SEC S20W4 - Interactive UI Elements in Flutter: Crafting Dynamic Layouts with Card, Scrolls, and ClipRRect in Flutter
Hello everyone!
You are warmly welcome to the 4th week of Steemit Engagement Challenge Season 20. Here you will learn:
- Card
- SingleChildScrollView
- Scroll Types
- ClipRRect
So let's start.
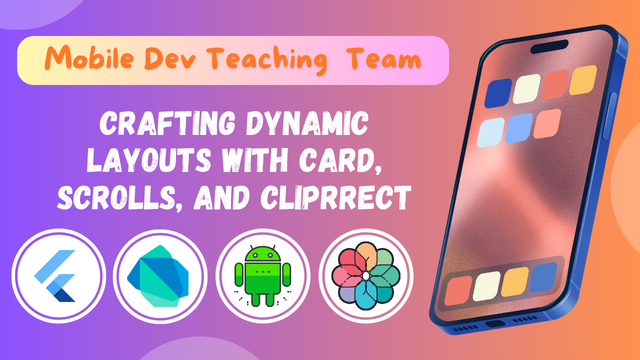
Card
The card in flutter is a widget. It is a material design which provides a ready made container with sightly rounded corners. It provides elevation to create a shadow. We can use it to group related information. It makes the content look organized and distinct.
Key Features of the Card Widget
Elevation: The card widget has an
elevation
property. It defines the height of the shadow below the card. So it gives a raised effect to the card.Rounded Corners: Card widget has rounded corners. Furthermore we can adjust the corners by using its border radius property. We can make the border to look more rounded or less.
Child: We can add any widget in the card widget in the card such as Text, Image or any other complex layouts.
Padding and Margin: We can use padding and margin in the card to make it look more organized and beautiful. It will help for the alignment of the content inside the card widget.
Color: We can change the color of the card by using its built in
color
property.
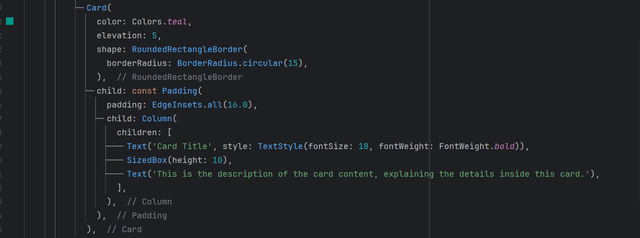
This is the simple syntax of the card widget where I have used all of its properties. I have added color: Colors.teal
to make the card look more beautiful. Th elevation of the card is adding shadow to the car widget. You can see that how we can manage the shape of the card by making its corners round. I have implemented all the properties which I have explained. You can also use these properties and ca make the card look more attractive.
Use Cases
- Displaying user profiles or information.
- Presenting content like news articles or products in a list.
- Organizing different sections of data in a visually structured format.
We can use card widget for the multiple purposes. Here are some examples of the cards:
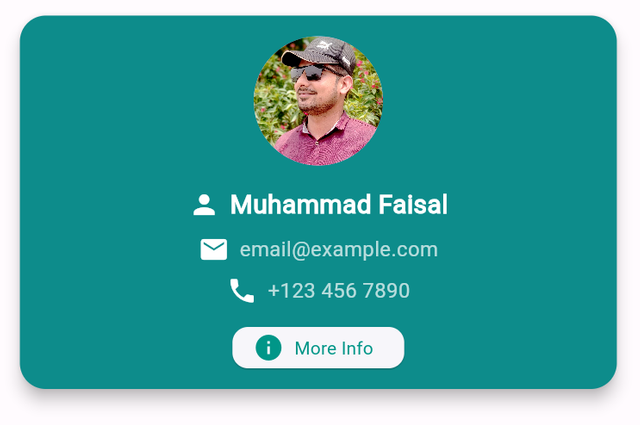
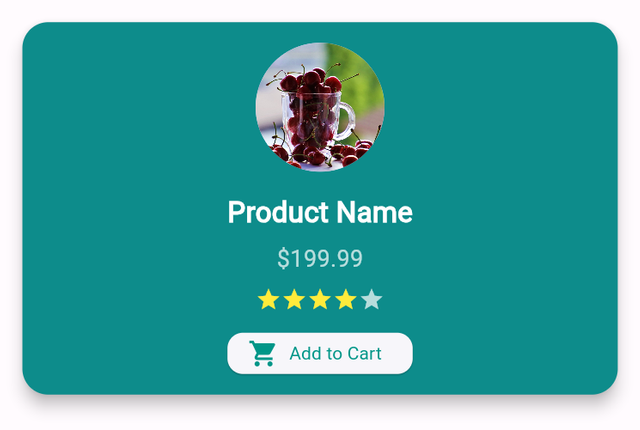
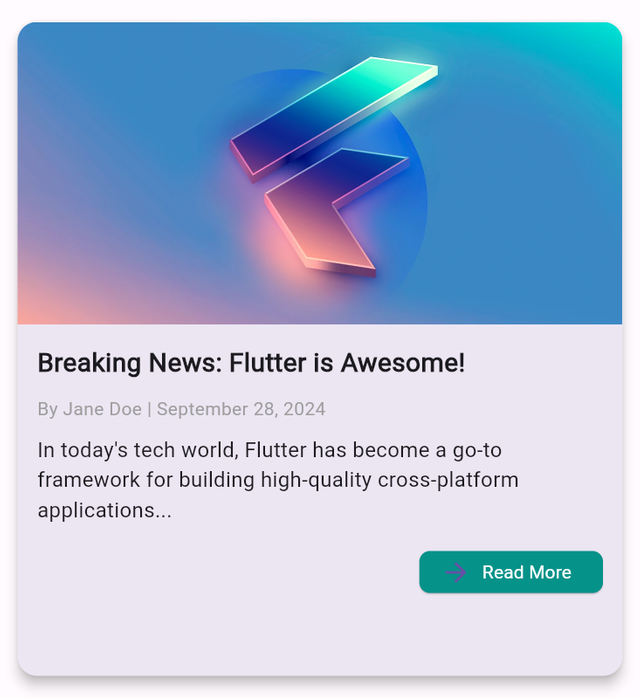
In this way we can use card widget for different purposes to make the required layouts.We can customize the card widget easily to fit according to the needs of the layout. So from this flexible and powerful widget we can create structured and modern layouts.

SingleChildScrollView
Scrolling is significant for every screen on the application. In the last lectures we made a scrollable list with the help of the ListView
. Actually this widget offers built-in scrolling and we can create a scrollable list. But if we do not need any list and we want to place different widget and data on the screen then we will not be able to use ListView.
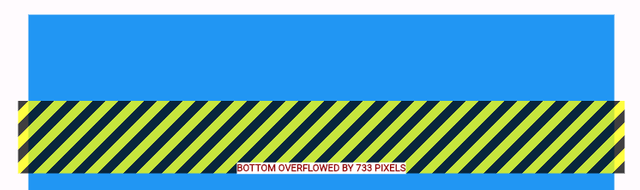
In the previous lecture while doing homework some users faced the error of bottom overflowed. Actually their content was more than the screen and it was overflowing from the screen. And it makes our layout fail to look beautiful. If we want to add containers, cards, images, texts and icons and the screen is overflowing then we need to fix it.
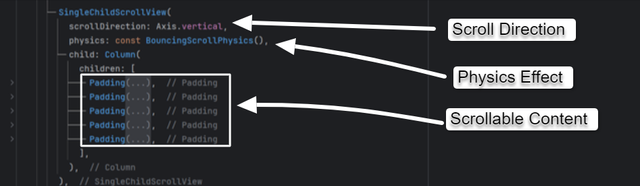
We will fix the screen overflow error with SingleChildScrollView
. It makes all the content scrollable and to take the size according to the content on the screen. It helps to create a responsive layout and design.
Features of SingleChildScrollView
Single Child: This widget can have only a single direct child as you can guess from the name as well. We can wrap our
column
androw
widget with this to make the content inside them scrollable.Vertical Scrolling: By default SingleChildScrollView scrolls the items in the vertical direction. We don't need to mention it's vertical scrolling and it will scroll automatically.
Horizontal Scrolling: SingleChildScrollView can also scroll the items horizontally. But for this we have to give commands and direction to it in this properties.
Properties
SingleChildScrollView has following properties:
scrollDirection: The most useful property is
scrollDirection
. With this we can set the scrolling direction either in vertical or horizontal.scrollDirection: Axis.vertical
is used to scroll things vertically and it is by default.scrollDirection: Axis.horizontal
is used to scroll things horizontally.reverse: This property helps us to reverse the direction of the scrolling. We can scroll from bottom to top in vertical scrolling and similarly right to left in horizontal scrolling.
padding: It is used to add space around the child widget.
controller: This property is used to control the scrolling behaviour with the help of the programming.
physics: It controls that how the scroll view should behave when the user interacts with it such as we can make it bouncing or clamping.
![]() | ![]() | ![]() |
---|---|---|
Vertical Scroling | Horizontal Scrolling | Bouncing Scrolling |

ClipRRect
This is another layout widget. It is used to to clip its child widgets with rounded corners. We can use any custom border radius for the child widget. Mostly we use this widget to create rounded images and buttons. We can use any content to give it a specific shape.
Features of ClipRRect
Rounded Corners: The main purpose of the
ClipRRect
is to apply rounded corners to its child widget. It is done by using itsborderRadius
property.Clipping: It ensures that any part of the child widget which goes outside of the parent widget will be cut off. It will be clipped automatically. It will make the widget to look like fit by rounding the corners of the child widget.
Custom Border Radius: We can define the rounding of the corners by ourselves according to the needs of the layout and design.
Properties of ClipRRect
It has two properties:
borderRdaius: It fixes the border radius for the corners. WE can use predefined methods such as
BorderRadius.circular()
or customize each corner withBorderRadius.only()
.child: The widget that will be clipped within the rounded rectangle.
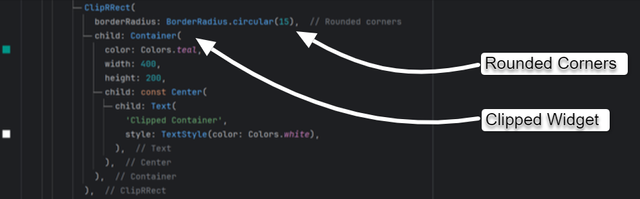
ClipRRect
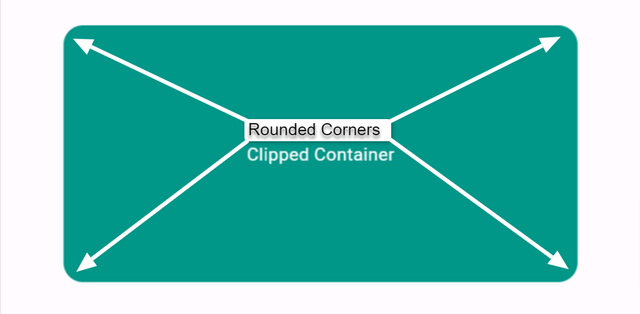
Similarly this container widget we can wrap any widget with ClipRRect
widget to make the corners of the widget look round. And it makes the layout look beautiful.
![]() | ![]() |
---|
This is another example of ClipRRect
where I have used an image from Pixabay as a child widget of the ClipRRect
widget. You can see difference between both the outputs. In one there is no use of the ClipRRect
widget and in the second one I have used ClipRRect
. And the corners are looking round than the first image.
In this way we can use CilpRRect
widget to wrap any other widget to make that look more beautiful with rounded corners and we can change the radius to make the borders look more or less round.

Homework Assignments
Create a Profile Card: Design a user profile card using the Card widget. It should include an avatar image, a username, and a description. Use the elevation, color, padding, and margin properties to style the card. Show your creativity and you will get marks based on the creativity and beautiful layout of the profile card. (2 Points)
Task Title: How to create a user profile card layout in flutter?
Create Weather Card: The design of the weather card is given below make it correctly as shown in the below image. Add all the widgets used in the card. (2 Points)
Task Title: How to create a weather card layout in flutter?
Scrollable Image Gallery: Implement a horizontal scrolling image gallery. The gallery should display a series of images that can be scrolled through horizontally. You can use asset images or network image. (2 Points)
Task Title: How to create a scrollable image gallery in flutter?
Project:
- Build a news feed screen where each news item is displayed in a Card widget. The entire list of news should be scrollable. Each card should has a cover image, title, description of the news, date, author and a button to read more. ( 4 Points)
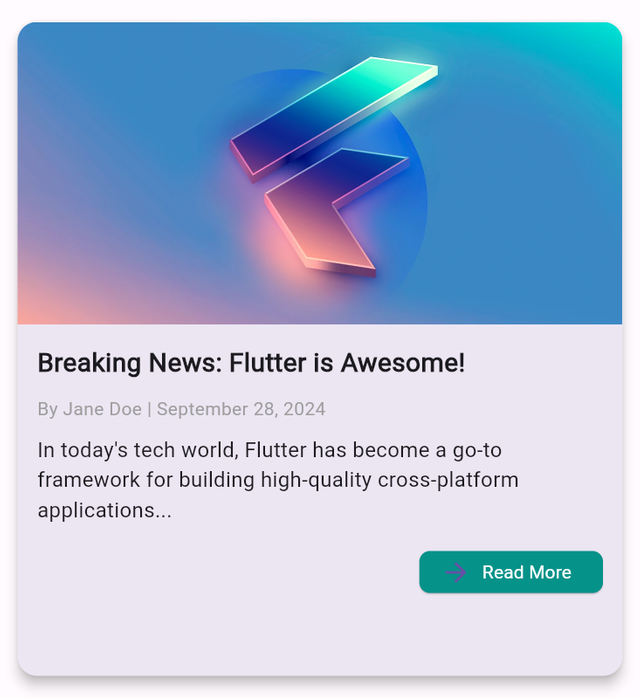
Each card widget of the news feed application should look like this.
Task Title: How to create news feed user interface in flutter?
Note: Please use an IDE and your own mobile phone to complete all the tasks. And present video of the tasks by making gifs. You can make gif of the video using Ezgif.

Contest Guidelines
Post can be written in any community or in your own blog.
Post must be #steemexclusive.
Use the following title: SEC S20W4 - Crafting Dynamic Layouts with Card, Scrolls, and ClipRRect in Flutter
Participants must be verified and active users on the platform.
The images used must be the author's own or free of copyright. (Don't forget to include the source.)
The participation schedule is between Monday, September 30 , 2024 at 00:00 UTC to Sunday, October 6, 2024 at 23:59 UTC.
The publication can be in any language.
Plagiarism and use of AI is strictly prohibited.
Participants must appropriately follow club status such as #club5050 or #club75 or #club100.
Use the tags #flutterdev-s20w4 , #country (example - #Pakistan, #Nigeria), #steemexclusive in your first 4 tags.
Use the #burnsteem25 tag only if you have set the 25% payee to @null.
Post the link to your entry in the comments section of this contest post. (Must)
Invite at least 3 friends to participate in this contest.
Try to leave valuable feedback on other people's entries.
Share your post on Twitter and drop the link as a comment on your post.

Rewards
SC01 would be checking on the entire 16 participating teaching teams and challengers and upvoting outstanding content. Upvote is not guaranteed for all articles. Kindly take note.
At the end of the week, I would nominate the top 5 users who had performed well in the contest and would be eligible for votes from SC01/SC02.
Important Notice: The nomination of the top 5 users is solely based on the quality of the post. So focus on the quality and creativity of the post. Also ensure your quality engagement.
Flutter Mobile Application Dev Team (mohammadfaisal)

Upvoted! Thank you for supporting witness @jswit.
X Promotion: https://x.com/stylishtiger3/status/1840270085216166164
It is another wonderful lecture and all the tasks are looking very interesting including the project. I'll surely do my best to complete all the tasks.
You are welcome to join it.
My link.
https://steemit.com/flutterdev-s20w4/@josepha/sec-s20w4-crafting-dynamic-layouts-with-card-scrolls-and-cliprrect-in-flutter
https://www.steempro.com/hive-145157/@sergeyk/sec-s20w4-interactive-ui-elements
https://steemit.com/flutterdev-s20w4/@alejos7ven/sec-s20w4-crafting-dynamic-layouts-with-card-scrolls-and-cliprrect-in-flutter
My Participation: https://steemit.com/flutterdev-s20w4/@akmalshakir/sec-s20w4-crafting-dynamic-layouts-with-card-scrolls-and-cliprrect-in-flutter