Sort: implementation of sorting algorithms in C and CUDA
sort
Repository of sorting algorithms in C and CUDA.
Proof of work
Information
The program generates and fills arrays in four different ways:
- arrays with totally random elements
- arrays already ordered
- arrays ordered in descending order
- arrays 90% ordered.
Sorting methods implemented
- Selection sort
- Insertion sort
- Shell sort
- Quick sort
- Heap sort
- Merge sort
- CUDA Quick sort
- CUDA Merge sort
Requirements
NVIDIA CUDA Toolkit 6.0, NVCC v6.0.1, GCC and G++
Follow these instructions to set up your environment:
prosciens’s tutorial to set up CUDA 6 compiler environment on Debian testing/sid
Our CUDA sorting code requires devices with CUDA compute capability 3.5 or higher, in order to use
the Dinamic Parallelism technology, read more about it here:
NVIDIA blog describing Dinamic Parallelism in Kepler GPUs
Compiling
Run the MAKEFILE
Instructions
To run the program, type:
./a.out -a $algorithm -n $number_of_elements -s $state [-P]
Parameters
- -a sorting algorithm
- -n number of elements
- -s array state
- -P print results
Param | Value |
---|---|
-a | selection |
insertion | |
shell | |
quick | |
heap | |
merge | |
gpuquick | |
gpumerge | |
-n | int > 0 |
-s | random |
ascending | |
descending | |
almost | |
-P |
Tested
CUDA code tested on a GeForce GT 740M
GeForce GT 740M | Features |
---|---|
CUDA Driver Version / Runtime Version | 6.5 / 6.0 |
CUDA Capability Major/Minor version number: | 3.5 |
Total amount of global memory: | 2048 MBytes (2147352576 bytes) |
( 2) Multiprocessors, (192) CUDA Cores/MP: | 384 CUDA Cores |
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @icaro I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
The fastest sorting algorithm for CUDA I know of is radix sort.
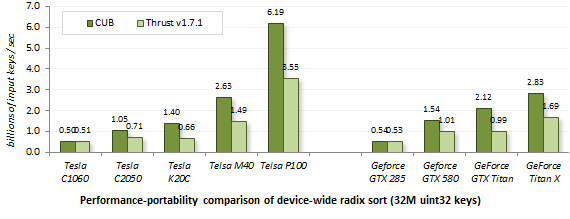
It is included in the CUDA unbound library.