Object Oriented Programming in Java with IntelliJ Part 4
What Will I Learn?
- You will learn abstract class and method in Java
- You will learn interface in Java
Requirements
- Intellij
- Basic knowledge about Java programming language
Difficulty
- Basic
Tutorial Contents
What is Java?
Java is a computer programming language and computing platform.
What is IntelliJ?
IntelliJ is an IDE created that make possible for us to code or develop something useful for people.
What is IDE?
IDE is an Integrated Development Environment that means provide the basic tool for developers to code and test the software. Basicly, it will contain code editor, compiler and debugger.
What is OOP?
OOP stands for Object Oriented Programming. OOP is a programming concept which applies object concept that the object is consisted of attribute and method.
Section 1
Step 1
Install and run IntelliJ which can be downloaded at here or directly from the community at here. Yes, it's officially built by community. That's why it's called as community edition from the official jetbrains web and it's free.
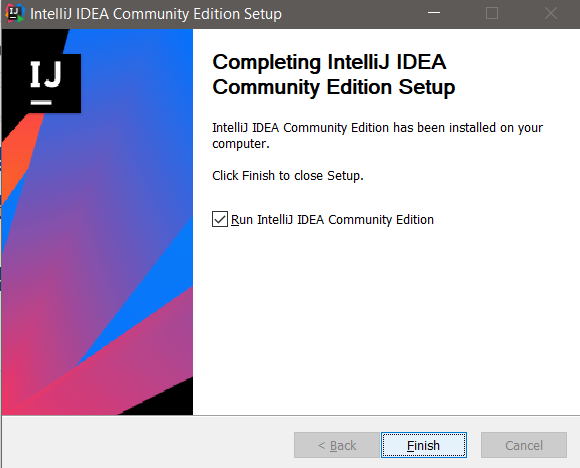
This is the old code from my post. We'll start from this.
public class Score {
private double Quiz,MidExam,LastExam;
public Score(){
Quiz=0;
MidExam=0;
LastExam=0;
}
public Score(double Q, double M, double L){
if(Q>=0 && Q<=100)
Quiz=Q;
if(M>=0 && M<=100)
MidExam=M;
if(L>=0 && L<=100)
LastExam=L;
}
public void setQuiz(double Quiz){
if(Quiz>=0 && Quiz<=100)
this.Quiz=Quiz;
}
public void setMidExam(double MidExam){
if(MidExam>=0 && MidExam<=100)
this.MidExam=MidExam;
}
public void setLastExam(double LastExam){
if(LastExam>=0 && LastExam<=100)
this.LastExam=LastExam;
}
public double getQuiz() {
return this.Quiz;
}
public double getMidExam() {
return this.MidExam;
}
public double getLastExam() {
return this.LastExam;
}
public double getScore(){
return 0.2*Quiz+0.3*MidExam+0.5*LastExam;
}
}
But, before we continue coding, we have to know about the basic knowledge about abstract class and method. We can't create an instance of abstract class or method, because abstract doesn't have a complete implementation. Abstract is created as a function base of subclass. We should extend before we can use it.
Step 2
Add abstract
for Score
class.
Before
public class Score {
After
public abstract class Score {
Complete Codes will be like this.
public abstract class Score {
private double Quiz,MidExam,LastExam;
public Score(){
Quiz=0;
MidExam=0;
LastExam=0;
}
public Score(double Q, double M, double L){
if(Q>=0 && Q<=100)
Quiz=Q;
if(M>=0 && M<=100)
MidExam=M;
if(L>=0 && L<=100)
LastExam=L;
}
public void setQuiz(double Quiz){
if(Quiz>=0 && Quiz<=100)
this.Quiz=Quiz;
}
public void setMidExam(double MidExam){
if(MidExam>=0 && MidExam<=100)
this.MidExam=MidExam;
}
public void setLastExam(double LastExam){
if(LastExam>=0 && LastExam<=100)
this.LastExam=LastExam;
}
public double getQuiz() {
return this.Quiz;
}
public double getMidExam() {
return this.MidExam;
}
public double getLastExam() {
return this.LastExam;
}
public double getScore(){
return 0.2*Quiz+0.3*MidExam+0.5*LastExam;
}
}
When we've done, we extend Score2
class, but on my previous post it should have been done, because we discussed about inheritance.
Complete Codes will be like this.
public class Score2 extends Score {
private double Exercise;
public Score2(){
super();
Exercise=0;
}
public Score2(double Q, double M, double L, double E){
super(Q,M,L);
if(E>=0 && E<=100)
Exercise=E;
}
public void setExercise(double Exercise){
if(Exercise>=0 && Exercise<=100)
this.Exercise=Exercise;
}
public double getExercise() {
return this.Exercise;
}
public double getScore(){
return super.getScore()+0.1*Exercise;
}
}
Now, we want to implement abstract method.
Abstract method must be implemented in the subclass, because in the superclass abstract method is just declared without the statement. Only name of the method and its parameter.
Step 3
Write whatever abstract method you want to, but this is for example. Just add abstract
to the following method without the statement, because this is in superclass or abstract class.
public abstract void sendReport(String to, String subject, String message);
Complete Codes will be like this.
public abstract class Score {
private double Quiz,MidExam,LastExam;
public Score(){
Quiz=0;
MidExam=0;
LastExam=0;
}
public Score(double Q, double M, double L){
if(Q>=0 && Q<=100)
Quiz=Q;
if(M>=0 && M<=100)
MidExam=M;
if(L>=0 && L<=100)
LastExam=L;
}
public void setQuiz(double Quiz){
if(Quiz>=0 && Quiz<=100)
this.Quiz=Quiz;
}
public void setMidExam(double MidExam){
if(MidExam>=0 && MidExam<=100)
this.MidExam=MidExam;
}
public void setLastExam(double LastExam){
if(LastExam>=0 && LastExam<=100)
this.LastExam=LastExam;
}
public abstract void sendReport(String to, String subject, String message);
public double getQuiz() {
return this.Quiz;
}
public double getMidExam() {
return this.MidExam;
}
public double getLastExam() {
return this.LastExam;
}
public double getScore(){
return 0.2*Quiz+0.3*MidExam+0.5*LastExam;
}
}
Step 4
Now we can develop or implement the abstract method in the subclass, Score2
. The method name is the same as what have been declared in Score
class, but without abstract defined. Because, it's already extended and abstract can't have body or statement itself. So, to develop it, the code will be like this.
public void sendReport(String to, String subject, String message){
System.out.println("Send Report\nTo: "+to+"\nSubject: "+subject+"\nMessage: "+message);
}
For Complete Codes, they will be like this.
public class Score2 extends Score {
private double Exercise;
public Score2(){
super();
Exercise=0;
}
public Score2(double Q, double M, double L, double E){
super(Q,M,L);
if(E>=0 && E<=100)
Exercise=E;
}
public void setExercise(double Exercise){
if(Exercise>=0 && Exercise<=100)
this.Exercise=Exercise;
}
public void sendReport(String to, String subject, String message){
System.out.println("Send Report\nTo: "+to+"\nSubject: "+subject+"\nMessage: "+message);
}
public double getExercise() {
return this.Exercise;
}
public double getScore(){
return super.getScore()+0.1*Exercise;
}
}
Step 5
Back to the main or tester class. Write the tester to test how your abstract works or implemented. Fill out with the parameters you've defined before.
For Example :
s2.sendReport("[email protected]","Score","Your Quiz Score: "+s2.getQuiz()+"\nYour Mid Exam Score: "+s2.getMidExam()+"\nYour Last Exam Score: "+s2.getLastExam()+"\nYour Exercise Score: "+s2.getExercise());
The Final Result
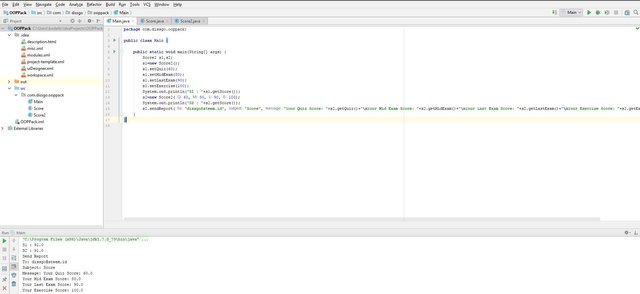
Section 2
Now, I want to tell you about interface in Java.
What is interface?
Interface is a group of abstract methods or a group of methods with empty bodies. All methods in interface should be implemented, while in class, not all of them should be done.
Step 1
Create Interface by right-click on the package, New>Class
Select Interface
on the Kind
field.
Step 2
Create new class again and name it as you want to.
When you've done, implement your class to the interface we've created before.
public class Quiz implements FinalScore
Complete codes will be like this.
Step 3
Now, back to FinalScore
interface which has been made. Declares whatever you want to implement. If I want to know final score for quiz, the complete codes will be like this.
public interface FinalScore {
double getQuiz();
}
Step 4
Now, get back to Quiz
class and write or develop the methods. For example, I want to know what's my total score for Quiz and what's the final score. totalquiz
is how much the quiz score in total and return totalquiz/4;
is how we calculate the final quiz score, because we have 4 times a semester for one subject. So, the complete codes will be like this.
public class Quiz implements FinalScore {
public double totalquiz;
public double getQuiz(){
return totalquiz/4;
}
}
Step 5
Write for testing the interface you've implemented. As always, create new Object of your class you created before in this tester class first, because It will refer as an instance of every object created, Quiz q=new Quiz();
. q.totalquiz=340;
means we set the value for totalquiz
which has been created before. System.out.println("Total Score Quiz: "+q.totalquiz+"\nFinal Score Quiz: "+q.getQuiz());
is the output to be displayed.
Complete codes will be like this.
public class Main {
public static void main(String[] args) {
Quiz q=new Quiz();
q.totalquiz=340;
System.out.println("Total Score Quiz: "+q.totalquiz+"\nFinal Score Quiz: "+q.getQuiz());
}
}
The Final Result
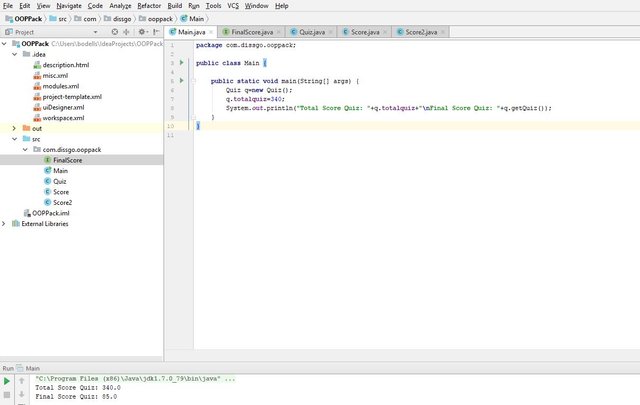
Curriculum
- Object Oriented Programming in Java with Intellij Part 1
- Object Oriented Programming in Java with Intellij Part 2
- Object Oriented Programming in Java with Intellij Part 3
Posted on Utopian.io - Rewarding Open Source Contributors
Intellij is my favorit
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Thanks for approval @manishmike10
Hey @dissgo I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x