SLC21 Week3 - Strings in C
Hello everyone! I hope you will be good. Today I am here to participate in Steemit Learning Challenge of @sergeyk about the Strings in C. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
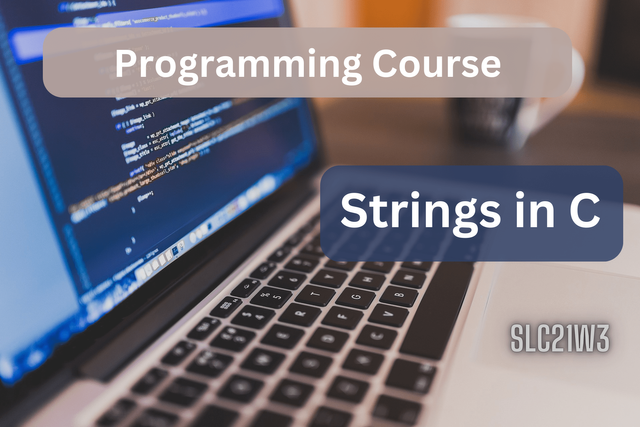-min.png)
Practically (i.e., with code examples) explain the theory from the first part of this lesson, where the concept of two sizes of an array is discussed. Demonstrate how to make it look like the array size can be increased/decreased. All loops should work with the array size stored in the size variable. Keep the physical, actual size in the constant N.
This code is a simple demonstration of managing an array in C++ with both a physical (fixed) and a logical size. The physical size is the array's total capacity, while the logical size represents the current number of meaningful elements stored in the array.
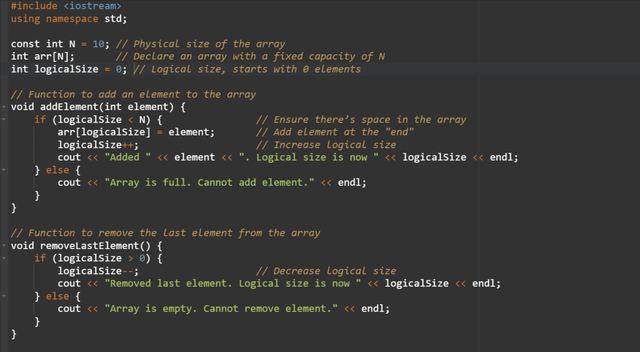
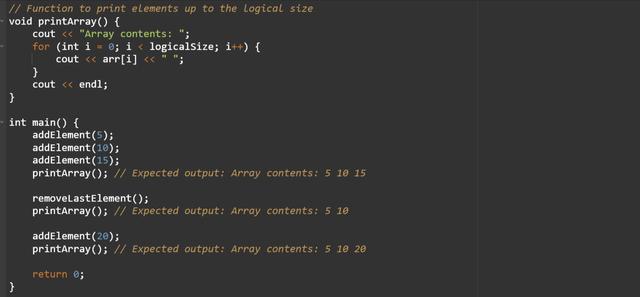
This code represents dynamic array behavior with a fixed size array by tracking the logical size separately from the physical capacity. The functions addElement
and removeLastElement
manage logical changes to the array content. It allows the array to mimic resizing even though its actual size (N
) is fixed.

N
is a constant representing the physical (maximum) size of the array. I have set its size to 10. Soarr
can hold up to 10 elements.arr
is the array with a fixed capacityN
, and I have initialized it as an empty array.logicalSize
This variable is used to keep track of the current number of elements in the array. It is starting at 0.
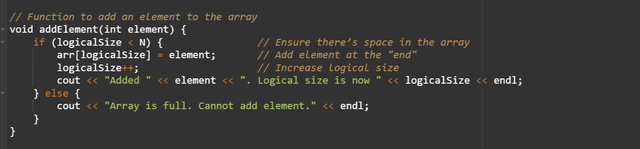
- Purpose: This part of code is sued to add a new element to the array if there is available space.
- Working:
- It checks if
logicalSize
is less thanN
which is the array's capacity. If it is true then it proceeds. - The element is added to
arr[logicalSize]
. The element is placed at the "end" of the currently used portion of the array. - The logical size
logicalSize
is then incremented to reflect the addition. Each time an element is added this variable is also incremented. - If the array is already full then a message will be displayed that no more elements can be added.
- It checks if

- Purpose: This is part of code is used to remove the last element in the logical part of the array if any elements exist.
- Working:
- It checks if
logicalSize
is greater than 0 to ensure if there are elements to remove. - If it is true then
logicalSize
is decremented by 1. It removes the last element by decreasing the count. - If the array is already empty a message is displayed to indicate this.
- It checks if

- Purpose: It prints the elements in the logical part of the array.
- Working:
- It iterates from
i = 0
up tologicalSize - 1
. It prints each element present in thearr
. - It only prints the elements in the logical range
[0, logicalSize-1]
.
- It iterates from
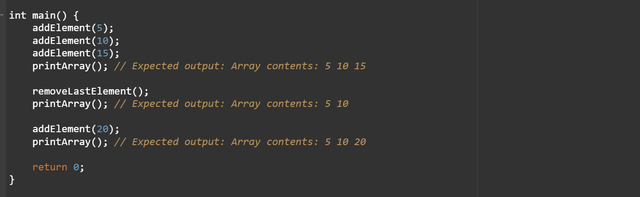
In the main function I have called all the functions such as to add elements in the array. And then I have called the print function to display the elements in the array. Then I have tested the the remove function. It reduces the logical size and printed the array. After that I again called the function to add another new element in the array.

This is the output of the program where you can see I have added elements in the array and then I have tested other functions as well to check out if the size of the array is changing and working correctly.
Declare a string variable (store any sentence in the array). Task: reverse the string, i.e., write it backward. For example: char s[]="ABCDEF";.....your code.....cout<<s; => FEDCBA
In order to reverse the the string stored in the character array we can swap elements from the beginning and the end of the array until we reach the middle. We use two pointers for this swapping. One pointer will start from the beginning such as as from i = 0
and at the end such as len - 1
. We will move the pointers inward where the start pointer will be incremented and the end pointer will be decremented. Each swap places two characters in their correct position.
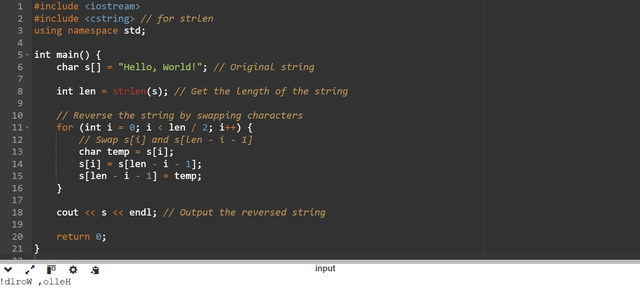
strlen(s)
calculates the length of the string by excluding the null terminator.- The
for
loop iterates fromi = 0
toi < len / 2
to swap elements from the start and end of the array. s[i]
ands[len - i - 1]
are swapped for each iteration effectively by reversing the string in place.
This reversing program has reversed Hello, World! to !dlroW ,olleH. This is how we can reverse the string. In this string if I store the text Faisal it will reverse it efficiently by outputting lasiaF.
Swap neighboring letters char s[]="ABCDEF";.....your code.....cout<<s; => BADCFE
In order to swap the neighboring letters in the string which is stored in the array we can iterate through the array two characters at a time and then we can swap each pair. I will define the string with a character array which will contain the original string such as char s[] = "ABCDEF";
. Then I will use a loop to go through the string by incrementing two characters at a time. Then I will swap each pair of characters with the next one.
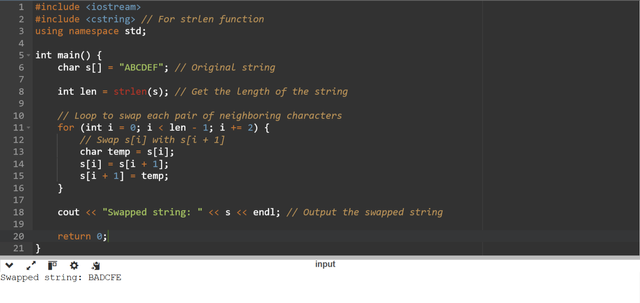
for (int i = 0; i < len - 1; i += 2)
loop iterates over the array moving by two characters at a time.len - 1
ensures thats[i + 1]
is valid.char temp = s[i];
temporarily stores the current character (s[i]
) to swap it with the next one.s[i] = s[i + 1];
assigns the next character (s[i + 1]
) to the current position (s[i]
).s[i + 1] = temp;
completes the swap by placingtemp
(originals[i]
) in the next position.
Shift the string cyclically to the left (it’s easier to start with this), then cyclically to the right. char s[]="ABCDEF", x[]="abrakadabra";.....your code.....cout<<s<<"\n"<<x; => BCDEFA aabrakadabr
In order to perform the cyclic shifts on a string in C++ we can use array manipulation to move the characters to the left or right while maintaining the original length of the array. We can do left cyclic move as well as right cyclic move.
- Right Cyclic Shift: For the right cyclic shift we move each character one position to the right side and similarly we bring the last character to the beginning of the string.
Explanation of Steps
char last = arr[len - 1];
temporarily saves the last character of the string. Fors = "ABCDEF"
,last
will hold'F'
.- The
for
loop goes from the end of the string to the start (i = len - 1
toi > 0
). - Each character is moved one position to the right:
arr[i] = arr[i - 1];
. - For example, if
s = "ABCDEF"
, after shifting, it looks like"AABCDE"
(note that the last position is overwritten). arr[0] = last;
saved last character ('F'
) is assigned to the first position, resulting in"FABCDE"
.
So after the right shifting of s = "ABCDEF"
it becomes "FABCDE"
.
- Left Cyclic Shift: For the left cyclic shift we move each character one position to the left and then bring the first character to the end of the string.
Explanation of Steps
char first = arr[0];
temporarily saves the first character of the string. Fors = "ABCDEF"
,first
will hold'A'
.- The
for
loop goes from the start of the string to the second-to-last position (i = 0
toi < len - 1
). - Each character is moved one position to the left:
arr[i] = arr[i + 1];
. - For example if
s = "ABCDEF"
then after shifting it looks like"BCDEEF"
(note that the first position is overwritten). arr[len - 1] = first;
saved first character ('A'
) is assigned to the last position, resulting in"BCDEFA"
.
So after the left shifting of s = "ABCDEF"
it becomes "BCDEFA"
.
Remove all vowel letters char s[]="this is some text";...your code...cout<<s; => ths s sm txt
It is very simple to remove the vowels from the string. In order to remove the vowel letters from the string s
we can use a loop to copy only non vowel characters into the string itself.
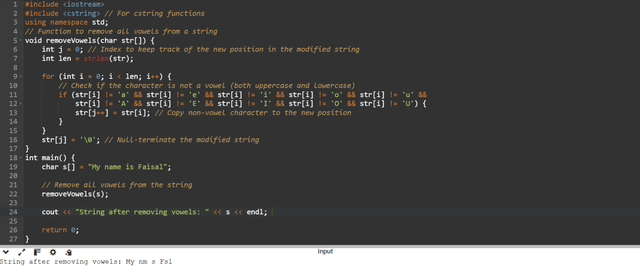
Working of removeVowels
Function:
- This loops through each character of the input string
str
. - This function checks if the character is not a vowel it checks both lowercase and uppercase letters. Ass we already know all the vowel letters so there is a check condition on all the vowel letters in upper and lower case.
- If it finds a consonant (non-vowel) letter it copy it to the next available position tracked by the index
j
. - After all non vowel characters have been copied the string is null terminated at the end.
I gave this input char s[] = "My name is Faisal";
and the program removed all the vowels from the string and it returned M nm s Fsl
. In this way we can remove vowels from any string and can return the remaining string after the filteration.
Double each vowel letter char s[]="this is some text";...your code...cout<<s; => thiis iis soomee teext
This is similar task with the previous one but in previous one we removed the vowels from the string where they were available. But now in this question we need to double the vowels found in the string on their place. We will use a loop to iterate through all the string and double the vowels.
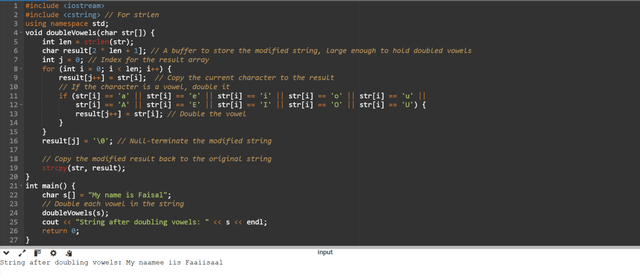
doubleVowels
Function:
- This function creates a new array
result
with sufficient space to accommodate doubled vowels. - With the help of the loop it iterates over each character in the original string.
- It checks if the character is a vowel then it copies it twice into the
result
array. - After doubling all the vowels the modified string in
result
is copied back to the originalstr
array.
Usage:
This approach preserves the original string structure while doubling only the vowels. You can see that I have given this input My name is Faisal
and after doubling all the vowels in this string it has returned this output My naamee iis Faaiisaal
. In this way we can double the vowels in the string.
Note: I have used Onlinegdb Compiler to compile the code in C++ language.
Congratulations, your post has been upvoted by @scilwa, which is a curating account for @R2cornell's Discord Community. We can also be found on our hive community & peakd as well as on my Discord Server
Felicitaciones, su publication ha sido votado por @scilwa. También puedo ser encontrado en nuestra comunidad de colmena y Peakd así como en mi servidor de discordia