SLC S22 Week5 || Threads in Java: Life Cycle and Threading Basics
Hello Everyone
I'm AhsanSharif From Pakistan
Greetings to you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
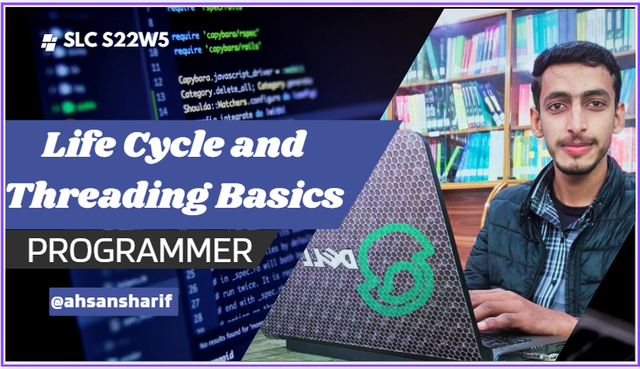
Design On Canva
01
Write a program that demonstrates how thread priorities affect execution order. Create three threads and assign them the priorities MIN_PRIORITY, NORM_PRIORITY, and MAX_PRIORITY. Run the threads and observe the execution order. Explain whether the thread priority influences their execution order and why. |
---|
First, we are writing a code explaining how thread priorities affect the implementation.
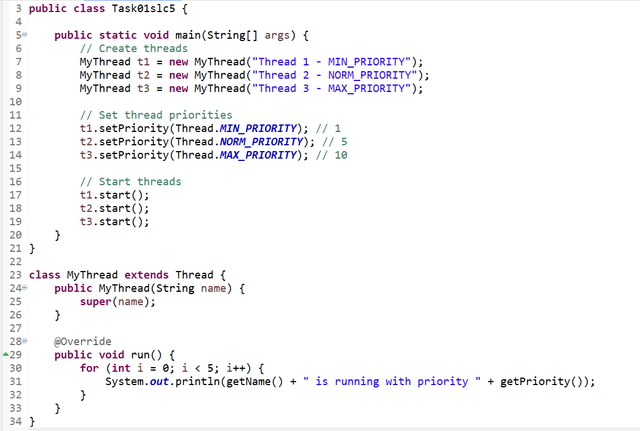
Execution:
Whenever we run our program, the higher-priority threads are scheduled first, ahead of the relatively lower-priority threads. However, this is not the same in all cases and may vary according to the operating system's thread schedule.
Output:
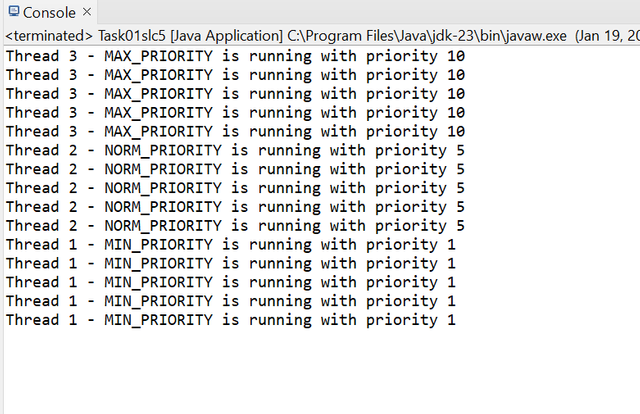
Does Thread Priority Affect Execution Order?
The priority of a thread can affect the performance of a process because these threads are competing for CPU time. Therefore, it is important that threads with higher priority run before threads with lower priority. We estimate our thread schedule through the operating system schedule.
In a time-sharing system, priority alone does not guarantee strict order, but threads with lower priority also get CPU time, although they may get less than them. In short, what this means is that the priority of a thread is a suggestion of the scheduler, it is not a fixed solution for it.
02
Develop a program that intentionally causes a deadlock using synchronized methods. Design a scenario where two or more threads attempt to acquire locks on shared resources in reverse order, leading to a deadlock. Provide a detailed explanation of how the deadlock occurs and suggest strategies to prevent it. |
---|
Here is a code that fulfills the question requirements:
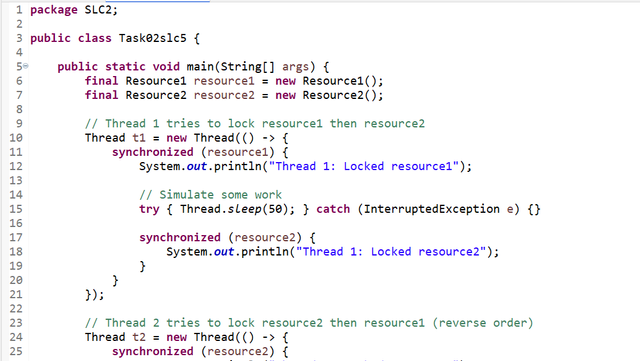
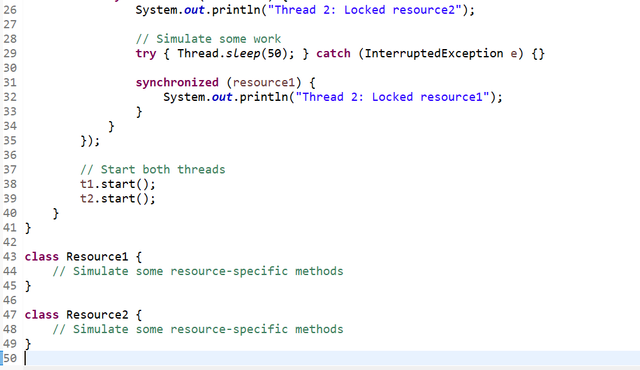
In this given program:
- First
Thread1
locksresource1
then locks toresource2
. - First
Thread2
locksresource2
then locks toresource1
.
Here we can see that one thread is holding one resource and waiting for another, but this creates a circular deadlock in which both threads are stuck in the loop and no progress is made between them.
Output:
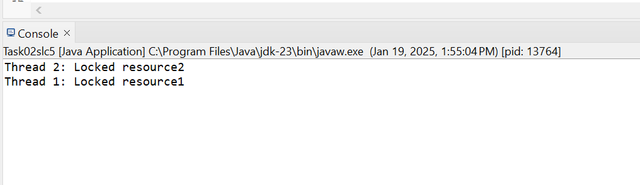
Prevent Deadlock:
Avoid Nested Locks: First of all, we should try to minimize the use of one or more locks or avoid nesting our blocks here. If possible, we need to limit one thread to one lock at a time.
Lock Ordering: To avoid circular dependencies, we need to ensure that both of our threads acquire locks in the same order, such that the first thread should lock resource one, and then resource two.
Time-outs: Use tryLock()
with a timeout to acquire the lock. The advantage of this is that if a thread does not get any locks at a certain time, it tries again using the ones it already has.
Deadlock Detection: Some advanced techniques track our locks to see which thread has which lock.
03
Create a program where multiple threads count from 1 to 100 concurrently. Ensure that the numbers are printed in the correct order without conflicts, even though the counting occurs in parallel. Describe the mechanisms (e.g., synchronization) used to maintain the correct sequence. |
---|
Here is a code that fulfills the question requirements:
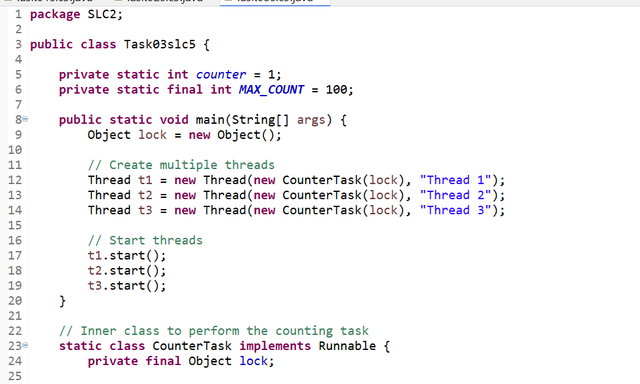
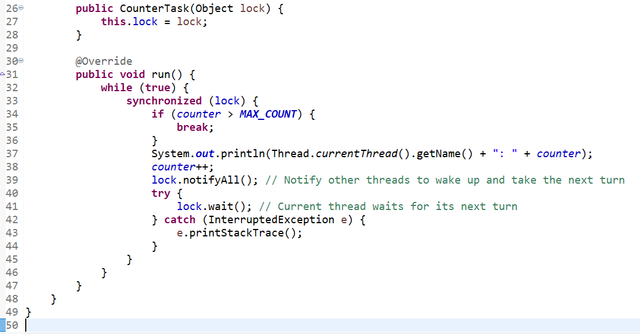
Mechanisms:
Synchronization: First, to access the counter, we use an object called a lock
. This allows us to synchronize shared resources. The advantage of this is that it ensures that only one thread counter value is updated or printed at a time.
notifyAll(): After printing the first counter, it then calls notifyAll()
the threads to shake up the next thread waiting and try to acquire the lock or continue the count.
wait(): After incrementing the counter, it then calls the thread wait()
to pause its execution and continue holding the lock, allowing other threads to continue acquiring the lock.
Output:
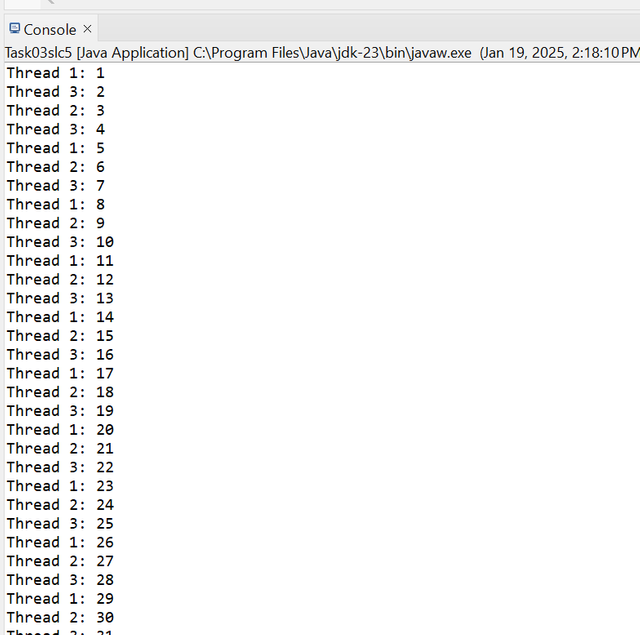
04
Write a program that uses a thread pool to process a list of tasks efficiently. Implement a set of simple tasks (e.g., displaying messages or performing basic calculations) and assign them to the threads in the pool. Explain how thread pools improve performance compared to creating threads for each task individually. |
---|
Here is a code that fulfills the question requirements:
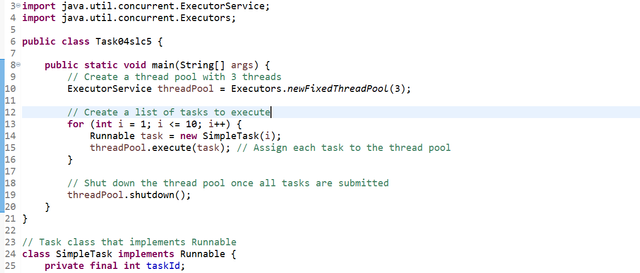
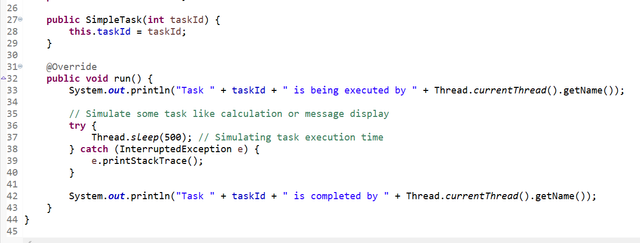
How Thread Pools Improve Performance?
Reusability of Threads: Thread pools are created with three threads using Executor.newFixedThreadPool(3). We will no longer need to create a new thread for each task. These three threads will handle multiple tasks. When the work of one thread is completed, then we will not create another thread, but it will become available for the next one without creating a new one.
Efficient Resource Management: It can be expensive for us in terms of CPU and memory to create and destroy our threads frequently, so the thread pool keeps the threads alive for a fixed number of times and keeps them ready to handle future tasks, thus reducing our costs.
Task Queuing: The job of the thread pool is to maintain the order of our work. If all the threads are busy then it keeps all the threads in a queue so that when resources are available it executes them one by one. This helps us to handle large numbers of tasks efficiently without exhausting the system resources.
Better Throughout: Reducing the number of threads reusing them and processing thread pool tasks at a stable rate is its best job. It improves throughput and also reduces the overload of our system.
Output:
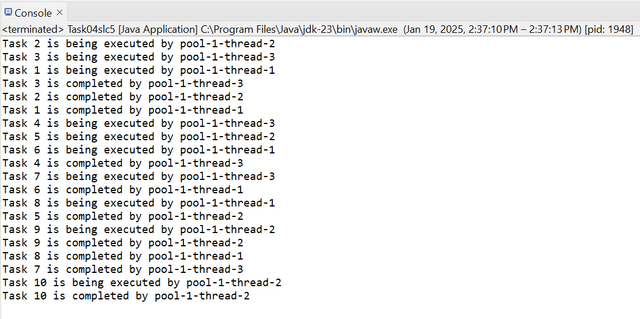
05
Write a program where multiple threads read different parts of a file simultaneously. Divide the file into distinct segments, assign each segment to a thread, and print the content read by each thread. Explain the logic used for dividing and assigning file segments to avoid conflicts and ensure efficiency. |
---|
Here is a code that fulfills the question requirements:
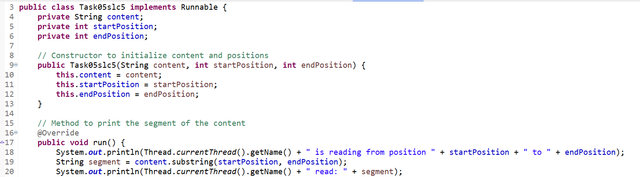
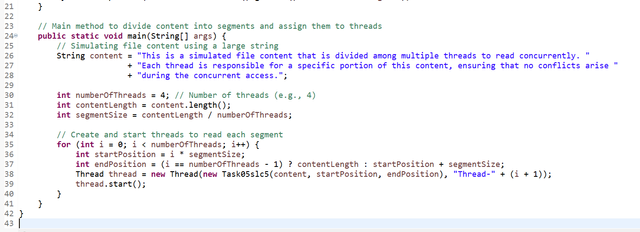
Explanation:
Simulated File Content: It copies the contents of a file into a string of content
. We can replace this string with any text we like.
Content Division: Here the program divides the content into different parts and each thread takes on its responsibility to read the string in one part. It imitates the behavior of reading separate parts of the file.
Concurrency: Here, multiple threads are created, each with a specific portion of the content string that reads it.
Output:
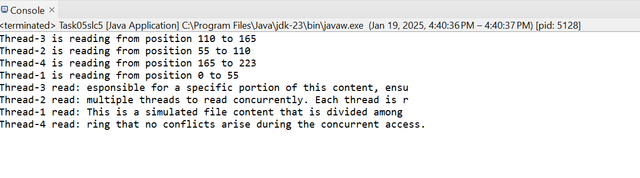
06
Develop a program simulating a bank system where multiple threads perform deposits and withdrawals on shared bank accounts. Use synchronization to ensure thread safety and prevent issues such as race conditions. Explain the techniques used to maintain data integrity in the presence of concurrent threads. |
---|
Here is a code that fulfills the question requirements:
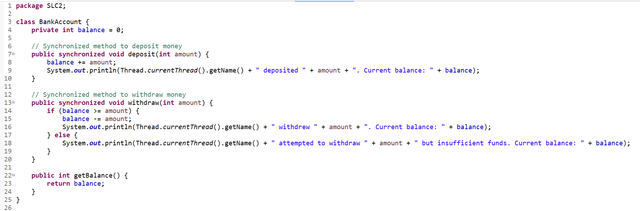
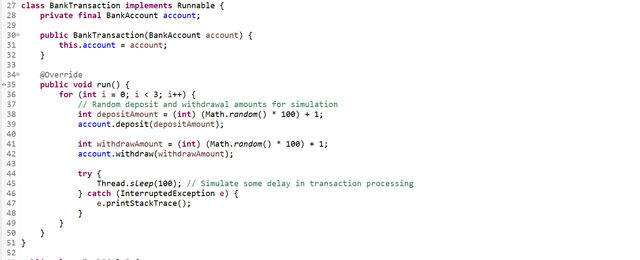
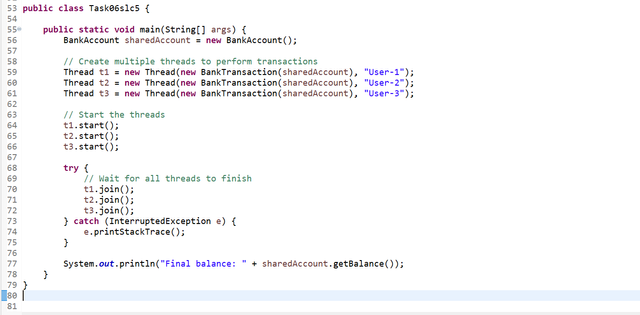
Explanation of Techniques for Thread Safety:
Synchronization: Both the deposit()
and withdrawal()
methods are designated as Synchronization. Their advantage is that they ensure that only one thread can execute its methods on any shared bank account object. The function of synchronization is to prevent race conditions when different threads try to modify the same shared data to read it.
Race Condition: Without synchronization, when one or more threads try to modify data, incorrect results are produced because, for example, if one thread is reading the balance of the data and has not updated it yet, while another thread is depositing or withdrawing from it, the data becomes corrupt.
Data Integrity: We use synchronization to maintain the integrity of our data. If one thread is making a deposit, the other thread will now have to wait until it finishes its work to access that account. This handles situations where one thread is trying to withdraw money while another has already deducted it from the account but has not yet completed the operation.
Thread Coordination: Thread.sleep(100)
simulates delays in between transactions so that it is easy to observe synchronization during them. Join()
ensures that the main thread waits for all subordinate threads to finish before printing the final balance.
Output:
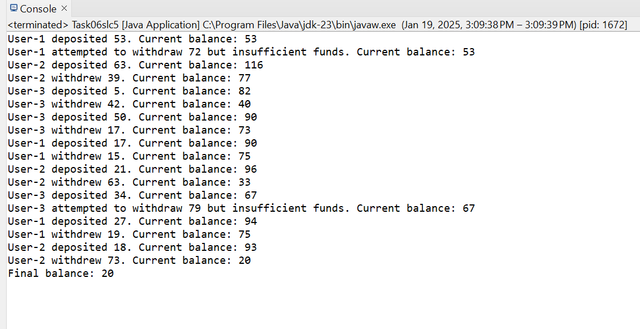
Thank you so much for staying here. I would like to invite @josepha, @ulfatulrahmah, and @suboohi to join this challenge.
Cc:
@kouba01