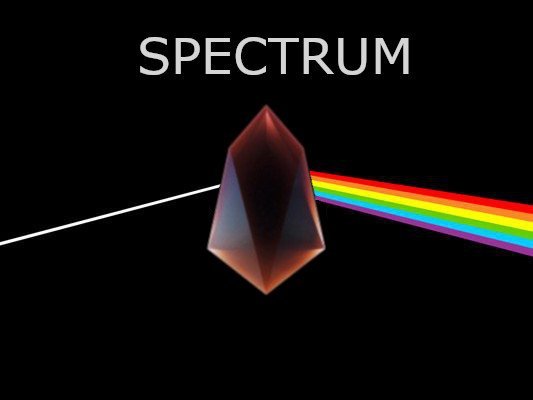
EOS Tribe launched a beta version of it's web-socket streaming Spectrum API on Telos Testnet (https://www.telosfoundation.io/).
Telos Testnet Spectrum API web-socket endpoint: wss://testnet.telos.eostribe.io
Sample HTML/JS Client code for Get Actions:
<html>
<head><title>Spectrum Web Sockets</title>
<script>
let socket = new WebSocket("wss://testnet.telos.eostribe.io/streaming");
var actionsList = ["transfer","buyram"];
var messageBody = {
"apikey":"test-api-key",
"event":"subscribe",
"type":"get_actions",
"data": {"account":"eosio"}
};
socket.onopen = function(e) {
console.log("[open] Connection established");
console.log("Sending to server: "+JSON.stringify(messageBody));
socket.send(JSON.stringify(messageBody));
};
socket.onmessage = function(event) {
html_log("[message] Data received from server: "+event.data);
};
socket.onclose = function(event) {
if (event.wasClean) {
html_log("[close] Connection closed cleanly, code=${event.code} reason=${event.reason}");
} else {
html_log("[close] Connection died");
}
};
socket.onerror = function(error) {
html_log("[error] ${error.message}");
};
function html_log(data) {
var divLog = document.getElementById("log");
divLog.innerHTML += "<p>"+data+"</p>";
}
</script>
</head>
<body>
<h2>Spectrum Web Sockets Test: Get Actions</h2>
<div id="log"></div>
</body>
Sample HTML/JS Client code for Get Blocks:
<html>
<head><title>Spectrum Web Sockets</title>
<script>
let socket = new WebSocket("wss://testnet.telos.eostribe.io/streaming");
var messageBody = {
"apikey":"test-api-key",
"event":"subscribe",
"type":"get_blocks"
};
socket.onopen = function(e) {
html_log("[open] Connection established");
html_log("[subscribe] sending message to server");
socket.send(JSON.stringify(messageBody));
};
socket.onmessage = function(event) {
html_log("[message] Data received from server: "+event.data);
};
socket.onclose = function(event) {
if (event.wasClean) {
html_log("[close] Connection closed cleanly, code=${event.code} reason=${event.reason}");
} else {
html_log("[close] Connection died");
}
};
socket.onerror = function(error) {
html_log("[error] ${error.message}");
};
function html_log(data) {
var divLog = document.getElementById("log");
divLog.innerHTML += "<p>"+data+"</p>";
}
</script>
</head>
<body>
<h2>Spectrum Web Sockets Test: Get Blocks</h2>
<div id="log"></div>
</body>
Sample HTML/JS Client code for Get Transaction:
<html>
<head><title>Spectrum Web Sockets</title>
<script>
let socket = new WebSocket("wss://testnet.telos.eostribe.io/streaming");
var messageBody ={
"apikey":"test-api-key",
"event":"subscribe",
"type":"get_transaction",
"data": {"account":"eosio"}
};
socket.onopen = function(e) {
console.log("[open] Connection established");
console.log("Sending to server: "+JSON.stringify(messageBody));
socket.send(JSON.stringify(messageBody));
};
socket.onmessage = function(event) {
html_log("[message] Data received from server: "+event.data);
};
socket.onclose = function(event) {
if (event.wasClean) {
html_log("[close] Connection closed cleanly, code=${event.code} reason=${event.reason}");
} else {
html_log("[close] Connection died");
}
};
socket.onerror = function(error) {
html_log("[error] ${error.message}");
};
function html_log(data) {
var divLog = document.getElementById("log");
divLog.innerHTML += "<p>"+data+"</p>";
}
</script>
</head>
<body>
<h2>Spectrum Web Sockets Test: Get Transactions</h2>
<div id="log"></div>
</body>
See a working example on EOS Tribe website: https://eostribe.io
Feel free to give it a try and provide your feedback at out Telegram channel: https://t.me/EOSTribe
EOS Tribe is committed to continue work on creating innovative solutions for EOSIO.