SLC21 Week2 - Programming arrays
Hello everyone! I hope you will be good. Today I am here to participate in Steemit Learning Challenge of @sergeyk about the Programmin arrays. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
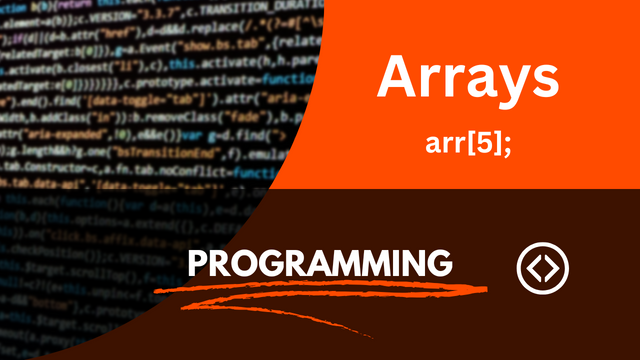.png)
Question 1
Declare an array of any type:
Arrays is an important element of data structure. It stores collection of the data with same data types. It is very simple to declare an array in any programming language. I will talk about declaring it in C++.
- We need to define the data type of the array
- Then we need to set the name of the array
- Then we need to define the size of the array
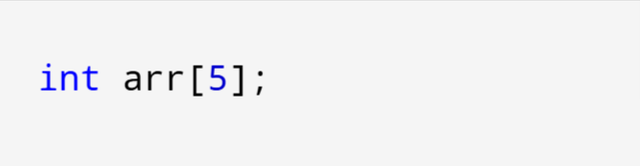
Here:
int
is data typearr
is name5
is the size of the array
explain how to use an array, how to access array elements.
This part is also linked with the previous one in order to use an array first of all we should have an array. I will use previously declared array int arr[5];
. So the array has declared already now we need to initialize the array.

Each element of the array is accessed trough the index of the array. Each index has a memory location and it returns the memory stored in that index.

Here I am accessing the first and third element by using the index of the value.

We can use loops to print all the elements in the array without writing print statement one by one for the elements.
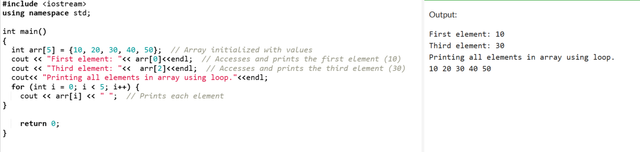
What are the advantages of an array over ordinary variables
Arrays have a number of advantages over the ordinary variables. Array is widely used in the data structure. Some advantages are given below:
Arrays allow us to to store multiple values with only one name. It helps to manage the large data without declaring many individual variables.
Arrays are efficient in the allocation of the memory. They allocate contiguous blocks of memory. It makes accessing and processing of the elements faster as compared to other individual variables which are scattered in the memory.
Arrays allow access to elements with the help of the indices. This enables easy access and modification. We can process data using loops. It simplifies complex and time taking tasks.
Arrays reduce the complexity and length of the code. For example
for
loop can process all elements by making the code shorter and clean.
These advantages make the arrays ideal for handling collections of data efficiently in the programming.
Question 2
What is the name of the array? What will happen if you display this value on the screen? What does cout<<a+2;that mean cout<<a-2;? If cout<<a;4,000 is displayed when outputting, then how much will it bea+1?
Name of the Array: The name of the array such as
a
inint a[5];
represents the address of the first element in the array. Soa
itself is a pointer to the first elementa[0]
.Displaying the Array Name (
cout << a;
): When we will display the array name such ascout << a;
then it will show the memory address of the first element of the array. It will not show the array values.cout << a + 2;
andcout << a - 2;
:cout << a + 2;
displays the memory address of the third element in the arraya[2]
. When we add 2 toa
then the pointer moves by two elements in the forward direction.cout << a - 2;
tries to display a memory address two elements beforea
. It can lead to undefined behaviour because it will go outside the boundary of array.
Given
cout << a;
Displays4000
:- If
cout << a;
displays4000
, thencout << a + 1;
will output4004
for anint
array. Because eachint
takes 4 bytes in memory. The address increases by the size of theint
type (4 bytes). So each increment adds 4 bytes.
- Moreover if we use
double
orlong
data type then it will behave differently because in the these data types each element takes 8 bytes. And after one increment in4000
will become4008
.
- If
Question 3
Can an array have two dimensions?
Usually we study about the one dimensional arrays but arrays can also have 2 dimensions. In the 2 dimensional array the rows and columns are defined for the data. It is commonly known as a 2D array and it is an array of arrays. In the 2D array we can access the elements with two indices one is used for the rows and the other is used for the columns.
We can declare the 2D array in this way:
int arr[3][4];
Here:
- The 2D array has 3 rows and 4 columns.
After declaring I have intialized this 2D array with some values to show the working.
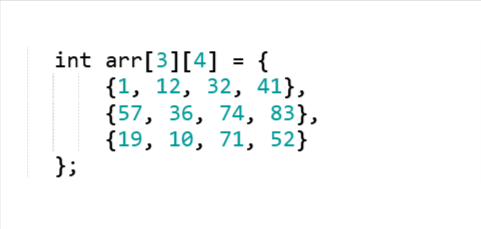
Now in order to access the elements of the 2D array we need to access the required element by traversing through the row and the column. We need to mention the row and column of the number to access.
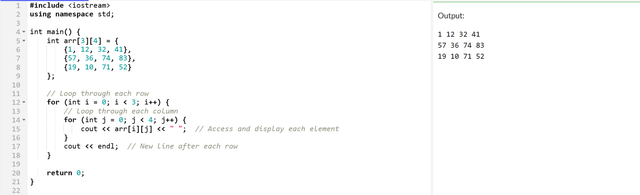
i
iterates through the rows.
j
iterates through the columns within each row.
arr[i][j]
accesses each element in the 2D array.
Question 4
Write a random number in the variable k. int k=(rand()%101) * (rand()%101) * (rand()%101)+500;Try to solve the problem of finding divisors from the last lesson more efficiently (so that the divisors are found faster) and write the results (not on the screen) but in an array. Since the transfer of arrays to a function is not a simple topic - filling the array should not be done in the form of a function!!!
In order to solve this problem effectively I will generate a random number for k
. Then I will find the divisors effectively by just iterating up to the square root of k
. And I will declare an array to store the divisors in that. And as per the restriction I will not use function for this purpose.
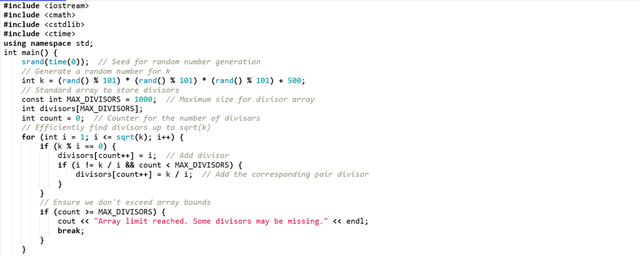
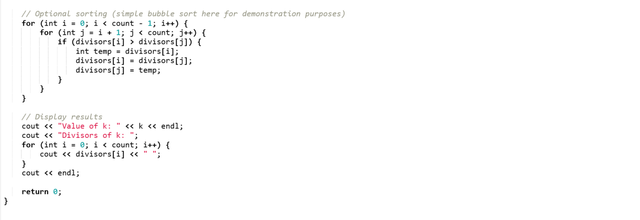
In the above program the expression (rand() % 101) * (rand() % 101) * (rand() % 101) + 500
is generating a random number and in each random number it is adding 500. And this random number is being stored in the integer k
.
Then there is a standard array. I have defined an array of size 1000 which will store the divisors of the random number k
. We can also use a dynamic array which will allocate memory according to the divisor coming but as it has not been taught so I am not using it.
After that there is the code to find divisors of the random number efficiently. In order to find the divisor the code is only iterating up to the sqrt(k)
and it reduces the number of checks to find the divisors. When a divisor i
is found then we store i
and k/i
to capture the divisor pair.
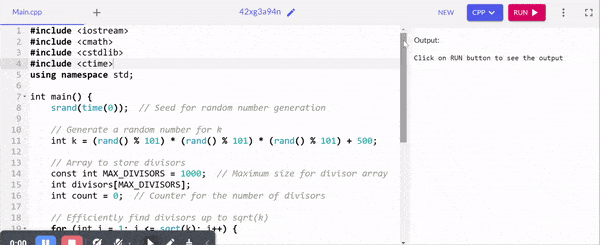
Question 5
Fill the array with 55 numbers with random numbers from 10 to 50. If there is a number 37 among the elements of the array, print it yes, and if there is no such number, print it no
We can easily fill a standard array with the 55 random numbers with the range of 10 to 50. And then we can check those generated numbers to check the availability of the number 37. Then we will implement the condition that if the number 37 is found we will print yes and if it is not found then we will print no.
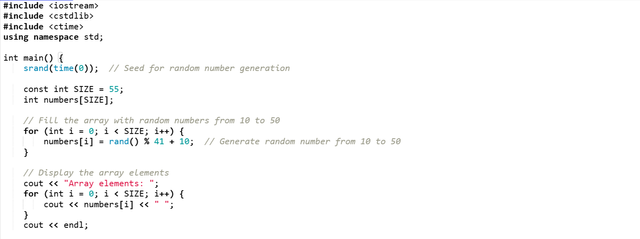
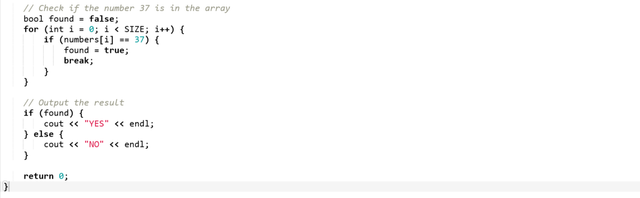
As we want to generate 55 random numbers so need a container to store those 55 numbers. I have declared an array of size 55 to store the random numbers. rand() % 41 + 10
is generating numbers from 10 to 50.
Then I have written the accessible code to display the array of 55 random numbers on the screen. Then I have defined a bool
variable as found
with the default value of false
. Then there is the condition to check the presence of number 37 and if the number is found then the variable found
is set true.
Then I am printing Yes or No based on the condition fulfilled.
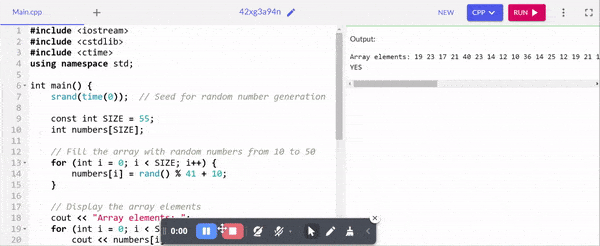
Question 6
Fill an array of 66 numbers with random numbers from 12 to 60. Replace even elements with 7 and odd elements with 77
Here is the program which will fill an array with 66 random numbers from 12 to 60. Then this will replace even elements with 7 and odd elements with 77.
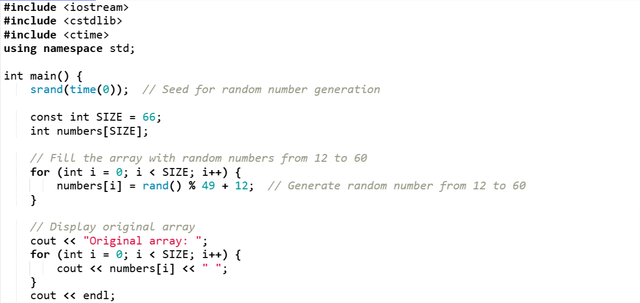
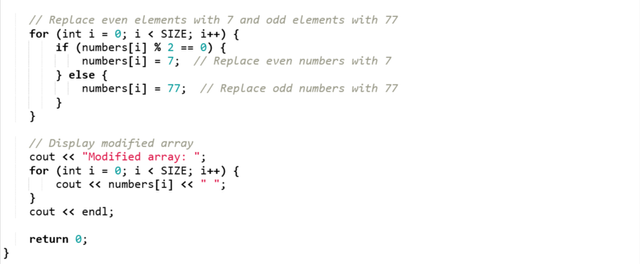
At first I have initialized an array of 66 size. I have then used a for loop to fill the array with the random numbers from 12 to 60.
Then there is a for
loop to display the original array filled with the random numbers.
Then I have used for loop again to traverse to all the numbers and to check if the number on each index is even or odd. If the even number is found then it is replaced with 7
and if an odd number is found then it is replaced with 77
.
At the end of the program I have displayed the modified array after replacing even with 7 and odd with 77.
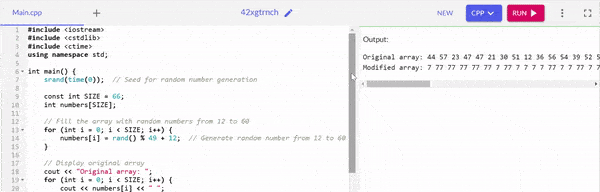
This is the output of the program.
Question 7
Fill the 77-number array with random numbers from 102 to 707. Find the two largest numbers. But the phrase "the two largest numbers" can have many interpretations. Therefore, first explain well how this phrase was understood. And then solve the problem.
In this case we can interpret the phrase "the two largest numbers" in two ways:
1. Two Largest Unique Numbers: We can find a largest number and the second largest unique value in the array. For example if the largest number appears multiple times then only one instance is considered for each unique value.
2. Two Largest Elements: We can simply select the two largest numbers from the array whether they are the same value or not.
In order to make it clear I will work with the first option. I will find the two largest unique numbers in the array. This ensures that if the largest number appears multiple times it is counted only once.
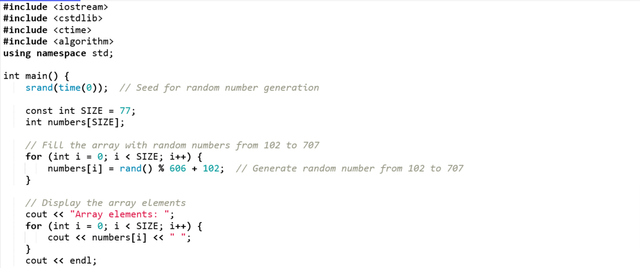
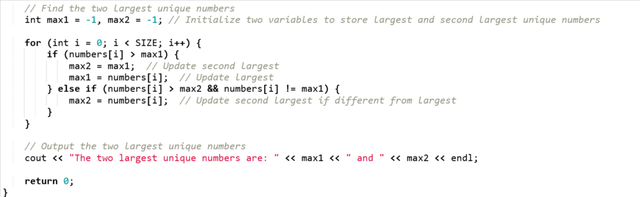
At the start there is an array which can take up to 77 numbers. Then rand() % 606 + 102
is generating random numbers from 102 to 707. Then I am printing the array with the random numbers on the screen.
After that I have initialized max1
and max2
to store the largest and the second largest unique numbers. It is checking each element. And if the element is greater than max1
the max1
becomes max2
and similarly the new number becomes max1
.
If the number is greater than max2
but not equal to max1
then it becomes the new max2
. In this way the two unique largest numbers are printed.
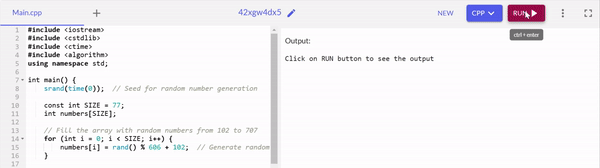
Here you can see the code is finding the two largest unique numbers by only making one pass through the array.
Disclaimer: I have used OneCompiler and Programiz to execute the code and to get the output.
X Promotion: https://x.com/stylishtiger3/status/1854828233520222378