Sending Encrypted Messages using Python
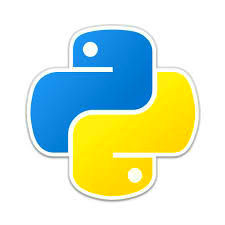
Sending encrypted messages to remote computers has been made easy with Python and simple-crypt
simple-crypt is module that can be added to python making encryption an easy task.
1) We will start by writing a script to receive our encrypted messages.
--receive.py
-import os from socket import * from simplecrypt import decrypt from config import port,key host = "" buf = 1024 addr = (host, port) UDPSock = socket(AF_INET, SOCK_DGRAM) UDPSock.bind(addr) print "Waiting to receive messages..." while True: (data, addr) = UDPSock.recvfrom(buf) msg = decrypt(key, data) print "Received message: " + msg if msg == "exit": break UDPSock.close() os._exit(0)--
2) Go ahead and save that script then we can create a script to send encrypted messages to the machine running the receive code.
--send.py
-import os from socket import * from simplecrypt import encrypt from config import host,port,key addr = (host, port) UDPSock = socket(AF_INET, SOCK_DGRAM) while True: data = raw_input("Enter message to send or type 'exit': ") ciphertext = encrypt(key, data) UDPSock.sendto(ciphertext, addr) if data == "exit": break UDPSock.close() os._exit(0)--
3) Now that we are ready to send and receive encrypted messages lets create our config file
--config.py
-host = "XX.XX.XX.XX" # IP address of computer running receive.py port = 13000 # Port number can be changed to communicate over any port key = "Your_password"--
You should now have 3 files name "receive.py", "send.py", and "config.py". Once everything is saved and your config file is filled out properly. You are now ready to send encrypted messages using python.