My code to encrypt any data
- First, we'll need to install Node.js https://nodejs.org/en
- I also like to use VSCODE program for code and there is also a handy terminal there through which you can run the code https://code.visualstudio.com/.
- Create a folder named cryptor. Right-click inside the empty folder and open this folder with VSCODE.
- Create a file named package and extension .json. Full name of package.json
- Create a file named cryptor and extension .js. Full name is cryptor.js
- Let's add some code to the package.json file:
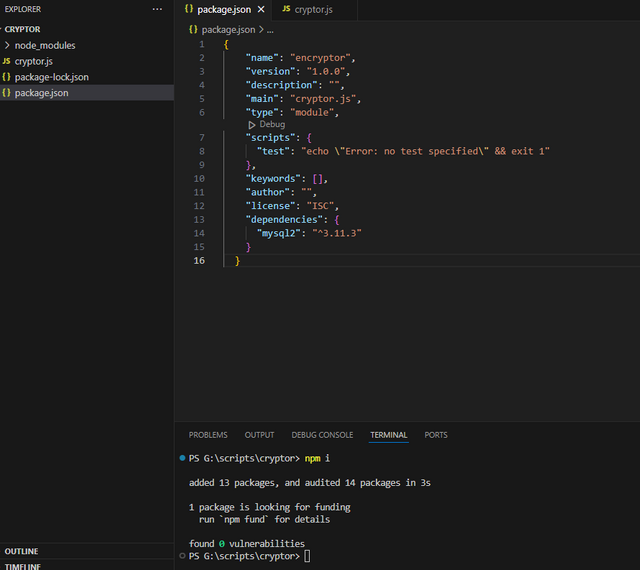
{
"name": "encryptor",
"version": "1.0.0",
"description": "",
"main": "cryptor.js",
"type": "module",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"mysql2": "^3.11.3"
}
}
Let's launch a new terminal through the menu. Terminal -> New Terminal. Install dependencies for node.js with the command:
npm i
To do this, paste the command into the terminal and press Enter
I have prepared 2 versions of the code, to work with and without databases.
Database version.
Use this version if you know how to work with a database, if not skip it and go to the next paragraph.
Let's add the code to the cryptor.js file:
import { createHash, randomBytes, createCipheriv, createDecipheriv } from 'crypto';
import mysql from 'mysql2/promise';
const config = {
host: "",
user: "",
database: "",
password: "",
connectionLimit: 20,
connectTimeout: 60000
}
const pool = mysql.createPool(config);
const date = 0; // Any number
const month = 0; // Any number
const year = 0; // Any number
const salt = 'ilonma777#!'; // Any data string
const phrase = 'ilonma best password'; // The data string to be encrypted
const ENCRYPT_MODE = true; // true - encrypt, false - decrypt
const decryptID = 1; // Decrypt ID
function createKey(date, month, year, salt) {
const baseString = `${date}${month}${year}${salt}`;
return createHash('sha256').update(baseString).digest();
}
function encrypt(phrase, key) {
const iv = randomBytes(16);
const cipher = createCipheriv('aes-256-cbc', key, iv);
let encrypted = cipher.update(phrase, 'utf8', 'base64');
encrypted += cipher.final('base64');
const combinedData = iv.toString('base64') + ':' + encrypted;
return combinedData;
}
function decrypt(combinedData, key) {
try {
const [ivBase64, encryptedData] = combinedData.split(':');
if (!ivBase64 || !encryptedData) {
throw new Error("Incorrect data");
}
const iv = Buffer.from(ivBase64, 'base64');
const decipher = createDecipheriv('aes-256-cbc', key, iv);
let decrypted = decipher.update(encryptedData, 'base64', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
} catch (error) {
console.error('Error:', error.message);
return 'Failed to decrypt';
}
}
if(ENCRYPT_MODE){
try {
const key = createKey(date, month, year, salt);
const encryptedResult = encrypt(phrase, key);
console.log('Encrypted phrase:', encryptedResult);
const conn = await pool.getConnection();
try {
const query = 'INSERT INTO mywallets (password) VALUE (?);';
await conn.execute(query, [encryptedResult]);
console.log('Encrypted password successfully inserted into the database.');
} finally {conn.release();}
const decryptedPhrase = decrypt(encryptedResult, key);
console.log('Decrypted phrase:', decryptedPhrase);
} catch (error) {console.error('Error:', error);
} finally {process.exit(0);}
}
else{
try {
const key = createKey(date, month, year, salt);
const conn = await pool.getConnection();
try {
const query = `SELECT PASSWORD FROM mywallets WHERE id=${decryptID} LIMIT 1;`;
const [rows] = await conn.execute(query);
if (rows.length > 0) {
const { PASSWORD } = rows[0];
console.log('Encrypted password:', PASSWORD);
const decryptedPhrase = decrypt(PASSWORD, key);
console.log('Decrypted phrase:', decryptedPhrase);
} else {
console.log('Password not found');
}
} finally {conn.release();}
} catch (error) {console.error('Error:', error);
} finally {process.exit(0);}
};
The database structure is very simple and consists of at least 2 columns id, password. This is the minimum necessary for the program to work. But you can add your own columns at will, for example you can add column “name” and “comments”.
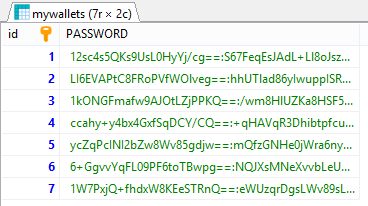
A version without a database.
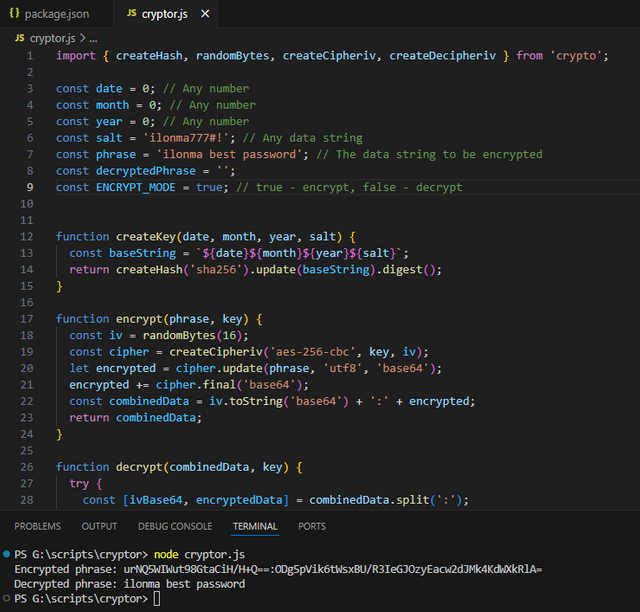
Let's add the code to the cryptor.js file:
import { createHash, randomBytes, createCipheriv, createDecipheriv } from 'crypto';
const date = 0; // Any number
const month = 0; // Any number
const year = 0; // Any number
const salt = 'ilonma777#!'; // Any data string
const phrase = 'ilonma best password'; // The data string to be encrypted
const decryptedPhrase = '';
const ENCRYPT_MODE = true; // true - encrypt, false - decrypt
function createKey(date, month, year, salt) {
const baseString = `${date}${month}${year}${salt}`;
return createHash('sha256').update(baseString).digest();
}
function encrypt(phrase, key) {
const iv = randomBytes(16);
const cipher = createCipheriv('aes-256-cbc', key, iv);
let encrypted = cipher.update(phrase, 'utf8', 'base64');
encrypted += cipher.final('base64');
const combinedData = iv.toString('base64') + ':' + encrypted;
return combinedData;
}
function decrypt(combinedData, key) {
try {
const [ivBase64, encryptedData] = combinedData.split(':');
if (!ivBase64 || !encryptedData) {
throw new Error("Incorrect data");
}
const iv = Buffer.from(ivBase64, 'base64');
const decipher = createDecipheriv('aes-256-cbc', key, iv);
let decrypted = decipher.update(encryptedData, 'base64', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
} catch (error) {
console.error('Error:', error.message);
return 'Failed to decrypt';
}
}
if(ENCRYPT_MODE){
const key = createKey(date, month, year, salt);
const encryptedResult = encrypt(phrase, key);
console.log('Encrypted phrase:', encryptedResult);
const decryptedPhrase = decrypt(encryptedResult, key);
console.log('Decrypted phrase:', decryptedPhrase);
process.exit(0);
}
else{
const key = createKey(date, month, year, salt);
console.log('Encrypted phrase:', decryptedPhrase);
const decrypted = decrypt(decryptedPhrase, key);
console.log('Decrypted phrase:', decrypted);
process.exit(0);
};
You can run this code with a command in the terminal:
node cryptor.js
Description of variables and general description of the script operation.
The program has 4 keys (date, month, year, salt) which are used for encryption and decryption. If at least 1 key is not correct, the encrypted phrase cannot be decrypted.
You can specify the date of birth of your grandmother or the birthday of President Lincoln, the main thing is to remember these data. In the key “salt” you can put any text string, the main thing do not forget that spaces and character case are also taken into account. For example, you can write the following string: “ My grandmother bakes very tasty pies “ or any other at your discretion.
The variable “phrase” is what you want to encrypt.
The variable “decryptedPhrase” is the encrypted phrase you want to decrypt (not used in encryption mode).
The variable “ENCRYPT_MODE” is a switch for the mode of the script. If “true” is specified, it will encrypt what is specified in the “phrase” variable. If “false” is specified, it will decrypt the phrase specified in the “decryptedPhrase” variable.
You will see the encrypted and decrypted phrase in the console and can copy it.
If you want to thank me, you can send one of 30 cryptocurrencies, to my address. The amount you can send at your discretion, I will be happy with any amount. If you have any questions, you can ask me below in the comments.
Address: 0xD8449C829B011aA4D2dbf4ed9A689fACedc6d90F
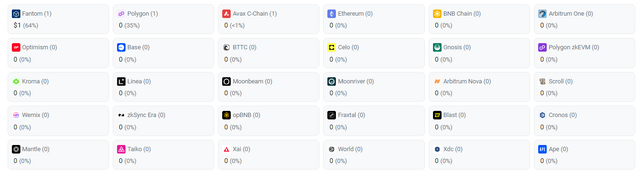
I wish you all a good day, thank you for your attention!