SLC-S23W3 - Docker & Devops - Candidate Evaluation Platform
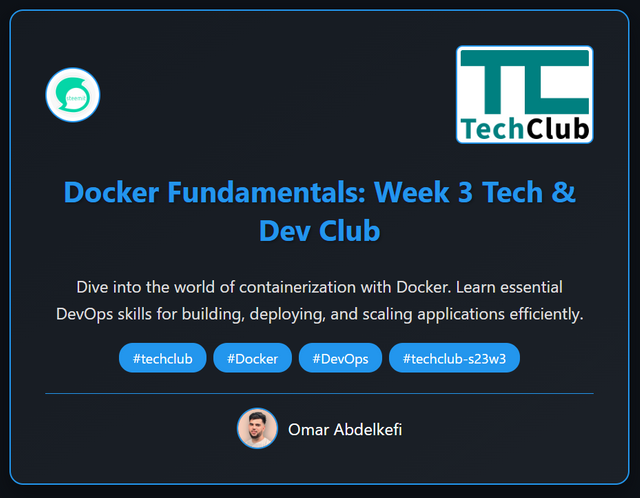
Welcome to Week 3 of our Tech & Dev Club learning series. After covering project planning, UML diagrams, and methodologies last week, we're now diving into the world of containerization with Docker. This is a crucial DevOps skill that will help you build, deploy, and scale applications more efficiently.
What you'll Learn.
- What Docker is and why it is useful
- Basic Docker parts like images and containers
- How to make Dockerfiles
- How to use Docker Compose for many containers
- Looking at real Dockerfiles from our project
What is docker?
Docker is a platform that helps developers put applications in containers. Containers are like small boxes that hold all the app needs. This makes the app work the same on all computers.
Why docker is good?
- No more "
works on my machine" problems - Apps don't mess with each other
- Containers are small and fast
- Easy to make more copies when needed
- Makes testing and deployment simple
Docker Architecture
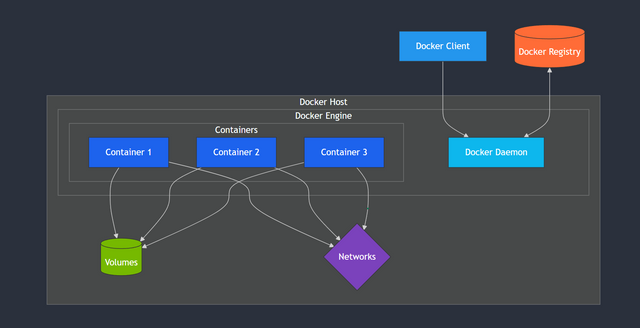
Docker has three main parts:
- Docker Client: where you type commands
- Docker Host: the server that runs containers
- Docker Registry: stores Docker images
Docker components
1. Images
Images are like blueprints for containers. They cannot change.
2. Containers
Containers are running copies of images. They have everything the app needs.
3. Volumes
Volumes save data even when containers stop.
Understanding Dockerfiles
A Dockerfile is a text file with steps to make a Docker image:
FROM node:16.5.0-alpine # Base image
WORKDIR /app # Sets working directory
COPY package.json ./ # Copies files from host to image
RUN npm install # Executes commands during build
CMD ["npm", "start"] # Default command when container starts
Maindockerfile instructions
- FROM: picks the base image
- WORKDIR: sets the work folder
- COPY/ADD: copies files into the image
- RUN: runs commands when building
- ENV: sets environment variables
- EXPOSE: shows which ports to use
- CMD/ENTRYPOINT: sets the start command
Docker Compose
Docker Compose helps run many containers together. It uses a YAML file:
version: "3"
services:
frontend:
build: ./frontend
ports:
- "3000:3000"
volumes:
- ./frontend:/app
backend:
build: ./backend
ports:
- "8080:8080"
depends_on:
- database
database:
image: postgres:13
environment:
- POSTGRES_PASSWORD=password
Key Docker Compose Ideas
- Services: the containers in your app
- Volumes: storage that stays
- Networks: how containers talk to each other
- Dependencies: which container starts first
Our Project's Dockerfiles
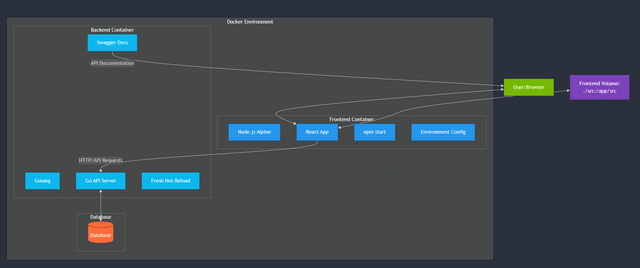
my architectcture application using mermaid
Let's analyze the Dockerfiles from our project:
Frontend Dockerfile
FROM node:16.5.0-alpine
WORKDIR /app
RUN node -v
RUN apk add libc6-compat
RUN apk add openssh-client
RUN apk add git
COPY package.json ./
COPY package-lock.json ./
RUN npm i
ADD . .
RUN ls -la
RUN chmod +x env.sh
RUN npm run env
RUN cp env-config.js ./public
CMD [ "npm", "run", "start"]
What this does:
- Starts with Alpine Linux Node.js
- Installs needed tools
- Copies package files first (good for caching)
- Installs npm packages
- Copies all project files
- Sets up environment settings
- Starts the app
Backend Dockerfile
FROM golang:1.16.5
WORKDIR /go/src/test/
COPY . .
COPY go.mod .
COPY go.sum .
RUN go mod download
RUN go get github.com/pilu/fresh
RUN go get github.com/swaggo/swag/cmd/[email protected]
RUN go get github.com/arsmn/fiber-swagger/v2
RUN go get gorm.io/[email protected]
ENTRYPOINT swag init --parseDependency --parseInternal && fresh
What this does:
- Starts with Golang image
- Sets the work folder
- Copies all files
- Downloads Go modules
- Gets tools for:
- Hot-reloading
- API docs
- Swagger UI
- Database work
- Sets up Swagger docs and starts the server
Docker Compose File
version: "3"
services:
web-dev:
build:
context: .
dockerfile: Dockerfile
ports:
- "3000:3000"
volumes:
- ./src:/app/src
environment:
- CHOKIDAR_USEPOLLING=true
container_name: front-app
What this does:
- Makes a service called "web-dev"
- Builds it using our Dockerfile
- Maps port 3000
- Mounts src folder (for live updates)
- Sets an environment variable
- Names the container
Docker Commands Cheatsheet
Command | Description |
---|---|
docker build -t myapp . | Build an image |
docker run -p 3000:3000 myapp | Run a container |
docker ps | List running containers |
docker images | List images |
docker-compose up | Start services defined in docker-compose.yml |
docker-compose down | Stop services |
docker exec -it container_name bash | Access a running container |
docker logs container_name | View container logs |
What's Next?
In Week 4, we'll explore frontend development with React & TypeScript, building on our containerized approach. We'll see how Docker simplifies the development process and helps us create a consistent environment across our tech stack.
Stay tuned for next week's topic: Frontend Development with React & TypeScript.
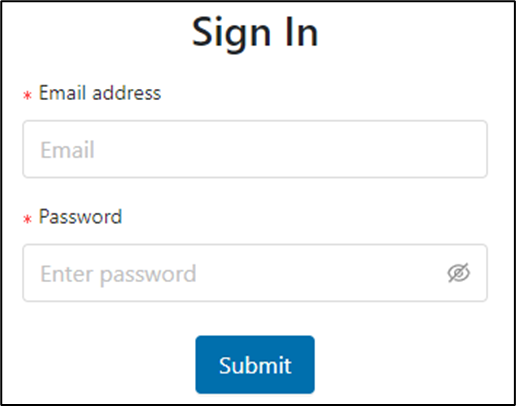
Authentication interface
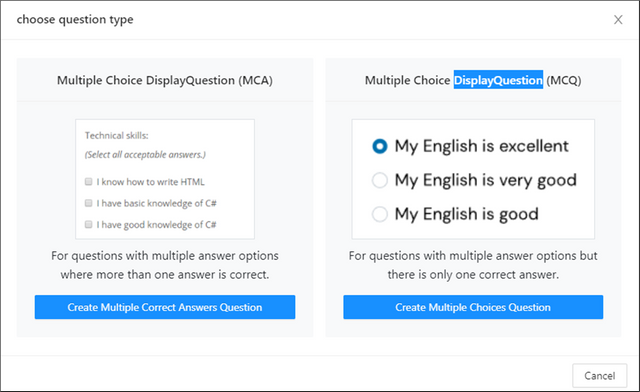
Question type selection interface
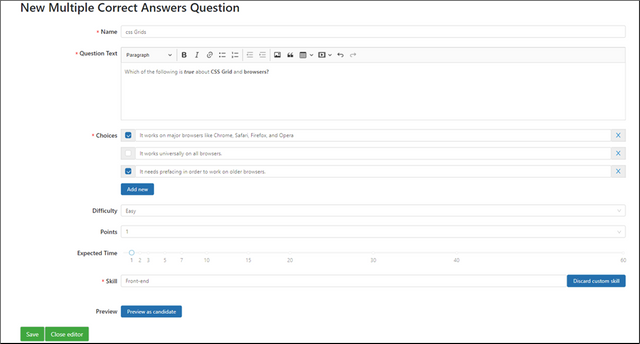
Interface for adding questions to a test
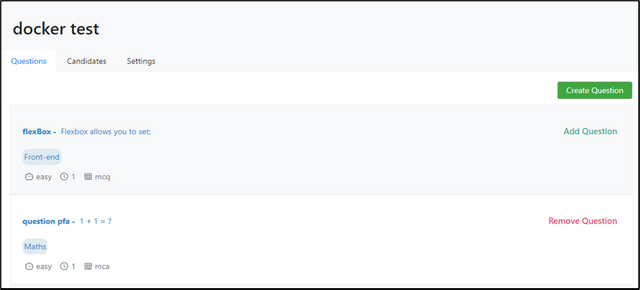
Interface for adding an MCQ-type question
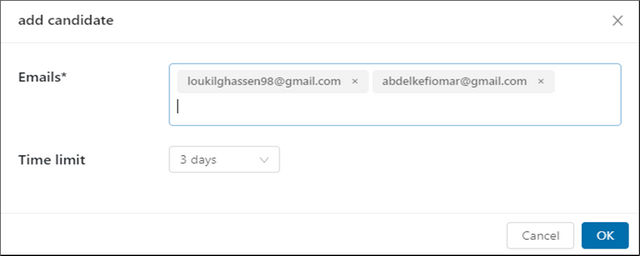
Interface for inviting a candidate to a test
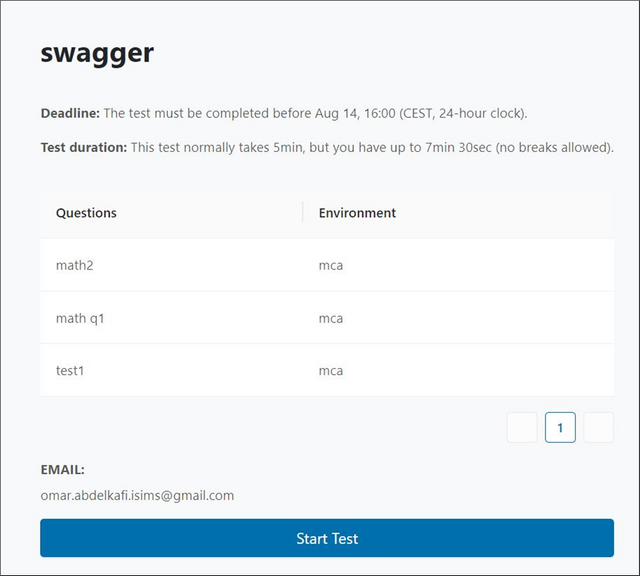
Candidate welcome interface
--
GitHub Repositories
- Frontend: TalentLens
- Backend: Candidly
Project Resources
- Complete System Overview: Google Drive Link
- Project Presentation Video:
What can you share in the club?
Our club is all about technology and development including:
- Web & Mobile Development
- AI & Machine Learning
- DevOps & Cloud Technologies
- Blockchain & Decentralized Applications
- Open-source Contributions
- Graphic Design & UI/UX
Any posts related to technology, reviews, information, tips, and practical experience must include original pictures, real-life reviews of the product, the changes it has brought to you, and a demonstration of practical experience
The club is open to everyone. Even if you're not interested in development, you can still share ideas for projects, and maybe someone will take the initiative to work on them and collaborate with you. Don’t worry if you don’t have much IT knowledge , just share your great ideas with us and provide feedback on the development projects. For example, if I create an extension, you can give your feedback as a user, test the specific project, and that will make you an important part of our club. We encourage people to talk and share posts and ideas related to Steemit.
For more information about the #techclub you can go through A New Era of Learning on Steemit: Join the Technology and Development Club. It has all the details about posting in the #techclub and if you have any doubts or needs clarification you can ask.
Our Club Rules
To ensure a fair and valuable experience, we have a few rules:
- No AI generated content. We want real human creativity and effort.
- Respect each other. This is a learning and collaborative space.
- No simple open source projects. If you submit an open source project, ensure you add significant modifications or improvements and share the source of the open source.
- Project code must be hosted in a Git repository (GitHub/GitLab preferred). If privacy is a concern, provide limited access to our mentors.
- Any post in our club that is published with the main tag #techclub please mention the mentors @kafio @mohammadfaisal @alejos7ven
- Use the tag #techclub, #techclub-s23w3, #country, other specific tags relevant to your post.
- In this thirdweek's #techclub you can participate from Monday, March 3, 2025, 00:00 UTC to Sunday, March 09, 2025, 23:59 UTC.
- Post the link to your entry in the comments section of this contest post. (Must)
- Invite at least 3 friends to participate.
- Try to leave valuable feedback on other people's entries.
- Share your post on Twitter and drop the link as a comment on your post.
Each post will be reviewed according to the working schedule as a major review which will have all the information about the post such as AI, Plagiarism, Creativity, Originality and suggestions for the improvements.
Other team members will also try to provide their suggestions just like a simple user but the major review will be one which will have all the details.
Rewards System
Sc01 and Sc02 will be visiting the posts of the users and participating teaching teams and learning clubs and upvoting outstanding content. Upvote is not guaranteed for all articles. Kindly take note.
Each week we will select Top 4 posts which has outperformed from others while maintaining the quality of their entry, interaction with other users, unique ideas, and creativity. These top 4 posts will receive additional winning rewards from SC01/SC02.
Note: If we find any valuable and outstanding comment than the post then we can select that comment as well instead of the post.
Technology and Development Club Team
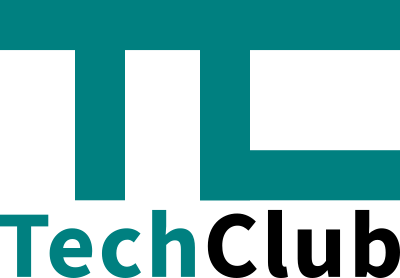
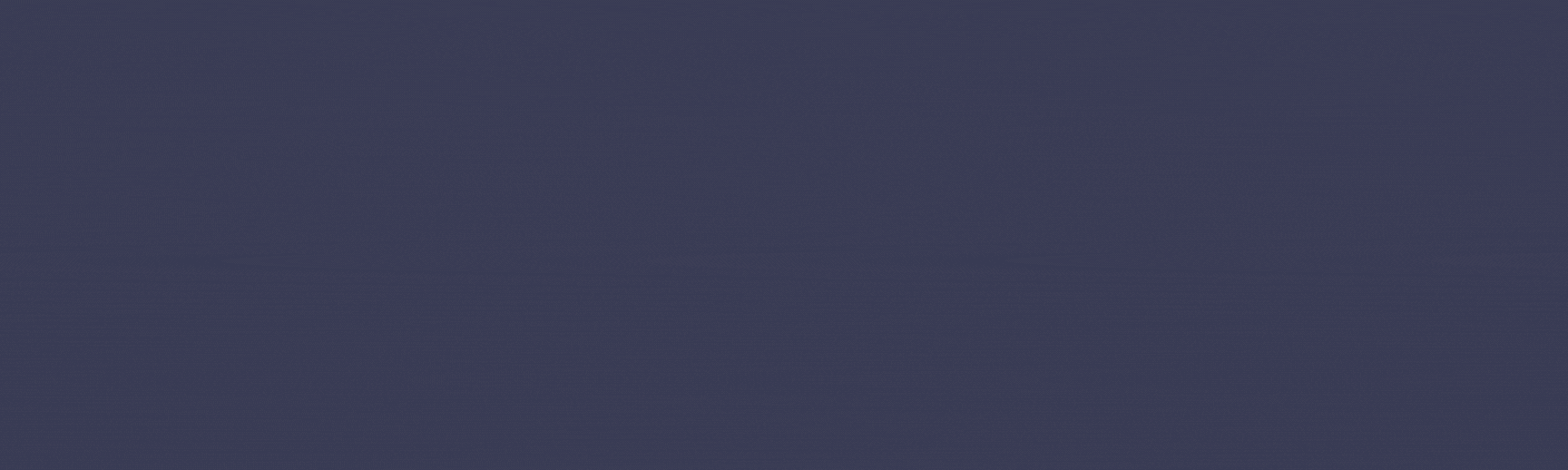
Upvoted! Thank you for supporting witness @jswit.
@aneukpineung78
@grebmot
what do you think?
Note
Title: Unclear what the content is
Hashtags: please, use what fits to the topic how should anyone find this (back)?
#countryis a hashtag because?🍀
Sorry, it's a more advanced technology, and even many programmers are not familiar with it. I will explain it to you in a simpler way. Docker is like a box where I write my code inside. Then, I can run this box on any machine. Usually, there is a common problem where code runs on my machine but not on another machine. This happens due to differences in tool versions or system configurations. However, with Docker, this issue does not occur because everything needed to run the code is packaged inside the Docker container.
Thanks for the extra explanation. I read it is a box although I cannot picture it but you described it well.
also I used docker for this tool
https://steemhub.tech/
I never understand programming, programming languages, and programmers. They live on different world, speak different language. So, I wouldn't bother, I will leave them be. =D
Just look at the picture, I left you something to complete.
Yes. I don't understand them.
Heh, I think I'm not smart enough to have strong opinions about that :-)
my entry post https://steemit.com/techclubs23w3/@hamzayousafzai/slc-s23w2-what-your-mood-a-thunkable-app-or-part-2
My entry link:
https://steemit.com/steem-for-betterlife/@azit1980/steemit-learning-club-s23w3-graphic-design-special-business-card-and-poster-design