SLC S22 Week4 || Exception handling in Java
Hello everyone! I hope you will be good. Today I am here to participate in the contest of @kouba01 about Exception handling in Java. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
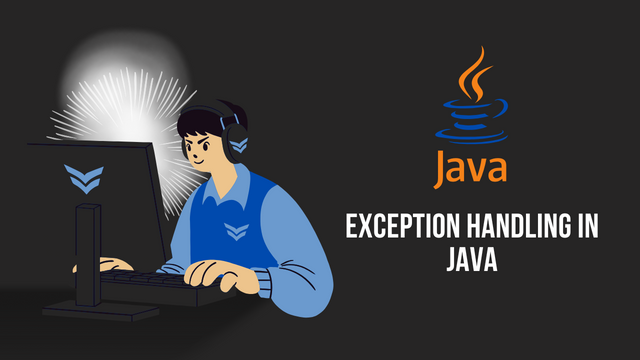
Task 1. Detecting Faulty Sensor Readings
We often encounter faulty sensor readings while interacting with the data processing. And it is very important to manage those exception values to keep our data and records neat and clean and with the desired values.
Here is a Java program that detects and handles faulty sensor readings. It will filter the faulty readings and then exclude them and continue to process the data which is valid. The program will use exception handling to manage invalid temperature readings.
Here is a detailed explanation of the code for Detecting Faulty Sensor Readings. I will explain how the program will work to detect the faulty sensor readings and will continue to process valid data.
The program processes a list of temperature readings from sensors. It identifies invalid readings which are negative values for above freezing sensors. Then it logs the errors and displays a meaningful message for the user as information about the error. After logging the error message it continues to process the valid data.
- This is an array. This array stores the values of the temperatures. These are collected from the sensors.
- In the array there are two types of values. One type is of the valid values such as
23
,18
and the other is of the invalid readings-5
,-10
for the temperature collected from the sensors.
- Here is another new list (
validReadings
). It is used to store the valid temperature readings for later use or display.
Error Handling with a try-catch
Block
In programming often to handle the errors try
and catch
blocks are used. Similarly in this program I have used try and catch block system. Actually the loop processes each reading and checks for validity and handles errors.
try
Block:- This block of code checks if the current reading is invalid such that
reading < 0
. - If the reading is invalid then it trows an
IllegalArgumentException
with a descriptive message such as"Temperature cannot be negative: -5"
.
- This block of code checks if the current reading is invalid such that
catch
Block:- This block of catches the exception and logs the detailed error message to
System.err
for administrators. - It prints a user friendly message to indicate the program is continuing without interruption such as
"Skipping invalid reading: -5"
.
- This block of catches the exception and logs the detailed error message to
After processing all readings now we need to output the valid readings. For this purpose the below code is used.
- It displays only valid readings to the user with the help of the list which we created to store the valid readings such as
[23, 18, 30, 25]
.
- After processing the given readings the program has successfully worked.
- We can see that the program has separate out the invalid readings by printing a message.
- Then after the filtration it has returned only valid values.
- In the provided data of readings the program detected -5 and -10 as invalid values.
Task 2. Dynamic Input Validation for an Event
Sometimes when we are dealing with the dynamic input validation then we can face problems in the input. If the user input wrong value then the system should response accordingly and inform the user to enter valid value for the input. But if we do not implement this check then our system is not capable to cope with the invalid inputs.
In the Java programming we handle these invalid dynamic input values by implementing check condition if the input meets our requirements or not. Here is the Java program which validates the dynamic input values of the age of the user between 18-60 years.
The program validates participant ages dynamically for an online registration form. It ensures that the age is within the range of 18 to 60. If the user enters an invalid age then the program:
- Throws a custom exception by using
InvalidAgeException
for out of range values. - It handles invalid inputs by displaying clear and meaningful error messages.
- This program allows the user to input age again without crashing the program. This functionality ensures smooth working of the program.
- This class extends
Exception
class of Java. It makes it a custom checked exception. - This class identify the invalid age inputs and then it provides detailed error message.
- We can also use
IllegalArgumentException
buty when we use custom exception class then it makes error handling more meaningful.
- The program reads the user input using
Scanner
. - It assumes input is an integer because the age should be an integer . If the user enters invalid input such as
abc
then the program handles it in the catch block.
This is the validation logic:
- If the age is less than 18 or greater than 60 then the program throws the custom exception by using
InvalidAgeException
with a detailed error message.
The program uses two catch
blocks to address different types of invalid inputs:
- This catch block catches the custom
InvalidAgeException
. - It displays a clear and user friendly error message such as
"Age must be between 18 and 60. You entered: 16"
in case if the user enters 16.
- This catch block catches all other exceptions such as entering non numeric values like "twenty".
- Moreover if the user does not enter the whole number such as 20.5 of the age then it again displays an error message.
- It provides meaningful feedback such as
"Invalid input: Please enter a valid number."
. - Uses
scanner.next()
to discard invalid input and prevent an infinite loop.
while (true) {
try {
// Input and validation logic
} catch (...) {
// Error handling
}
}
- This
while (true)
loop ensures that the program continuously prompts the user until valid input is provided by the user - Without using this loop the program would terminate after encountering an exception. And it will require the user to restart and run the program.
- If the user enters valid age within the given range then the program confirms successful registration and exits the loop using
break
statement.
- Here in the working of the program you can see that when I am entering alphabets instead of numbers then the program is displaying error.
- Similarly when I am entering value out of the range then it is throwing an error message.
- In the third case I entered value in the range and it displayed success message.
Task 3. Hierarchical Exception Handling in a Game
We often play games and in the games there are different roles and stages. And while playing the game we often click on the wrong attributes such as when we want to kill an enemy we need to use the weapon but instead of weapon we click on any other attribute like the direction then it will not work to kill the enemy. So we need to manage these exceptions. Here is the Java program where I have designed a simple game which handles the exceptions in the game hierarchically.
This code defines a simple RPG game where a player can perform actions such as moving in a direction, using an item and taking damage. The game demonstrates handling custom exceptions for different gameplay scenarios.
The program has the following different classes to handle the exceptions:
Base Class:
GameException
:- This is a custom exception class. It serves as the base class for all game related exceptions. All other custom exception classes extends this class.
- It extends
Exception
class of Java by allowing custom error messages.
Derived Exception Classes:
Here are three derived classes which are derived from base class GameException
:
HealthException
: This class handles errors related to player health such as health reaching zero. After the health reaches zero then this will handle it and will display the Game Over because of zero health.MoveException
: This derived class handles invalid moves such as a direction other than North, South, East, or West.InventoryException
: This derived class handles errors when an item not in the inventory is called for the usage in the game.
Variables:
health
:
- This is the health variable. It represents the current health of the player.
- Initially I have set it to
50
.
inventoryItem
:
- This variable is used to store the inventory item. This is single item inventory and initially it contains the string
"Sword"
.
- This variable is used to store the inventory item. This is single item inventory and initially it contains the string
Methods
There 3 different methods in the game to manage the functionality of the game. These are given below:
move(String direction)
:
- This method checks if the entered direction matches valid directions North, South, East, West.
- If the user enters invalid direction then it throws a
MoveException
to inform the user that the move is invalid. - It prints the movement direction if valid.
useItem(String item)
:
- Wen the user enters the item then it compares the entered item to the
inventoryItem
. And it is case insensitive. - It throws an
InventoryException
if the item does not match with the inventory. - It prints a message to confirm the item was used.
takeDamage(int damage)
:
- This method is used to manage the health of the player and keeps the record of the health. It reduces the
health
by the specifieddamage
. - If the damage is greater than or equal to the current health then all remaining health is used and a
HealthException
is thrown to indicate game over. - If the damage is less than the current health then the health is reduced and the current health is printed.
Main Method
The main method implements the game loop. It allows the player to perform actions and handle exceptions.
Game Loop:
- Runs in an infinite loop (
while (true)
) which allows repeated gameplay. - It breaks when the player chooses to end the game after a
HealthException
.
Gameplay Actions:
- Move:
- This prompts the user for a direction and validates it using
move()
method.
- This prompts the user for a direction and validates it using
- Use Item:
- This prompts the user for an item and validates it using
useItem()
method.
- This prompts the user for an item and validates it using
- Take Damage:
- This prompts the user to enter damage and applies it using
takeDamage()
method.
- This prompts the user to enter damage and applies it using
Exception Handling:
MoveException
andInventoryException
:- These functions print specific error messages for invalid moves or items.
HealthException
:
- It handles scenarios where health reaches zero.
- This prompts the player to reset the game or end it.
- This can also reset the game by reinitializing the
RPGGame
object if the player chooses to reset. - If the player chooses not to reset after a health exception then the loop exits and the game session ends.
At the end of the program the scanner.close() is used to avoid the resource leaks.
Task 4. Banking System Transaction Errors
Every banking system is very sensitive and there must be check and balances to ensure the safety and smooth transactions. We need to manage the exceptions in the transactions efficiently. Here is the Java program where the program will implement the error handling to filter out the exceptions in the transactions to ensure only correct transactions can be made.
This program simulates a banking system where transactions are processed and various exceptions are handled to ensure proper validation. It validates transactions by checking for these exceptions:
- Insufficient funds when attempting to withdraw more than the available balance.
- Invalid account numbers when trying to perform transactions between non existing accounts.
- Negative transfer amounts when the user enters a transfer amount less than or equal to zero.
The custom exception classes inherit from BankingException
and are used to handle specific types of errors:
BankingException
: The base class for all banking related exceptions.InsufficientFundsException
: Thrown when there are not enough funds to perform a withdrawal.InvalidAccountException
: Thrown when an invalid account is provided such as an account that does not exist.NegativeTransferException
: Thrown when the transfer amount is negative or zero.
These exceptions are then thrown in the appropriate places within the code to ensure that any transaction that violates the rules is flagged.
The BankAccount
class represents a bank account with methods to deposit and withdraw funds. These methods are given below:
deposit(double amount)
: This method adds the specified amount to the balance.withdraw(double amount)
: It subtracts the specified amount from the balance but checks if the account has sufficient funds. If the balance is insufficient then it throws anInsufficientFundsException
.
This is where the logic for the InsufficientFundsException is handled.
The BankingSystemLogic
class manages all the transactions betweenthe bank accounts. It contains logic to validate transfer requests. It uses these methods for the transfer:
addAccount(BankAccount account)
: It adds aBankAccount
to theaccounts
map with the account number as the key.transfer(String fromAccount, String toAccount, double amount)
:- First of all this method checks if the transfer amount is valid such as greater than zero. If it is not then it throws a NegativeTransferException.
- It checks if both the sender and receiver accounts exist. If either does not exist then it throws an InvalidAccountException.
- If both accounts are valid then the system proceeds with the withdrawal and deposit. If the sender has insufficient funds then an InsufficientFundsException is thrown.
- If everything is valid then it successfully transfers the funds and displays the updated balances.
In the main()
method the program interacts with the user and handles the entire flow of the transactions:
- User Input: The program prompts the user to input the sender account, receiver account and transfer amount.
Exception Handling:
- The try-catch
block ensures that any exception raised during the transfer such as insufficient funds, invalid accounts, or negative transfer amounts is caught and handled.
- If an exception occurs then an error message is displayed and the program logs the error.
This Banking System uses custom exceptions to handle different types of errors during money transfers. It checks for invalid accounts, negative amounts, and insufficient funds and ensures detailed feedback is provided to the user in case of failures. Successful transactions are processed and the remaining balances are displayed.
Task 5. Automating Resource Cleanup
Resource management is very important in each program and if we are unable to manage the resources in the programs correctly then memory leaks can occur and we can loose our data.
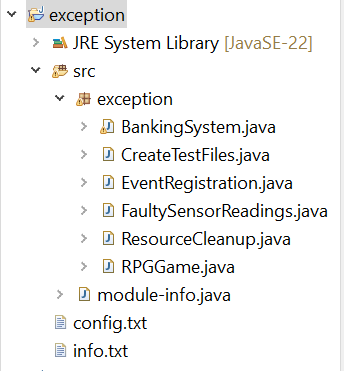
Before moving to the program here see I have created two files config.txt
and info.txt
in the same directory where the program is stored. S that I have access these files while running the program and test the functionality of the program. I added some dummy data in each file. Configuration file has its own data and similarly info.txt file.
Here is the Java code to demonstrate resource management and exception handling while reading files. The program reads multiple files and ensures they are closed properly even if an error occurs during the reading process.
The program imports and uses classes from the
java.io
package. It provides mechanisms to read from and write to files as well as handling file input and output (I/O) exceptions.In the main class the complete logic to handle the files is defined.
- Here I have defined an array to store the names of the files. These files will be used for processing the data. From this array the files will be read one by one.
- Here is a loop that iterates through each file name (
config.txt
,data.txt
, andinfo.txt
) in the filenames array. For each iteration it will try to open the file and read its contents.
- A
BufferedReader
is initialized to null at the start of each iteration. This reader will be used to read the contents of the file.
This is the try block which contains the code that attempts to open and read the file:
- A
BufferedReader
is created using theFileReader
class. It opens the specified file by using the name of the file. - The program prints a message to indicate the file being read.
- The while loop reads each line of the file and prints it to the console until there are no more lines left.
There are two catch blocks. The detail of those is given below:
FileNotFoundException
is caught if the file does not exist or cannot be opened for reading. The message"File not found: <filename>"
is printed.IOException
is caught for any general I/O issues that might occur while reading the file. This includes errors like permission issues or other I/O problems. A message"Error reading file: <filename>"
is printed.
The finally
block is always executed whether an exception occurred in the try
block or not. This is where the cleanup happens:
- If the
BufferedReader
reader is not null then it means the file was opened successfully. - The
reader.close()
method is called to close the file after reading. - If closing the file raises an
IOException
an error message is printed to indicate that closing the file failed. - If the file was closed successfully then a message
"Closed file: <filename>"
is printed. - If the file does not exist then it displays the relevant error that file does not exist.
On the whole the program reads the multiple files and for each file it checks if the file exists then reads the file and after reading it closes the file for the resources cleanup and then it opens the next file. The finally
block is executed always to close the file properly whether success or failure occurs.
Here you can see the output of the program. There three files in the list. Two files are available and one file is not available. It reads and display the data in the files which exist and shows error message for the file which does not exist.
Task 6. Predicting Future Errors Using Logs
Here is the Java program which implements a system that monitors user login attempts. It identifies suspicious activity and allows the administrators to view the login activity logs. The system is designed to handle incorrect login attempts and raise an exception when a user exceeds the allowed number of failed attempts. It provides a way for administrators to review all login attempts.
Here is the detail of this program:
- This is a custom exception that is thrown when suspicious activity is detected. It extends the built in
Exception
class. - When this exception is thrown it indicates that a user has attempted to log in incorrectly more than three times consecutively.
- There is a
UserLoginMonitor
class is the main handler of login attempts. It stores the failed login attempts. It also records login activity and allows administrators to view login logs. failedAttempts
: This is a map that stores the number of failed login attempts for each user. The key is the username and the value is the number of failed attempts in this map as it stores things in the key value pair.activityLogs
: This is a map that stores a list of login attempts for each user. The key is the username and the value is a list of strings (logs) representing login attempts.
The method processLogin
processes the login attempts for a given username and password. More details about this method are given below:
- If the password is incorrect then the number of failed attempts for the username is incremented.
- The system checks whether the user has exceeded 3 failed login attempts. If yes then a
SuspiciousActivityException
is thrown by indicating potential suspicious activity. - If the password is correct then the failed attempts counter for the username is reset to 0.
- The login attempt whether failed or successful is logged in the
activityLogs
map.
- This method is used by administrators to view all login activity logs.
- It iterates over the
activityLogs
map printing the username and all the login attempts including passwords used for each user.
This is the main method that runs the login system interactively More details about this function are given below:
- The user is prompted to enter their username. If the user types 'exit' the program ends.
- If the username is "admin" the administrator can view the login activity logs without needing to enter a password.
- For any other username the program prompts the user to enter a password then processes the login attempt using the
processLogin()
method. - If suspicious activity is detected after more than 3 failed login attempts then the program throws a
SuspiciousActivityException
which is caught and an alert message is shown.
Here you can see the working of the program. The program prompts to enter the username and after accepting the username it demands for the password. If the user enters wrong password more than 3 times then a message is displayed showing suspicious activity.
If the username and the password is correct then the program allows successful login. Moreover if we type admin
as the username then we can fetch all the log in details along with the username and the passwords entered by the username.
Hi, @mohammadfaisal,
Your post has been manually curated!