Learning to think like a programmer with Python - Fundamentals I
What will I learn?
- Numbers
- Operators
- Variables
- Print () and input () functions
Requirements
- A PC/laptop with any Operating system such as Linux, Mac OSX, Windows OS
Note: This tutorial is performed in Sublime Text 3 in laptop with Windows 7, 32 bit OS, Python 3.6.4.
Difficulty
Basic difficulty, anyone without previous programming knowledge or novices can catch up with this tutorial.
Tutorial Contents
Programming is a tool used to manipulate data automatically, but what data? When I refer to data I am talking about information, information that can be presented in many ways, from simple numbers, texts, sounds, images and even videos, with programming we can manipulate the data in a thousand ways, for example to search for information in registers, to solve complex mathematical operations, to create forms and save the answers, or to draw data from these same graphs, therefore without data the programming it would make no sense, this is the main reason why we have to start there, seeing the basic data types we can handle, in Python we have several, among them are: the numbers, the texts and the lists.
In this post I will talk a little about the numbers, types of operators and variables.
Numbers
The Python interpreter can fulfill the function of a simple calculator, where we enter an expression and it returns the desired result.
Integers and decimals:
Python distinguishes between integers and decimals. When writing a decimal number, the separator between the integer part and the decimal part is a point.
-Interpreter
>>> 7
Output:
7
-Interpreter
>>> 7.5
Output:
7.5
The type () instruction tells us the type of data.
-Interpreter
>>> type(7)
Output:
<class 'int'>
The number 7 is an integer type data.
-Interpreter
>>> type(7.5)
Output:
<class 'float'>
The number 7.5 is an float type data.
Complex numbers: Python allows us to do calculations with complex numbers. The imaginary part is accompanied by the letter "j".
-Interpreter
>>> 1 + 1j + 2 + 3j
Output:
(3+4j)
The letter "j" must always be accompanied by a number.
-Interpreter
>>> 7+j
Output:
Traceback (most recent call last):
File "<pyshell#5>", line 1, in <module>
7+j
NameError: name 'j' is not defined
Basic operations in Python
In Python you can work with four basic arithmetic operations: addition (+), subtraction (-), multiplication (*) and division (/).
When performing operations between integers and decimals, the result is always decimal.
-Interpreter
>>> 7.7 * 2
Output:
15.4
When adding, subtracting or multiplying integers, the result is integer.
-Interpreter
>>> 7+ 2
Output:
9
-Interpreter
>>> 7-2
Output:
5
-Interpreter
>>> 7* 2
Output:
14
When dividing integers, the result is always decimal, even if it is a whole number.
-Interpreter
>>> 7/ 2
Output:
3.5
When you have multiple operations, Python performs them applying the usual rules of priority of operations (first multiplications and divisions, after addition and subtraction).
-Interpreter
>>> 3 + 12 * 3
Output:
39
-Interpreter
>>> 30- 12 * 3
Output:
-6
If you want the operations to be performed in another order, it is recommended to use parentheses.
-Interpreter
>>> (5+20) / (17-5)
Output:
2.0833333333333335
Quotient and rest of a division
The quotient of a division is calculated in Python with the // operator.
-Interpreter
>>> 10 // 3
Output:
3
-Interpreter
>>> 20.0 // 7
Output:
2
The rest of a division is calculated in Python with the operator "%".
-Interpreter
>>> 10 % 4
Output:
2
-Interpreter
>>> 10 % 5
Output:
0
The potency
The potency are calculated with the operator **, taking into account that x ** y = xy:
-Interpreter
>>> 2 ** 8
Output:
256
As we saw earlier Python has a series of operators, these operators are symbols that represent simple calculations, such as addition, subtraction, multiplication, division, etc.
Operator | Description | Example |
---|---|---|
+ | Sum | 6 + 2 = 8 |
- | Subtraction | 6 - 2 = 4 |
* | Multiplication | 6 * 2 = 12 |
** | Exponent | 2 ** 4 = 16 |
/ | Division | 7/2 = 3.5 |
// | Entire division | 7 // 2 = 3 |
% | Module | 7% 2 = 1 |
Value and type of data
The values are one of the basic components with which a program works, such as a letter or a number. Each value is associated with a data type, these basic types are:
- Integer "int"
- Float "float"
- String "str"
- Boolean "bool"
If you are not sure of the type of value, you can ask the Python interpreter with the "type ()" statement. Let's see better this with some examples:
-Interpreter
>>> type(2)
Output:
<class 'int'>
<class 'int'> Is an integer type. The integers are precisely integers like 7, 55, 999, etc.
-Interpreter
>>> type(2.5)
Output:
<class 'float'>
<class 'float> It is a type of floating. These are precisely numbers with decimals such as 7.5 or 10.7.
The words are another type of data called string :
-Interpreter
>>> type('utopian')
Output:
<class 'str'>
<class 'str'> It is a type of data type strings. The strings are placed inside the quotes marks always
Finally, the last data type is Boolean, these represent the logical states, the false and true.
-Interpreter
>>> type(True)
Output:
<class 'bool'>
-Interpreter
>>> type(False)
Output:
<class 'bool'>
Variables
A variable is a space in the computer's memory that gives us a name and we assign a value to it. The variables are fundamental in all programming languages, in Python the variables are easy to manipulate since we do not have to specify what kind of variables it is:
With the assignment statement "=" a variable is assigned a value.
-Interpreter
>>> a = 5
>>> a
Output:
5
Rules for naming a variable
Rule | Description |
---|---|
1 | The name can not start with a number |
2 | The name can not start with a space |
3 | The name does not contain special symbols or contains spaces |
4 | The name can not be a reserved Python word |
The names of the variables can have an arbitrary length. They can be formed by letters and numbers, but they should always start with a letter. It is advisable to always use lowercase, since Python is case-sensitive: Steemit and steemit are two different variables.
The words that are reserved are those that not can be used as variables.
Python has 28 reserved words:
and | continue | else | import | not | raise | for |
---|---|---|---|---|---|---|
assert | def | except | from | in | or | return |
break | del | exec | global | is | pass | try |
class | elif | finally | if | lambda | while |
When the name of a variable leaves us an error, we look for the reserved words and verify that it is not in the list.
The print () function
The print () function allows you to display text on the screen. The text to be displayed is written as an argument to the function:
-Interpreter
>>> print("Hello Utopian! How are you?")
Output:
Hello Utopian! How are you?
The desired message is displayed on the screen.
The input () function
The input () function allows to obtain text written by keyboard. Upon reaching the function, the program waiting for something to be written and press the Enter key, as the following example shows:
Sublime Text
print("Hello Utopian! What is your name?")
name = input()
print(f"Welcome {name}! This is Utopian")
Output:
Hello Utopian! What is your name?
Ramses
Welcome Ramses! This is Utopian
Now that we know the basic input() and print() functions, we can implement somewhat complex programs. For example:
- Write a program that asks for five numbers and write your average. (The average is to divide the sum of several quantities by the number of addends).
Sublime tText
print("CALCULATION OF THE AVERAGE OF 5 NUMBERS")
number_1 = float(input("Write the first number: "))
number_2 = float(input("Write the second number:"))
number_3 = float(input("Write the third number:"))
number_4 = float(input("Write the fifth number:"))
number_5 = float(input("Write the second number:"))
average = (number_1+number_2+number_3+number_4+number_5) / 5
print(f"The average of {number_1}, {number_2}, {number_3}, {number_4} and {number_5}, is {average}")
OUTPUT
CALCULATION OF THE AVERAGE OF 5 NUMBERS
Write the first number: 10
Write the second number:20
Write the third number:30
Write the fifth number:40
Write the second number:50
The average of 10.0, 20.0, 30.0, 40.0 and 50.0, is 30.0
- Write a program that asks for a distance in feet and inches and write that distance in centimeters.
(Remember that one foot is twelve inches and one inch is 2.54 cm.)
Sublime Text
print ("CONVERTER OF FEET AND INCHES TO CENTIMETERS")
feet = float(input("Enter a number of feet:"))
inches = float(input("Enter an amount of inches:"))
centimeter = (feet * 12 + inches) * 2.54
print (f"{feet} feet and {inches} inches are {centimeter} cm")
OUTPUT
CONVERTER OF FEET AND INCHES TO CENTIMETERS
Enter a number of feet:4
Enter an amount of inches:9
4.0 feet and 9.0 inches are 144.78 cm
- Write a program that asks for a number of seconds and write how many minutes and seconds they are.
To convert from seconds to minutes, one division is enough:
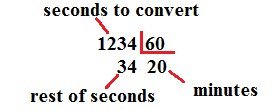
Sublime Text
print ("CONVERTER OF SECONDS TO MINUTES")
seconds = int(input("Enter a number of seconds:"))
minutes = seconds//60
rest = seconds%60
print(f"{seconds} seconds are {minutes} minutes and {rest} seconds")
OUTPUT
CONVERTER OF SECONDS TO MINUTES
Enter a number of seconds:1234
1234 seconds are 20 minutes and 34 seconds
What is the purpose of the Tutorial Learning to think like a programmer with Python?
This tutorial is being developed to teach you how to program in Python from scratch to an intermediate - advanced level. At the time of completion you will be able to create simple software by applying the concepts learned and even create your own graphical interface.
¡Thank you for your attention, until a next post!
Curriculum
Posted on Utopian.io - Rewarding Open Source Contributors
Your contribution cannot be approved because it does not follow the Utopian Rules.
Explanation:
Learn Python Series
myself, you might want to take a look. And I also had to begin with a "part 1" episode: Learn Python Series - Intro ;^^^ I strongly encourage you to have a good look at how I approach teaching Python. If you address your own tutorials in a similar way, I will approve your future contributions.
Good luck!
@scipio
You can contact us on Discord.
[utopian-moderator]
Hey @scipio, I just gave you a tip for your hard work on moderation. Upvote this comment to support the utopian moderators and increase your future rewards!
Interesting, the 2 ** 8 seems like a really nice shortcut to Math.pow