SLC S22 Week3 || Inheritance and Polymorphism in JAVA
Hello World!
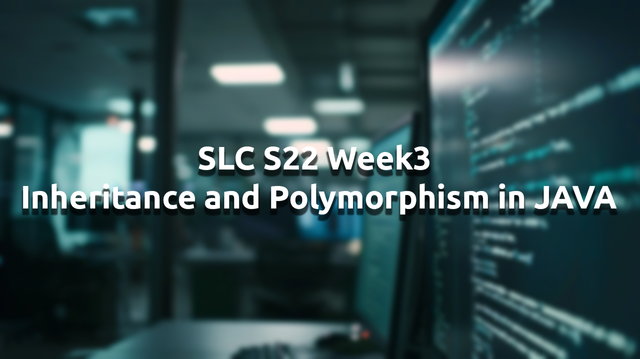
Image taken from Pixabay
Another week, another challenge, @kouba01 strikes again with a new Java Challenge, if you'd like to check it out and maybe join you can see it here SLC S22 Week3 || Inheritance and Polymorphism in JAVA.

- Write a Java program that creates a
Person
class with attributesname
andage
. Then, create aStudent
class that inherits fromPerson
and adds the attributestudentId
. Demonstrate how to create objects of both classes and display their details.
Let's start creating the two mentioned classes, first will be the Person
class. We declare the attributes first name
and age
, and the constructor Person
. All that's left for the Person
class is to setup a method that shows the details, displayDetail
that will print name and age. This is how it looks so far:
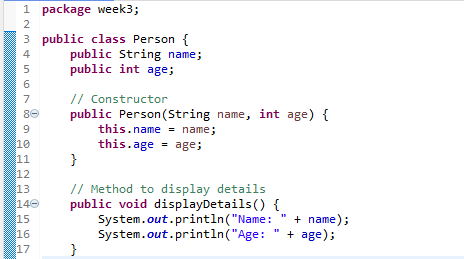
Moving to the Student
class, we need to declare the new attribute specific to this class, studentId
and constructor in which case needs to call super
for the Person
's class constructor and add the new attribute in the constructor. Super
calls the constructor of the parent's class, in our case the one from Person
.
We also need to create a new method to display the details for the Student
, in this case we are calling the Person
displayDetails
and add the new attribute we have in the Student
class, using it like super.displayDetails()
.
The Student
class looks like this:
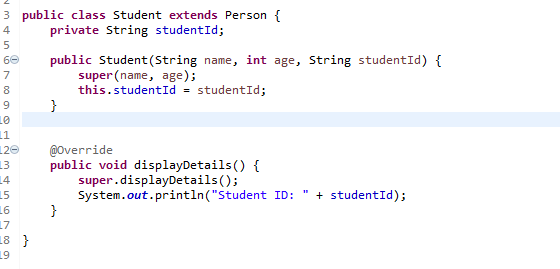
All that's left is to create the ```main`` and create the objects.
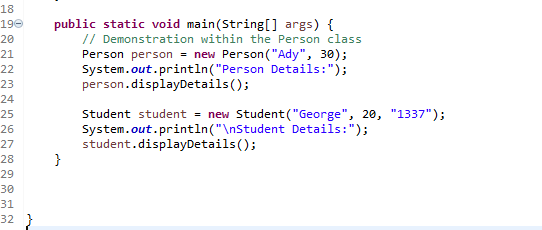
A GIF with the code being run:
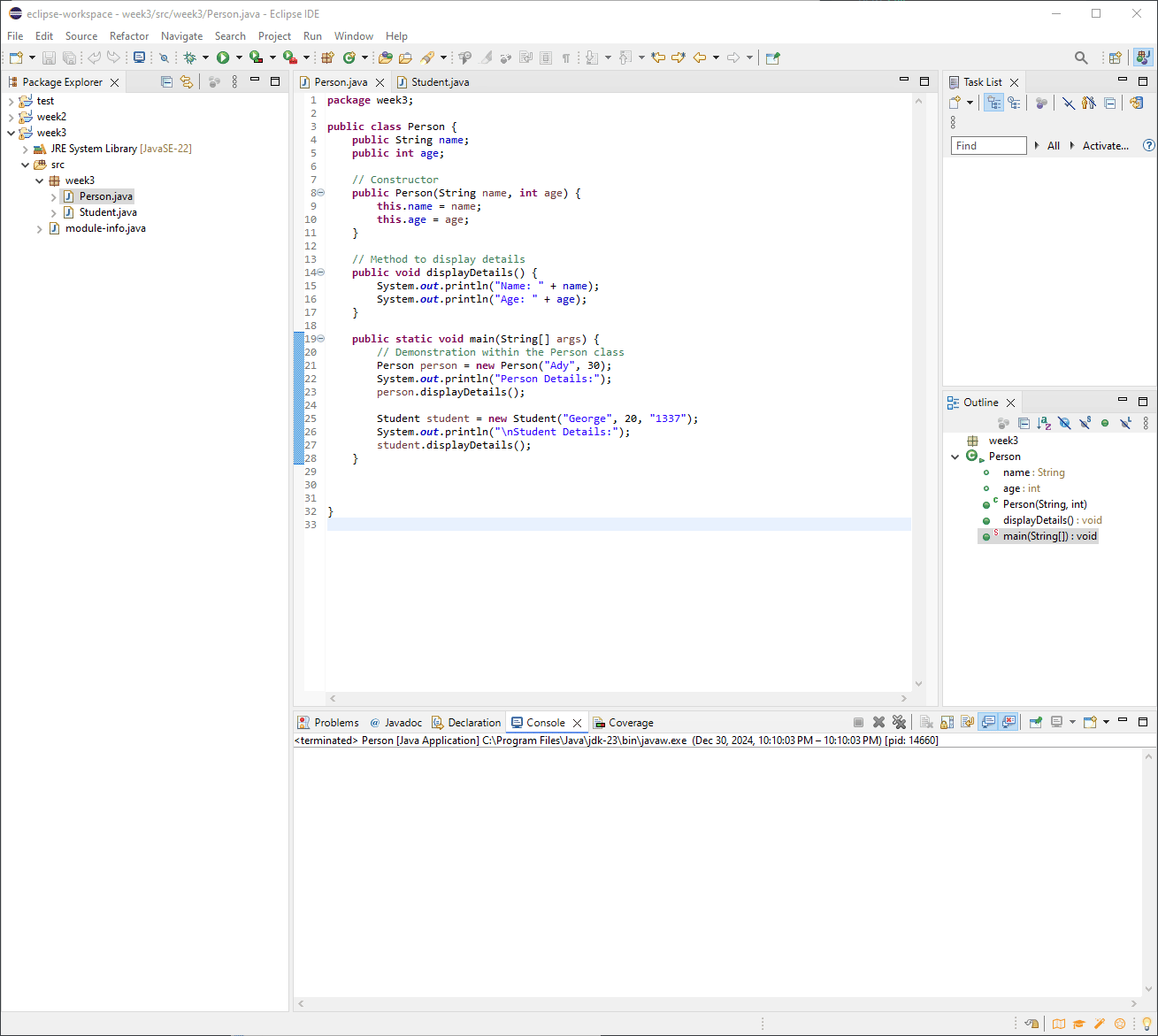


- Create a
Shape
class with overloaded methodsarea()
to calculate the area of different shapes (circle, rectangle, and triangle) based on the provided parameters.
We need to start with the area formulas that we need to apply:
- Circle:
pi * radius * radius
- Rectangle:
length * width
- Triangle:
1/2 * base * height
Now let's get to the coding part, this should be pretty easy as we have to overload the area method, based on it's parameters the right one will be picked and calculated, so we have it like this:
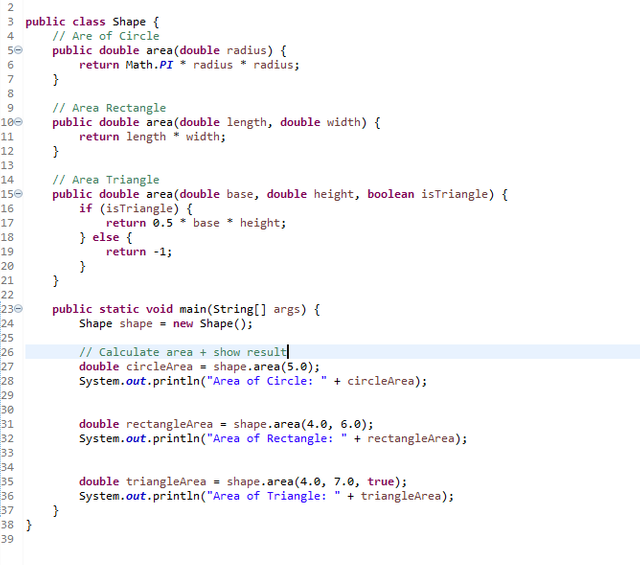
For the circle we have a method just with the radius, rectangle comes with length and width and triangle with base, height and a boolean to check if it's a triangle so it won't be confused with the rectangle one. All of them are being called in main with corresponding values and the result looks like this:
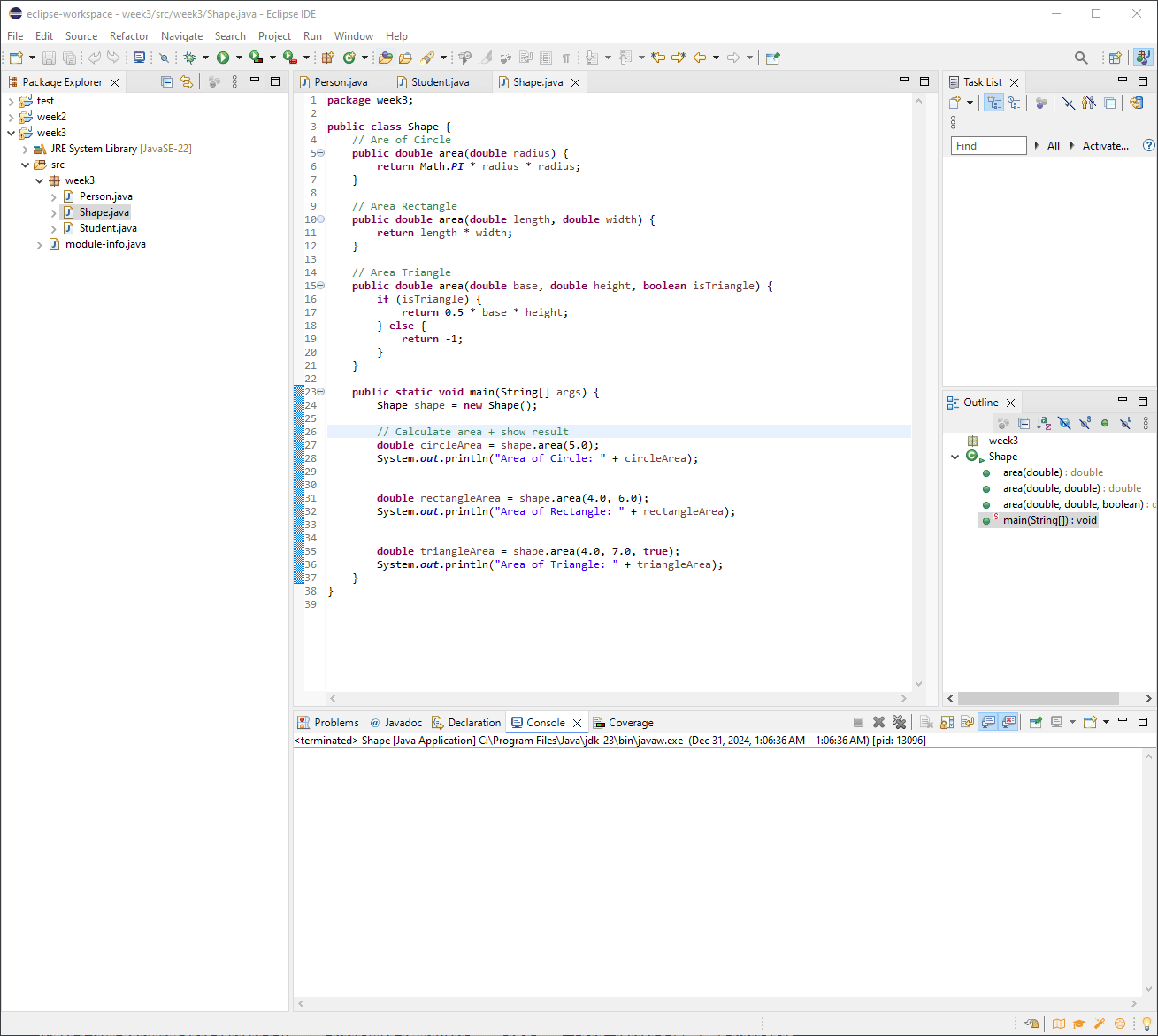


- Develop a
Bank
class with a methodgetInterestRate()
. Create two derived classesSavingsBank
andCurrentBank
that override thegetInterestRate()
method to return specific rates. Write a program to display the interest rates for both types of banks.
Now let's define the classes we are going to use, starting with Bank
, this will have a 0.0 default rate.
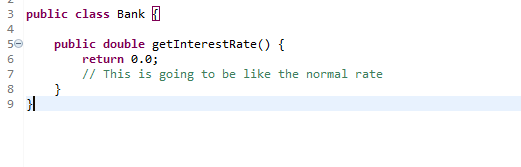
For the SavingsBank
we are going to create the same getInterestRate
but this time Overriding the one from the Bank
, returning 11.7. Also it needs to extend the original Bank
class.
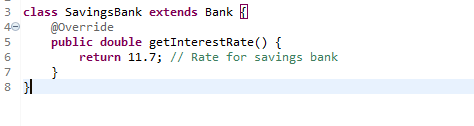
And for the CurrentBank
we are doing the same thing, create it's own getInterestRate
and make sure it extends the Bank
class. Also I will add the main
so we can run the code.
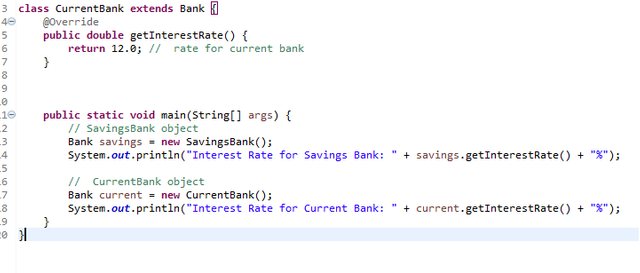
And the code live:
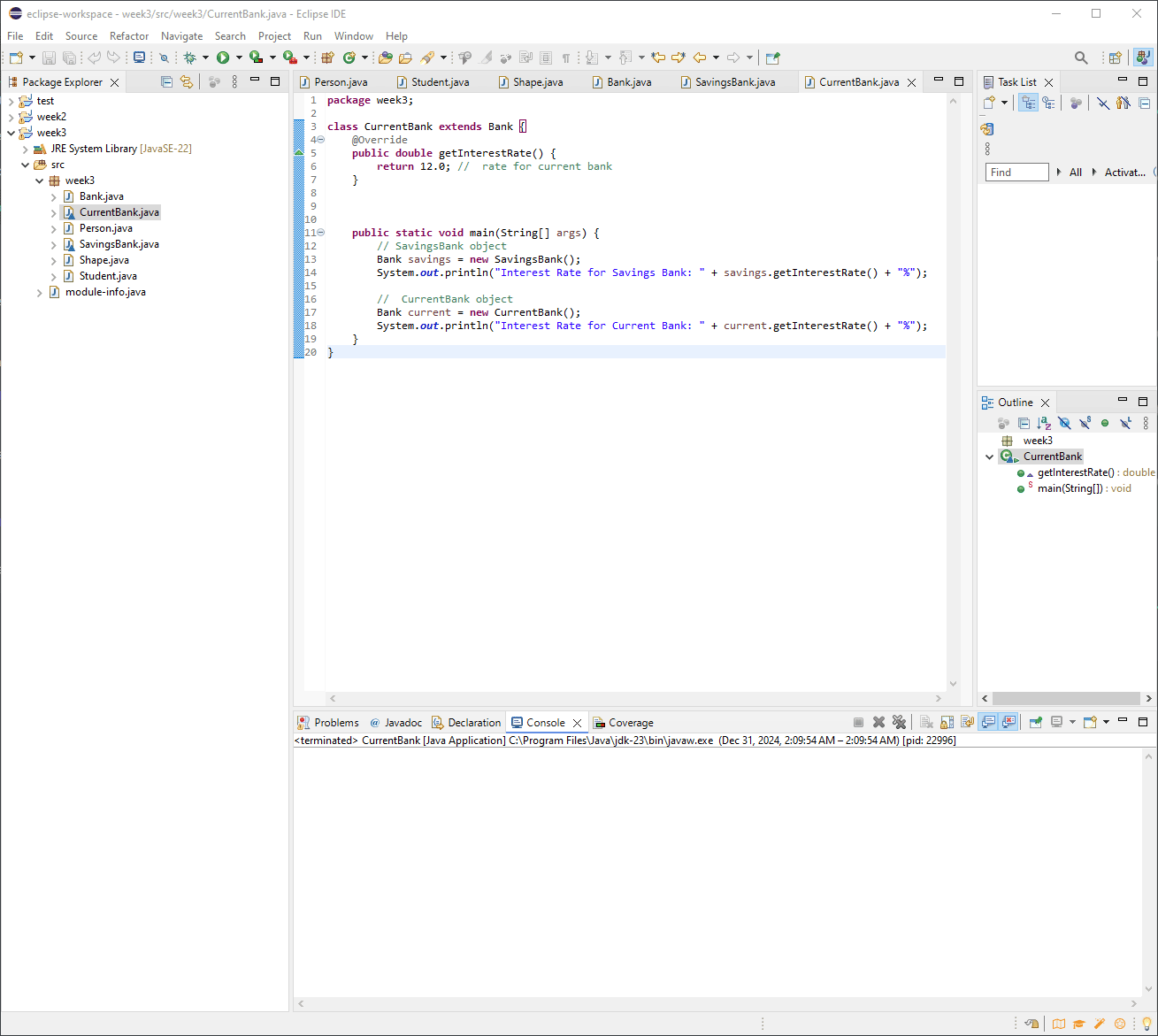


- Create a class hierarchy with a base class
Vehicle
and derived classesBike
andTruck
. Implement a methodstartEngine()
in the base class and override it in the derived classes to display specific messages. Use polymorphism to call the methods through a base class reference.
Starting with the Vehicle
class, we need to create a startEngine()
method for it. The class would look like this:

The same thing applies for Bike
and Truck
but the difference is that for these we are going to override the startEngine()
method, like this:
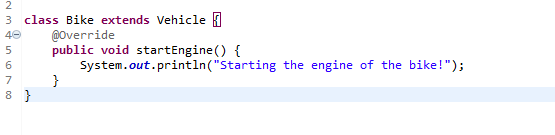
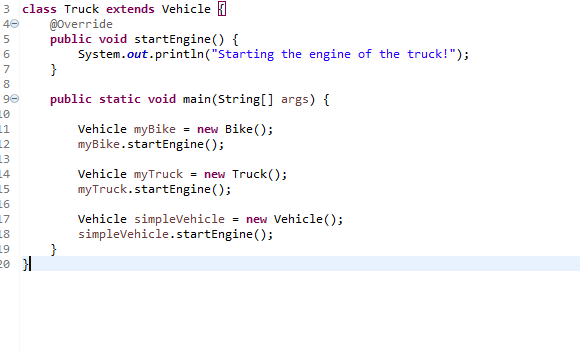
Also in the Truck
I've added the main
and created the objects and called startEngine()
. The output looks like this:
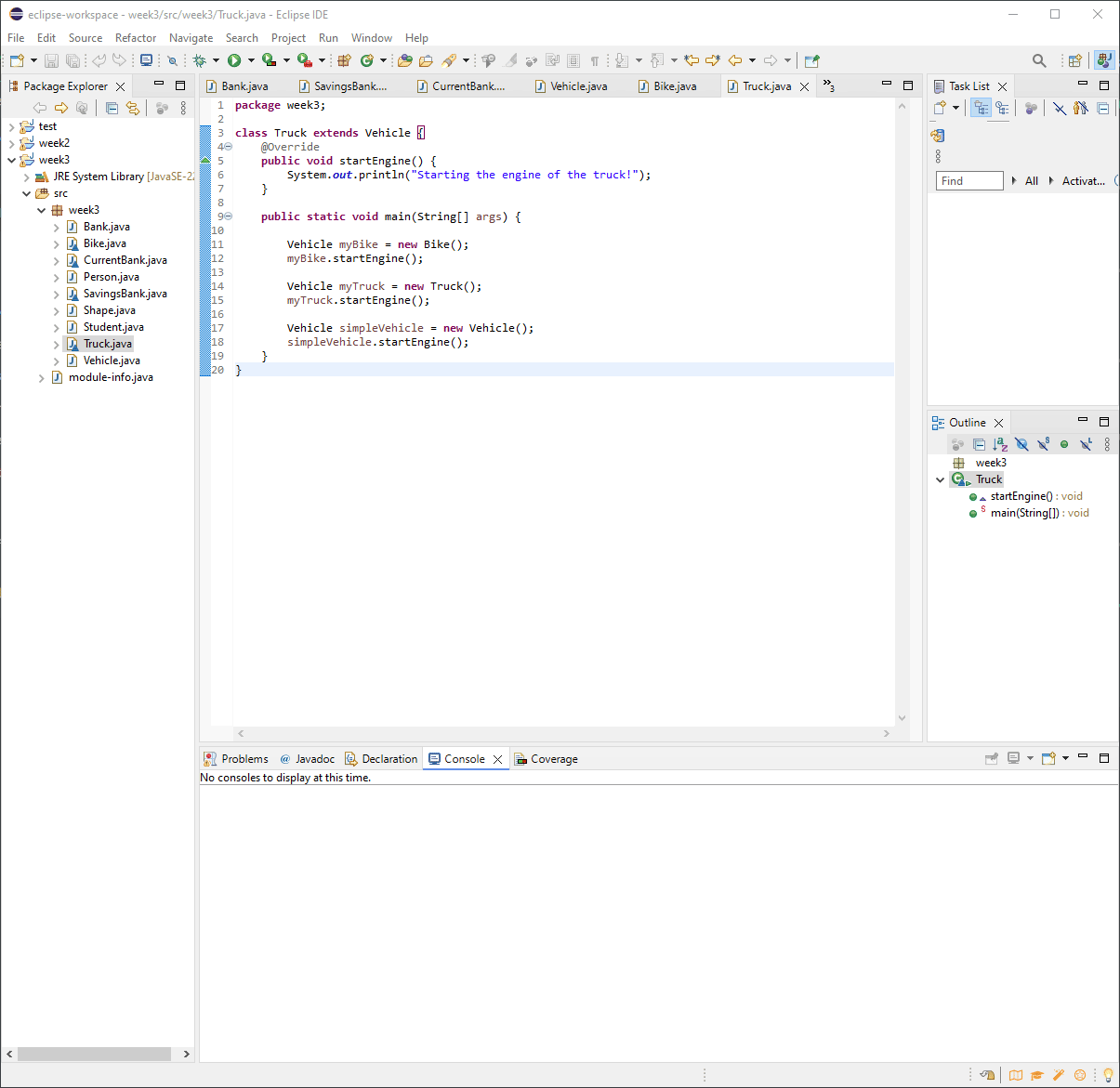


- Build a Library Management System that uses inheritance and polymorphism. Create a base class
Item
with attributesid
,title
, andisAvailable
. Extend it into derived classesBook
andMagazine
with additional attributes. Implement polymorphic methods to borrow and return items, and display their details.
This task is a little bit longer than the ones we worked above, but shouldn't be a problem as the idea behind it is the same. Let's start with the base class, Item
.
Defining the attributes id
,title
,isAvailable
, creating the constructor Item
, and the methods that will be used.
We need a method to borrow item, return item, display details and some getters for title and id. These are pretty basic, in the borrowItem
and returnItem
we will use a boolean variable to check if the title is available or not.
The class looks like this:
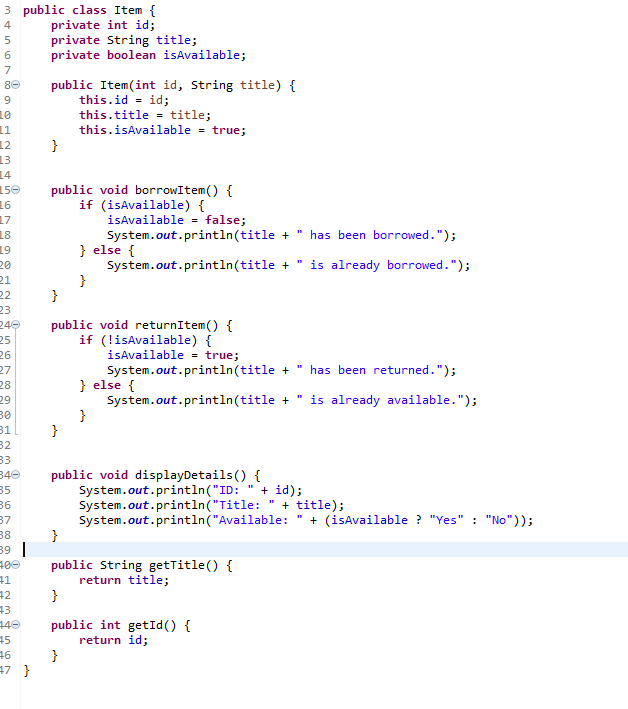
Now for the Book
class we need to add an extra attribute, we'll add the author
, we create the constructor and call super for the base class constructor and also we need to Override
the displayDetails
because here we have an extra attribute, the author
.
This is how the Book class looks like:
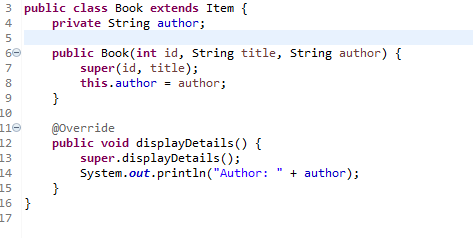
Same goes for the Magazine
class, we are going to add a new attribute issueDate
and use it in the constructor, also we are going to Override
the displayDetails
.
Class looks like this:
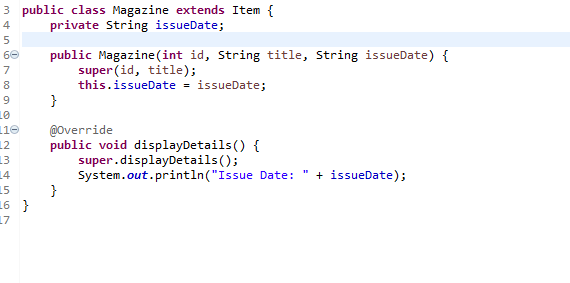
We have the Item
, Book
and Magazine
, now we need to manage them in a LibraryManagement
class.
Creating some objects and adding them in an array for simple use later on.
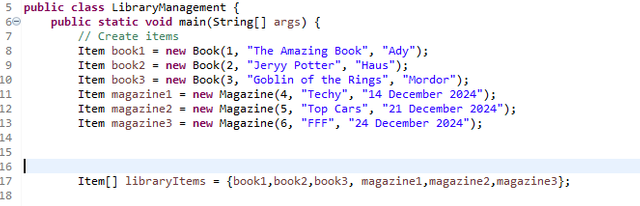
Now using a scanner and some options presented to the user, we are going to apply different actions to our library. Using a while loop and a switch we allow the user to borrow, return, or list all the available items. This is how the menu of options looks like.
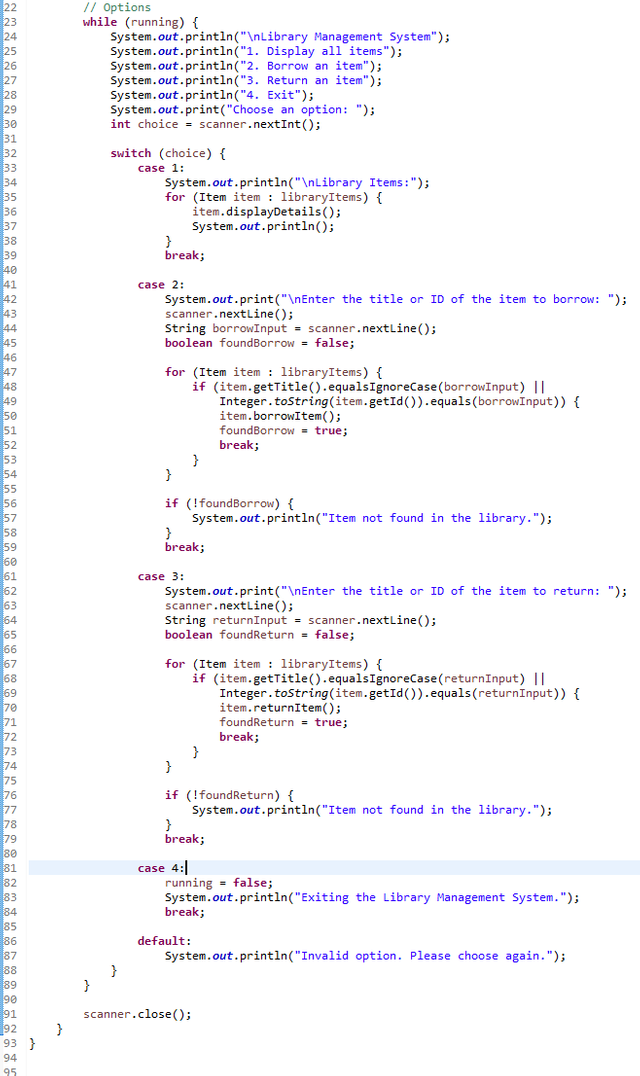
Now let's see everything in action.
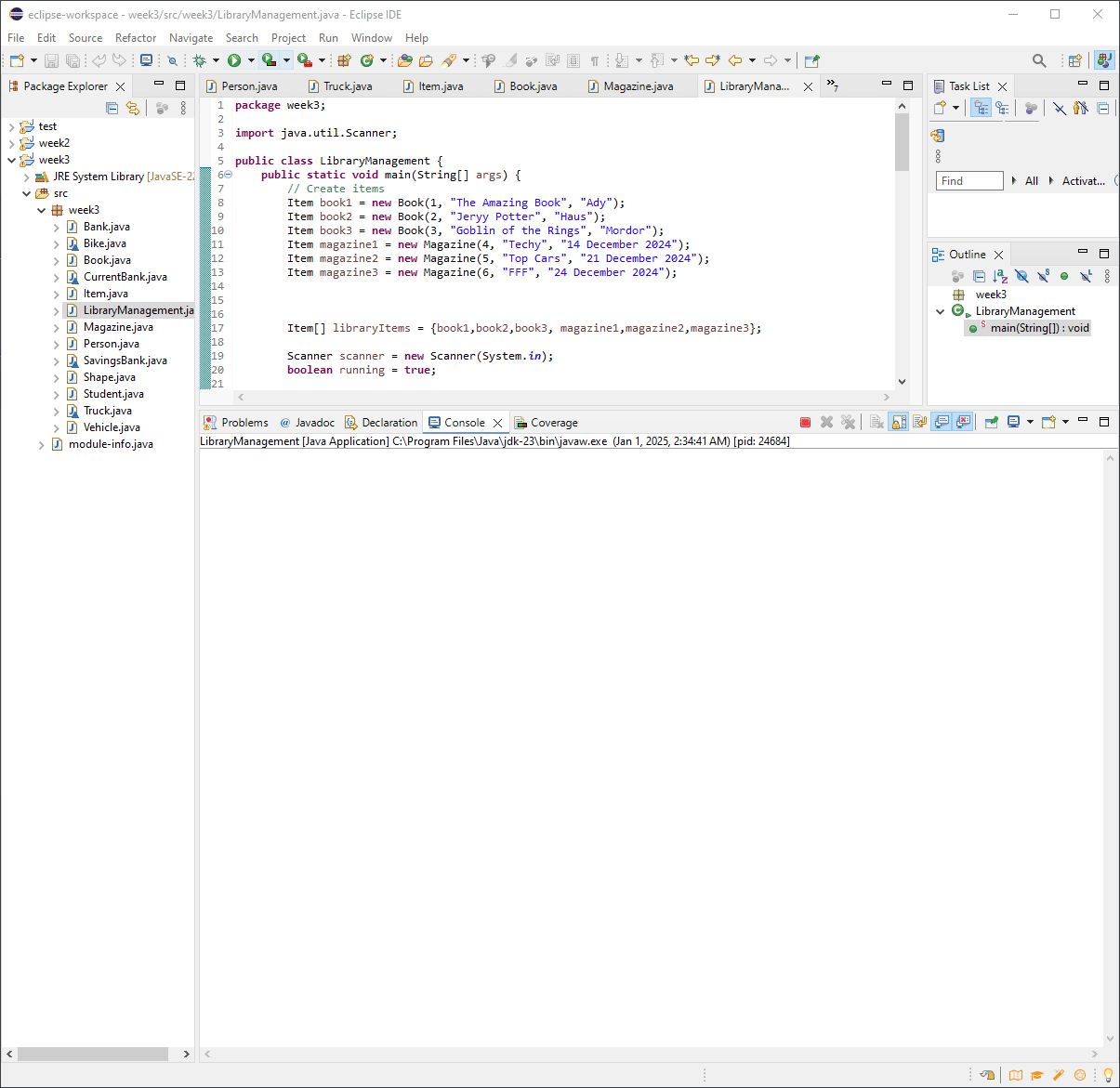
You can see we can borrow an item with the id
or name
, once we borrowed it, it shows in the list that is not available, we can also return it with id
or name
and also if it's borrowed you can't take it and borrow again.


Employee Management System:
An organization employs three types of workers: contractual employees, permanent employees, and hourly workers. The following data is tracked:
- Contractual employees: receive a fixed monthly salary.
- Permanent employees: receive a base salary plus a performance bonus.
- Hourly workers: paid based on the number of hours worked and the hourly rate.
Tasks:
- Design a class hierarchy to represent the relationship between
Employee
,HourlyWorker
,ContractualEmployee
, andPermanentEmployee
. Indicate attributes and methods. - Define the base class
Employee
with attributes such asname
,CIN
,address
, andsalary
, and a methoddisplay()
. - Define the derived classes to include specific attributes for salary calculation, a
calculateSalary()
method, and adisplay()
method. - In the main program, create a vector to hold three employees of different types and display their details.
Let's start by creating an abstract (a blueprint) Employee
to use for next classes. This abstract will define layout of our other classes. Here we define the constructor, attributes and methods, like this:
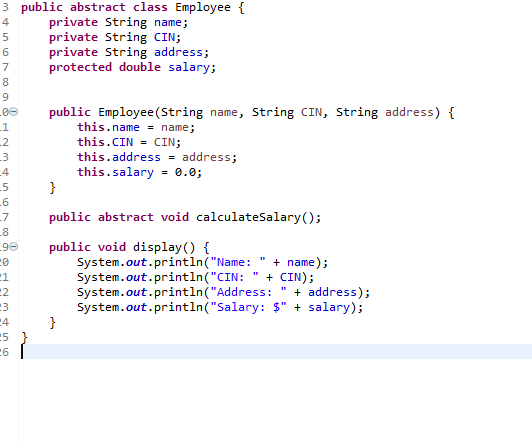
Now that we have a layout set time to create the other Employee classes and the management, lets go over the Employee classes first, starting with HourlyWorker
.
In the HourlyWorker
class we get two new attributes hoursWorked
and hourlyRate
, we declare them and use in the constructor. Also now that we have hours worked and hourly rate we need to Override
the calculateSalary
and also the display
so we know what Employee we are working with.
This is how the HourlyWorker
looks like:
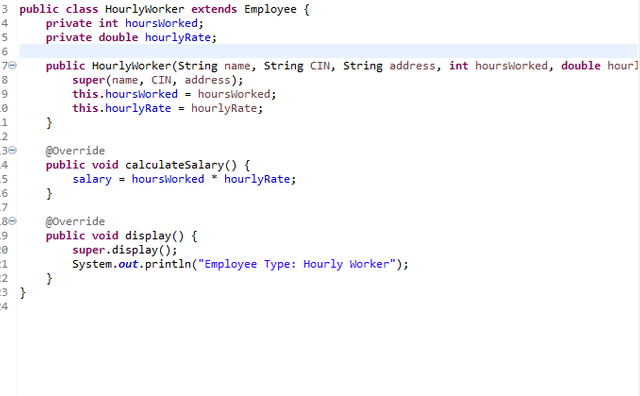
Now for the ContractualEmployee
we are going to use an attribute fixedSalary
that will be updated from the Management class and applied to all Contractual Employees because in this case all have a fixed salary. That's why here we are going to create setter/getter .
This is how it looks like:
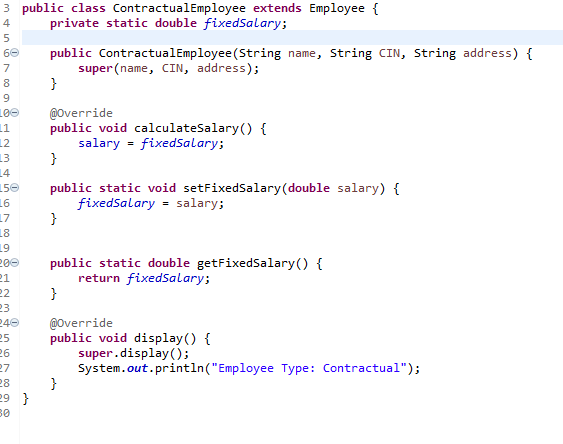
For the PermanentEmployee
we are going to add two attributes and use them in the constructor, also we Override
the calculateSalary
to get the salary for this class and also the display
to know which Employee is this one.
Code looks like this:
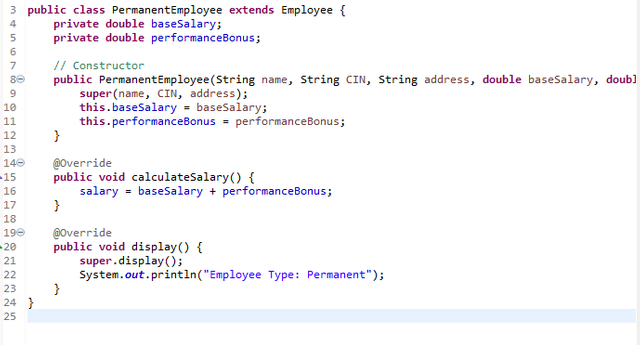
In the end we need a Management class to handle all these, creating EmployeeManagementSystem
. Here we set the fixedSalary
for the ContractualEmplyee
, we define an array and add employees into it. Now about the objects being created, as you can see in the screenshot below, based on the type of Employee we are creating, different attributes are used/or not in the constructor.
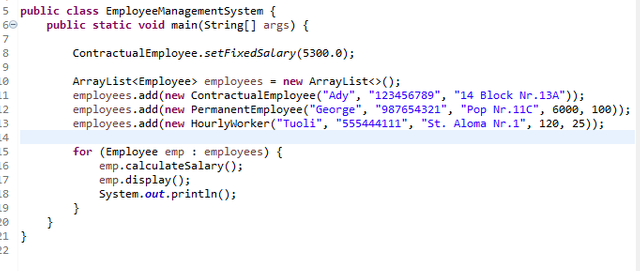
And now the code in action, GIF:
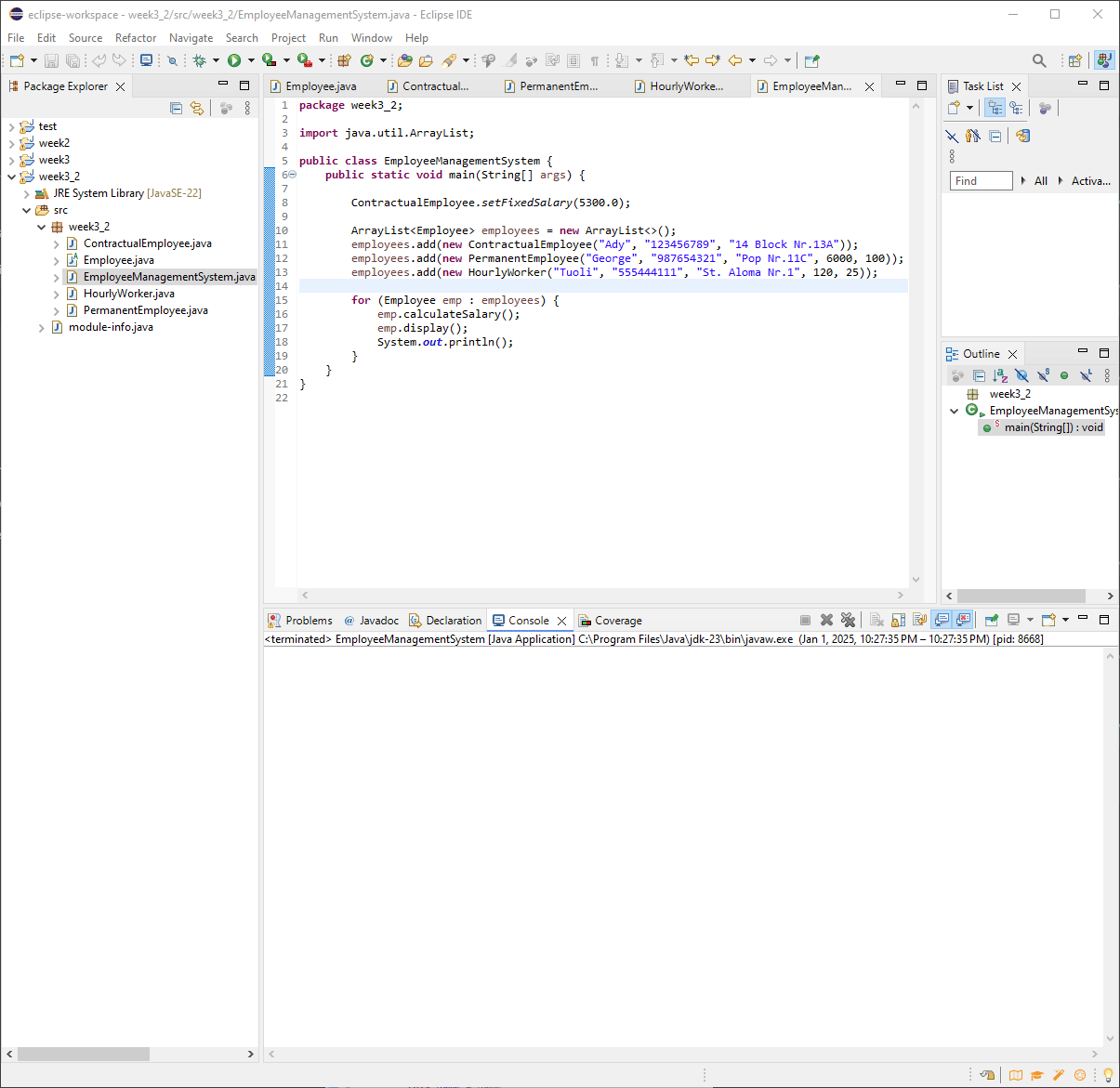
A diagram of the classes should look like this:
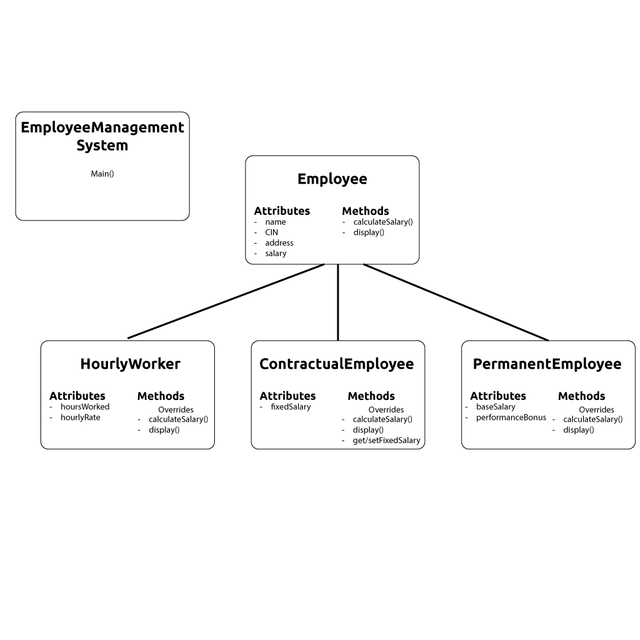


Understanding Class Relationships:
- Given the following class definitions, analyze whether the instructions 1 to 8 in the main method are valid. If invalid, specify whether the error occurs at compilation or runtime.
class Test {
public static void main(String args[]) {
C1 o1 = new C1();
C1 o2 = new C11();
C111 o3 = new C111();
C11 o4 = new C111();
C1 o5 = new C111();
o1 = o2; // Instruction 1
o1 = o3; // Instruction 2
o3 = o1; // Instruction 3
o4 = o5; // Instruction 4
o3 = (C111) o1; // Instruction 5
o4 = (C11) o5; // Instruction 6
o4 = (C111) o2; // Instruction 7
o3 = (C11) o5; // Instruction 8
}
}
class C1 {}
class C11 extends C1 {}
class C111 extends C11 {}
So from the code provided we have the following:
C1
as the base classC11
that extendsC1
C111
that extendsC11
and also by thisC1
Let's go through the cases where an error occurs:
Instruction 3
o3 = o1
,o1
is declared asC1
whileo3
asC111
, we can't assignC1
toC111
without an explicit cast, in this case we'll get a Compilation error. To avoid the error it should be written likeo3 = (C111) o1
. Still runtime errors might occur if o1 is not an instance of C111.Instruction 4
o4 = o5
, same as in the example above, we are getting an error during the compilation, to fix it we need to explicit cast it like thiso4 = (C111) o5
, also runtime error might occur.Instruction 5
o3 = (C111) o1
, in this case we have the explicit casting but we still need a check to see ifo1
is an instance ofC111
, otherwise we can get errors at runtime.Instruction 7
o4 = (C111) o2
, same as the one above, we need to check ifo2
is an instance ofC111
and only if true we explicit cast it, otherwise it's a runtime error.Instruction 8
o3 = (C11) o5
in this case it's a compilation error, we need to explicit cast it to(C111)
like thiso3 = (C111) (C11) o5
.
All the other instruction not mentioned should be valid.
Not sure if @kouba01 picked these name intentionally but I feel dizzy after C1
C11
C111
left and right.


Geometric Inheritance:
- Create a Point class with attributes x (abscissa) and y (ordinate). Include constructors Point() and Point(x, y). Add getters, setters, and a toString() method.
- Create a Rectangle class inheriting from Point with attributes length and width. Include constructors Rectangle() and Rectangle(x, y, length, width). Add getters, setters, and methods area() (calculate the area) and toString() (override).
- Create a Parallelogram class inheriting from Rectangle with an additional attribute height. Include constructors Parallelogram() and Parallelogram(x, y, length, width, height). Add getters, setters, and methods area() (override), volume(), and toString() (override).
Write a test class to demonstrate the functionalities of all three classes.
Last but not least after fighting 7 tasks we are at the final one, lets see what do we do here.
Starting with the Point
class, we have x
and y
as attributes, the Point
constructor and setters/getters for x
and y
, also a toString
method to print the x
and y
.
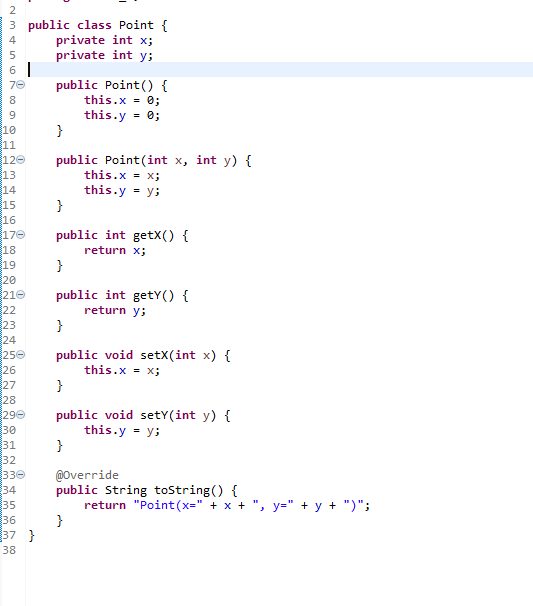
Now for the Rectangle
class, we have the length
and width
as attributes, the constructor where we call the Point
constructor in the Rectangle
one, the one with no parameters, in the second constructor we are using the Point
one with parameters along length
and width
. We have setters and getters for length and width an area
method and the override method toString
. Here I also included the main, but we talk about it in the end when we test everything.
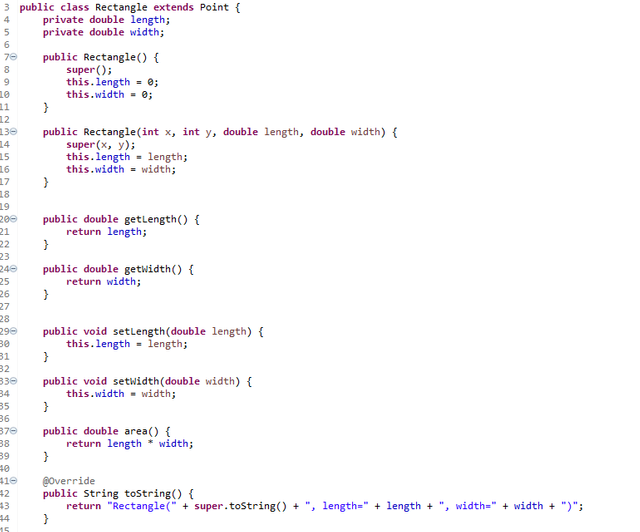
For the Parallelogram
class, we define the height
as an attribute, two constructors one with parameters one without, setters and getters, an override area
and toString
and also a method for volume
.
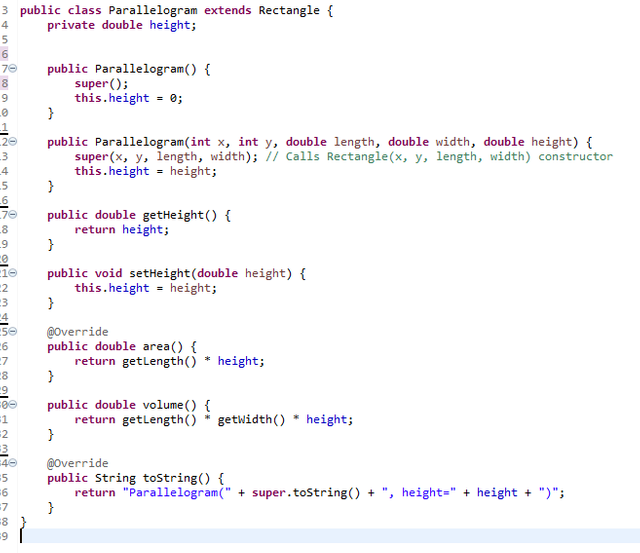
Now to test all these, I've added the Main
inside the Rectangle class to avoid creating a new one just for some testing, I've created a few objects inside, it looks like this:
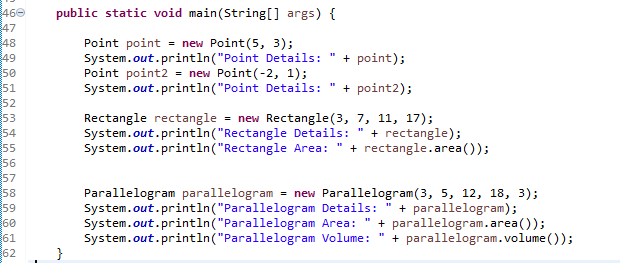
And a GIF representation of the code:
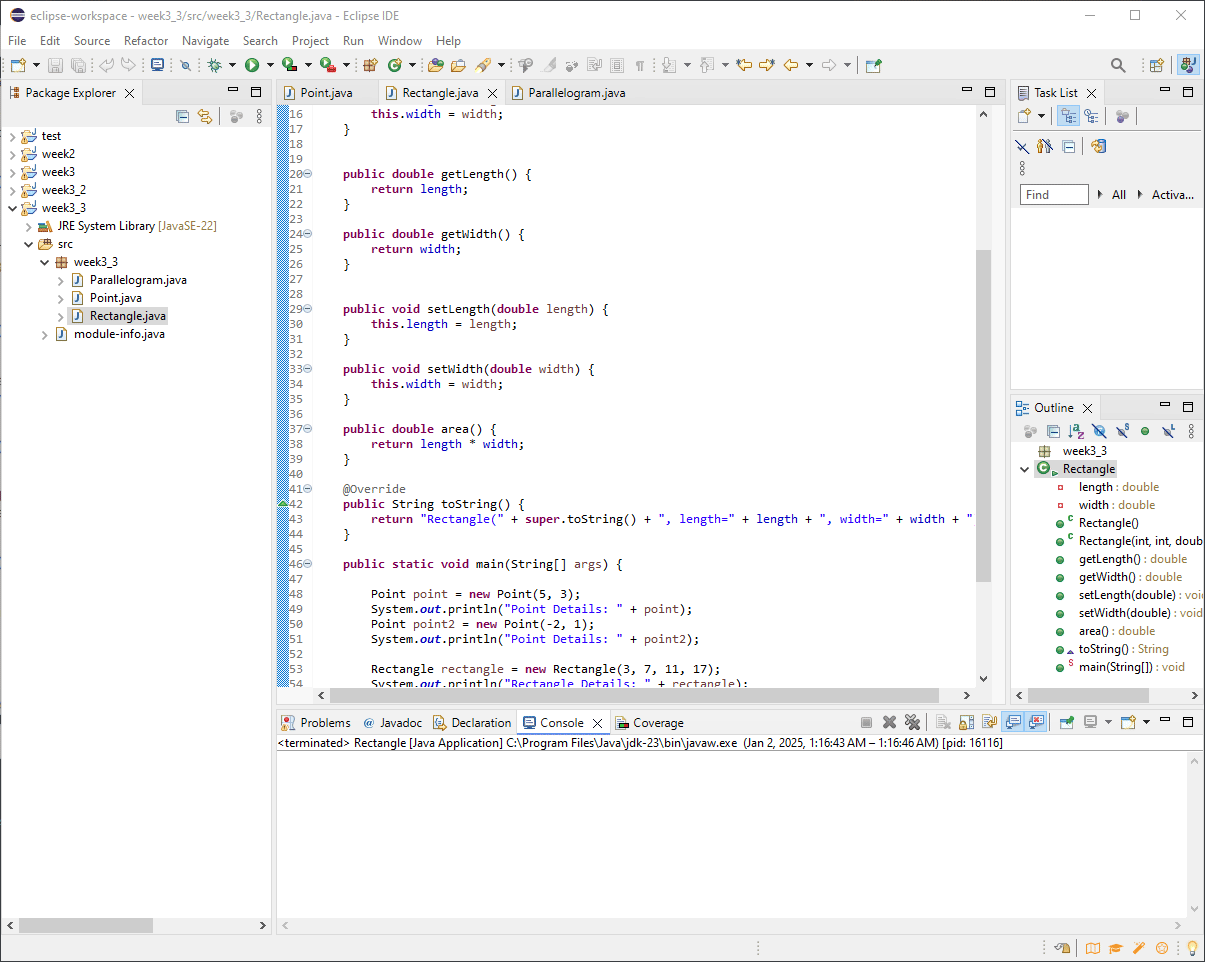
I know there is this thing in output Parallelogram Details: Parallelogram(Rectangle(Point(x=3, y=5), length=12.0, width=18.0), height=3.0)
Point
inside Rectangle
inside Parallelogram
but it happens because I've added the string in Override of toString
.
It's just a string visual thing, the numbers behind are ok.

Now that was it for this Java homework, thank you @kouba01 again for creating these classes, in the end I'd like to invite @titans, @r0ssi and @mojociocio to try it!
Until next time, have a great day and a happy new year!
Good points!
This article is now being scheduled for featuring on our WOX channel on Telegram, https://t.me/woxchannel.
Everyone is also invited to join ChatSteemBot on Telegram through this link: https://t.me/SteemBot or scan the QR Code on the flyer below. Thanks.
World of Xpilar Telegram Channel | Chat SteemBot on Telegram
Everyone can support this initiative by delegating some SP to @sbsupport. Thanks in advance.
50SP | 100SP | 200SP | 500SP | 1000SP | 2000SP | 5000SP