<ion-input [(ngModel)]="product.name" type="text"></ion-input>//Bind all values to their variables
</ion-item>
</ion-list>
<br>
<hr>
<ion-list>
<ion-list-header>
What type of product
</ion-list-header>
<ion-item>
<ion-label>Product type</ion-label>
<ion-select [(ngModel)]="product.type">
<ion-option value="cafe">Beverage</ion-option>
<ion-option value="eye">Cosmetic</ion-option>
<ion-option value="phone-potrait">Gadget</ion-option>
<ion-option value="woman">Clothing</ion-option>
<ion-option value="watch">Clothing accesory</ion-option>
<ion-option value="square">Not in options</ion-option>
</ion-select>
</ion-item>
</ion-list>
<br>
<hr>
<ion-list>
<ion-list-header>What is the Cost Price of your product?</ion-list-header>
<p>How much did you buy the product?</p>
<ion-item>
<ion-label floating>Product Price</ion-label>
<ion-input type="number" [(ngModel)]="product.costprice"></ion-input>
</ion-item>
</ion-list>
<br>
<hr>
<ion-list>
<ion-list-header>What is the Selling Price of your product?</ion-list-header>
<p>How much do you sell the product?</p>
<ion-item>
<ion-label floating>Product Price</ion-label>
<ion-input type="number" [(ngModel)]="product.sellingprice"></ion-input>
</ion-item>
</ion-list>
<button ion-button light (click)="savedata()">
Save
</button>
</ion-content>
If you look through this html content you would see that we binded many angular directives to variables that dont exist yet. So well head to the newproductpage.ts
and add the variables.
newproductpage.ts
@IonicPage()
@Component({
selector: 'page-newproduct',
templateUrl: 'newproduct.html',
})
export class NewproductPage {
product: any={name: "",type: "",costprice: 0,sellingprice: 0}//These are the values we want to collect from this page
So now when you save you should see all the nice content on the page.
When the user inputs data he would want to save this data and you would like this data to add to the items
in the products page. That would be done with the saveData()
function that we bound to the save
button in our html file.
To dismiss a modal ionic uses a different import from ionic-angular
called the ViewController
So go to your newproductpage.ts
and import ViewController
and add it to your controller like this.
import { Component } from '@angular/core';
import { IonicPage, NavController, NavParams, ViewController } from 'ionic-angular';
@IonicPage()
@Component({
selector: 'page-newproduct',
templateUrl: 'newproduct.html',
})
export class NewproductPage {
product: any={name: "",type: "",costprice: 0,sellingprice: 0}
constructor(public navCtrl: NavController, public navParams: NavParams, public viewCtrl: ViewController) {
}//This makes the ViewController usable in this page.
So now that we've injected the viewcontroller
its time to use it in our saveData()
function and pass data using it.
Create the new function beneath your controller like this.
savedata(){
if(this.product){
let data = this.product;
this.viewCtrl.dismiss(data); //This is the function that dismisses the modal and passes the data we've collected from the input to the products page.
} else {
this.viewCtrl.dismiss();
console.log("No data input");
}//We do this so that if no data isnt added it wont add a blank value to our products page
}
The final thing we'll be doing today is collecting the data we've collected from this list and adding it to the items
array in our products page.
To do this we add something to the function that create the modal. The function is called onDidDismiss
and it is inbuilt into ionic.
Add this to your createnewproduct
function.
createNewProduct(){
let newItemModal = this.modalCtrl.create(NewproductPage);
newItemModal.onDidDismiss((data) => {
if(data){//This checks if data is actually collected so we wont log blank values to the items array
this.items.unshift(data);//This adds the data collected to the array
this.balance = this.balance + parseInt(data.sellingprice);//This updates our balance record
let balance = this.balance;
let items = this.items
this.events.publish('balance',balance,items);//This creates a new event.
}
else {
console.log('no data received')
}
})
newItemModal.present();
}
We see that all the data we've collected so you can save and test. In the next tutorial we will be dealing with events
that we added to the function and explaining its use and how it will be used in this application.
You can find my code in Github
Thank you for your contribution. Few notes to keep an eye on for future work:
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Well Noted,
At the end of the tutorial I realized I had already used a bit of the
event
but if I had chosen to explain it detailed it would have been twice as long and would probably be too much to take on at once. Also the styling techniques are mostly in the.html
so it wouldn't be too easy to bring out each component and explain but I'll do well to add way more comments in my code in the next tutorial. And lastly theion-option
is an input menu, so you have to explicitly list all the possible values as you would want them to be directly in the.html
. There's no way to loop that.Thank you for your review, @mcfarhat!
So far this week you've reviewed 3 contributions. Keep up the good work!
Hi @yalzeee!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
I upvoted your post.
Mabuhay, keep steeming.
@Filipino
Posted using https://Steeming.com condenser site.
Hey, @yalzeee!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
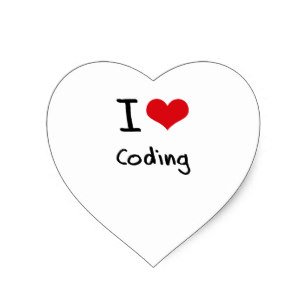
Reply !stop to disable the comment. Thanks!
Hello! I find your post valuable for the wafrica community! Thanks for the great post! We encourage and support quality contents and projects from the West African region.
Do you have a suggestion, concern or want to appear as a guest author on WAfrica, join our discord server and discuss with a member of our curation team.
Don't forget to join us every Sunday by 20:30GMT for our Sunday WAFRO party on our discord channel. Thank you.