SLC-S23W4 - Frontend & React - Candidate Evaluation Platform
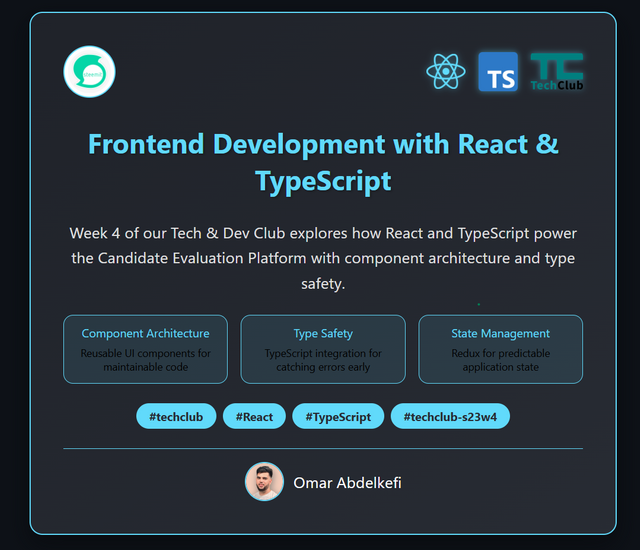
Welcome to Week 4 of Tech & Dev Club
We are now in week 4 of our club. We talked about project planning before. We also learned about methodologies. We learned about docker too. Now we will learn about frontend development. We will use React and TypeScript. These tools helped us make the Candidate Evaluation Platform.
What you will learn:
- Basic React ideas and how to make components
- How to use TypeScript with React
- How to manage state with Redux
- How to organize your project so it can grow
- Real examples from our TalentLens project
React: the foundation of modern UIs
React is a JavaScript library developed by Facebook that enables developers to build user interfaces through reusable components, the component ased architecture makes it easier to maintain and scale applications.
Key React Ideas in Our Project:
1. using something called functional components with hooks:
Example from DisplayCandidates.tsx
const DisplayCandidates = ({idTest=""}) => {
const dispatch = useDispatch();
const [dataSource, setDataSource] = useState<any>([]);
const [value, setValue] = useState('');
const [data, setData] = useState([]);
useEffect(() => {
// Component lifecycle logic
}, []);
// Component rendering logic
};
2. Props and component composition
The McaQuestion
component demonstrates how props are used to pass data between components:
const McaQuestion = ({
quizName,
questionIndex,
totalQuestion,
currentQuestion,
handleCheckChange,
handleRadioChange,
handleSubmit,
lastUpdate,
disabled
}: any) => {
// Component rendering
};
TypeScript: adding type safety to react
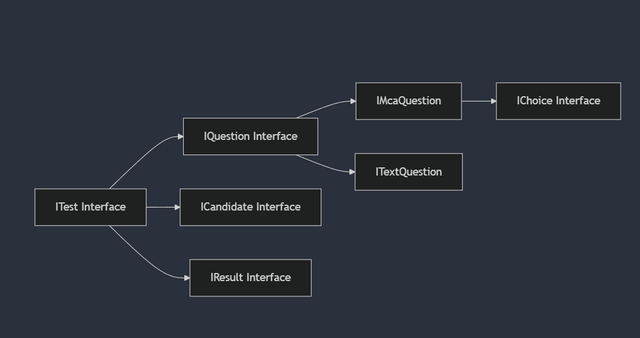
TypeScript Type Relationships (using mermaid)
TypeScript enhances javascript by adding static type definitions, making code more robust and easier to maintain, In this project, it helps catch type related errors before runtime.
Example of TypeScript interfaces:
// From components/questions_types/McaQuestions/McaQuestions.tsx
interface Ichoice {
id: string,
choice_text: string,
is_correct: boolean
}
interface Iquestion {
name: string,
body: string,
choices: Array<Ichoice>,
time: number,
type: string,
skills: Array<string>
}
Redux: State Management for Complex Applications
Redux provides a predictable state container for JavaScript applications, It helps manage global state across components in a structured and maintainable way.
Redux architecture in TalentLens
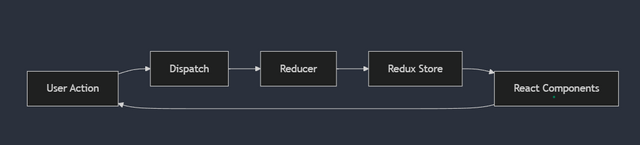
Redux Data Flow (using mermaid)
The application uses the classic Redux architecture:
- Actions: Define what happened ( quiz start, answer submission).
- Reducers: Specify how state changes in response to actions.
- Store: Holds the application state.
Actions Example
From redux/actions/quiz.tsx
:
export function startQuiz(idTestCandidate: any) {
return (dispatch: any) => {
dispatch(request(true));
service.startTest.startTest(idTestCandidate)
.then(
(res: any) => {
dispatch(success(res.data.data, false));
},
(res: any) => {
dispatch(failure(res.error, false));
}
);
};
function request(loading: boolean) {
return { loading, type: quizConstants.START_QUIZ_REQUEST };
}
function success(testInfo: any, loading: boolean) {
return { testInfo, loading, type: quizConstants.START_QUIZ_SUCCESS };
}
function failure(error: any, loading: boolean) {
return { error, loading, type: quizConstants.START_QUIZ_FAILURE };
}
}
Using Redux in Components
The useDispatch
and useSelector
hooks connect components to the Redux store:
// From Quiz.tsx
const dispatch = useDispatch();
const quiz = useSelector((state: any) => state.quiz.quiz);
const currentQuestion = useSelector((state: any) => state.quiz.testInfo.current_question);
// Dispatch an action
dispatch(startQuiz(idTestCandidate));
Project Structure
The TalentLens project follows a well-organized structure that separates concerns and promotes maintainability:
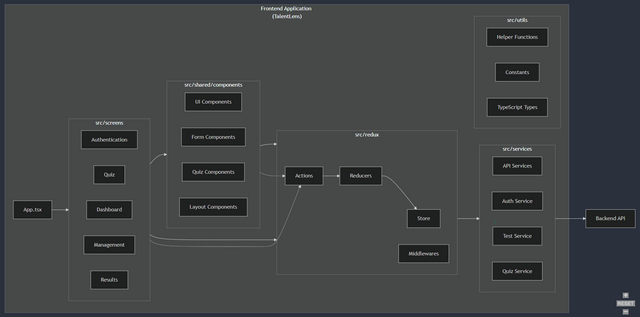
created with mermaid
This structure separates UI components from business logic and makes it easier to navigate and maintain the codebase.
Key Features Implemented
1. Test Management System
The DisplayQuestion.tsx
component allows administrators to manage test questions:
useEffect(() => {
dispatch(setCurrentScreen('3'));
service.baseApiParams.headers = { Authorization: 'Bearer ' + localStorage.getItem('token') };
service.questions.questionsList().then(
(questions: any) => {
setQuestions(questions.data.data);
setDataSource(questions.data.data);
},
(data: any) => {
if (data.error.error === 'token invalid') {
history.push('/403');
}
}
);
}, []);
2. Quiz Taking Interface
The Quiz.tsx
component provides a user-friendly interface for candidates to take tests:
return (
<>
{isLoading ? <></> :
<div className={'quiz__container'}>
<McaQuestion
quizName={quiz.name}
questionIndex={currentQuestion + 1}
totalQuestion={quiz.questions.length}
handleSubmit={handleSubmit}
handleCheckChange={handleCheckChange}
currentQuestion={quiz.questions[currentQuestion]}
lastUpdate={Date.parse(lastUpdate)}
disabled={(loadingTest || isLoading)}
handleRadioChange={handleRadioChange}
/>
</div>
}
</>
);
3. Results Display
After completing a test, candidates see their results through the FinishQuiz.tsx
component:
return (
<div className={'finish-quiz__container'}>
{result.test_status === 'failed' ?
<div>
<div style={{textAlign: 'center' }}>Sorry !</div>
<div style={{textAlign: 'center'}}>
Your total score for the test was {result.score} %.
</div>
</div>
:
<div>
<div style={{textAlign: 'center'}}>Congratulation !</div>
<div style={{textAlign: 'center'}}>Your total score for the test was {result.score} %.</div>
<div style={{textAlign: 'center'}}>we will contact as soon as possible</div>
</div>
}
</div>
);
What I Learned from This Project
Developing the TalentLens platform taught me several valuable lessons:
Component Design: Breaking the UI into small, reusable components makes the code more maintainable and easier to test.
State Management: Redux greatly simplifies state management in complex applications, though it requires careful planning.
TypeScript Integration: TypeScript catches type errors early in the development process, saving debugging time later.
Responsive Design: Using Ant Design components and custom CSS helps create responsive interfaces that work on different devices.
API Integration: Structuring API calls in service modules keeps the code clean and makes it easier to manage backend interactions.
Next Steps
In Week 5, we'll explore database management with SQL and ORM tools, showing how the frontend connects with the backend to store and retrieve data. We'll see how the React components we've examined this week interact with API endpoints to create a complete application.
Stay tuned for next week's topic: Database Management with SQL & ORM.
GitHub Repositories
- Frontend: TalentLens
- Backend: Candidly
Project Resources
- Complete System Overview: Google Drive Link
- Project Presentation Video: Watch Here
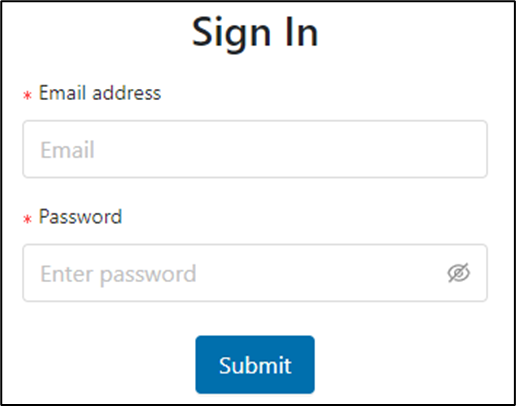
Authentication interface
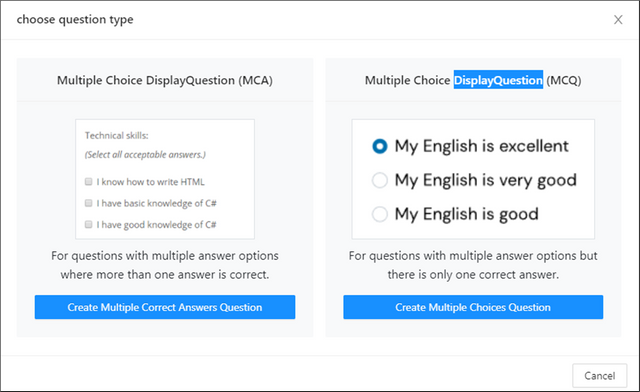
Question type selection interface
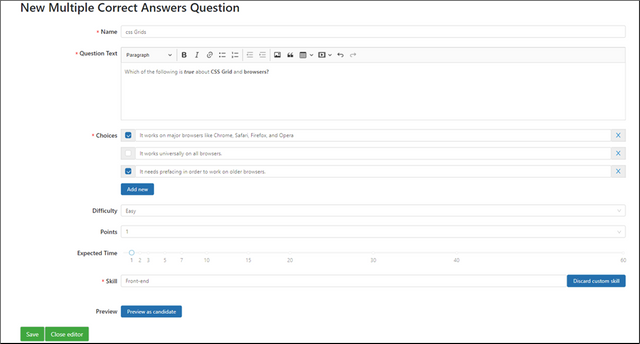
Interface for adding questions to a test
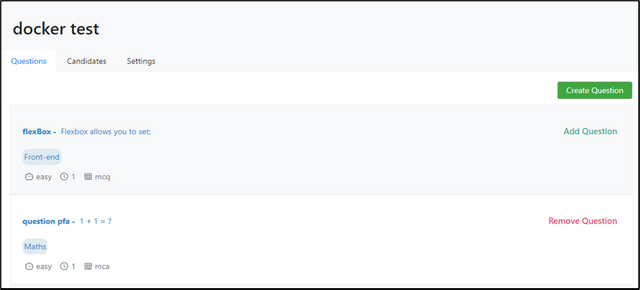
Interface for adding an MCQ-type question
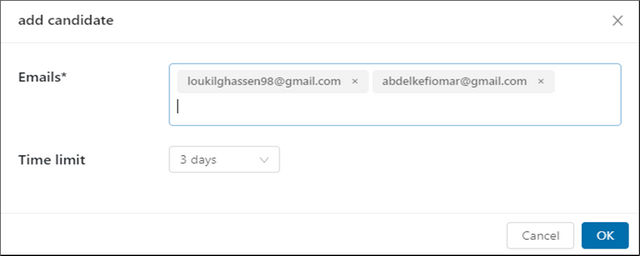
Interface for inviting a candidate to a test
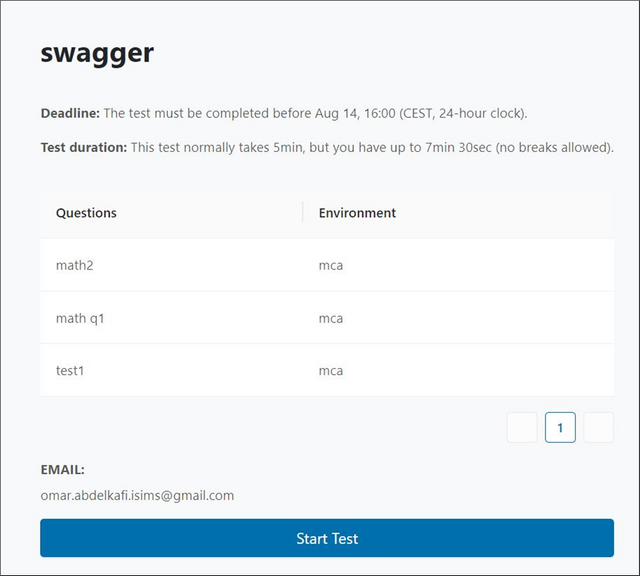
Candidate welcome interface
--
GitHub Repositories
- Frontend: TalentLens
- Backend: Candidly
Project Resources
- Complete System Overview: Google Drive Link
- Project Presentation Video:
What can you share in the club?
Our club is all about technology and development including:
- Web & Mobile Development
- AI & Machine Learning
- DevOps & Cloud Technologies
- Blockchain & Decentralized Applications
- Open-source Contributions
- Graphic Design & UI/UX
Any posts related to technology, reviews, information, tips, and practical experience must include original pictures, real-life reviews of the product, the changes it has brought to you, and a demonstration of practical experience
The club is open to everyone. Even if you're not interested in development, you can still share ideas for projects, and maybe someone will take the initiative to work on them and collaborate with you. Don’t worry if you don’t have much IT knowledge , just share your great ideas with us and provide feedback on the development projects. For example, if I create an extension, you can give your feedback as a user, test the specific project, and that will make you an important part of our club. We encourage people to talk and share posts and ideas related to Steemit.
For more information about the #techclub you can go through A New Era of Learning on Steemit: Join the Technology and Development Club. It has all the details about posting in the "#techclub" and if you have any doubts or needs clarification you can ask.
Our Club Rules
To ensure a fair and valuable experience, we have a few rules:
- No AI generated content. We want real human creativity and effort.
- Respect each other. This is a learning and collaborative space.
- No simple open source projects. If you submit an open source project, ensure you add significant modifications or improvements and share the source of the open source.
- Project code must be hosted in a Git repository (GitHub/GitLab preferred). If privacy is a concern, provide limited access to our mentors.
- Any post in our club that is published with the main tag "#techclub" please mention the mentors @kafio @mohammadfaisal @alejos7ven
- Use the tag #techclub, #techclub-s23w4, "#country", other specific tags relevant to your post.
- In this thirdweek's "#techclub" you can participate from Monday, March 3, 2025, 00:00 UTC to Sunday, March 09, 2025, 23:59 UTC.
- Post the link to your entry in the comments section of this contest post. (Must)
- Invite at least 3 friends to participate.
- Try to leave valuable feedback on other people's entries.
- Share your post on Twitter and drop the link as a comment on your post.
Each post will be reviewed according to the working schedule as a major review which will have all the information about the post such as AI, Plagiarism, Creativity, Originality and suggestions for the improvements.
Other team members will also try to provide their suggestions just like a simple user but the major review will be one which will have all the details.
Rewards System
Sc01 and Sc02 will be visiting the posts of the users and participating teaching teams and learning clubs and upvoting outstanding content. Upvote is not guaranteed for all articles. Kindly take note.
Each week we will select Top 4 posts which has outperformed from others while maintaining the quality of their entry, interaction with other users, unique ideas, and creativity. These top 4 posts will receive additional winning rewards from SC01/SC02.
Note: If we find any valuable and outstanding comment than the post then we can select that comment as well instead of the post.
Technology and Development Club Team
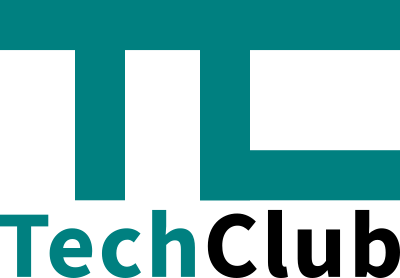
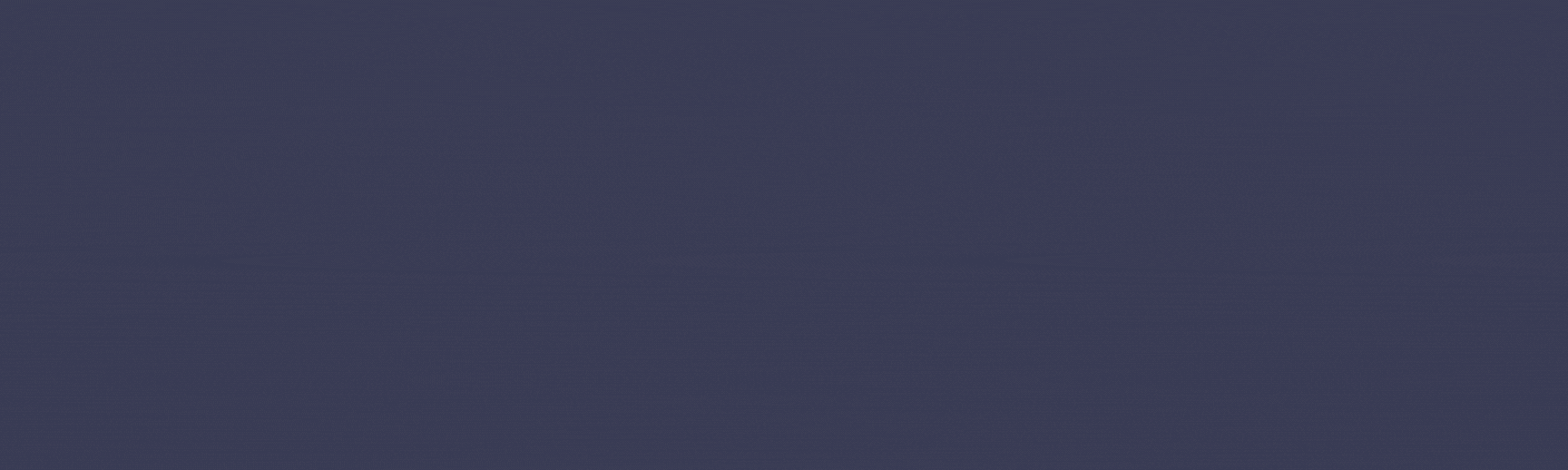
Upvoted! Thank you for supporting witness @jswit.
My entry link is here:
https://steemit.com/steem-for-bangladesh/@azit1980/steemit-learning-club-s23w4-graphic-design-unique-vertical-business-card-design
My Participation,
https://steemit.com/techclubs23w4/@faran-nabeel/steemit-learning-club-s23w2-or-new-feature-in-tradingboard-to-calculate-trade-s-risk-reward-profit-and-position-liquidation