SLC S22 Week2 || The Object Approach In JAVA
Assalamualaikum my fellows I hope you will be fine by the grace of Allah. Today I am going to participate in the steemit learning challenge season 22 week 2 by @kouba01 under the umbrella of steemit team. It is about The Object Approach In JAVA. Let us start exploring this week's teaching course.
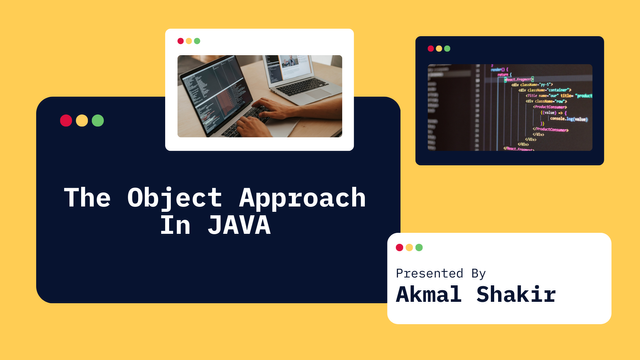.png)
Which of the following statements about Java classes and objects is true?
Here are the correct answers for each question:
1. What is a class in Java?
(A) A blueprint for creating objects.
Explanation: A class is a template or blueprint that defines the structure and behavior of objects created from it.
2. What does the new
keyword do in Java?
(B) Creates a new object of a class.
Explanation: The new
keyword is used to create a new instance of a class, allocating memory for the object.
3. Which of the following statements is true about attributes in a class?
(C) Attributes store data for an object.
Explanation: Attributes (fields) are used to store data or properties specific to an object.
4. What is the purpose of a constructor in a class?
(B) To initialize the fields of a class. A constructor is a special method used to initialize an object's fields when it is created.
Write a program that demonstrates the creation and use of a class by including attributes, methods, and object instantiation.
Here is the Java program which demonstrates the creation and use of the class by including attributes, methods, and object instantiation:
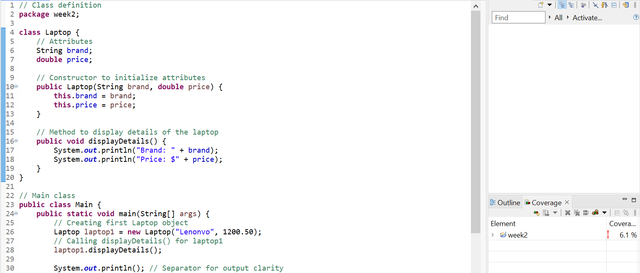
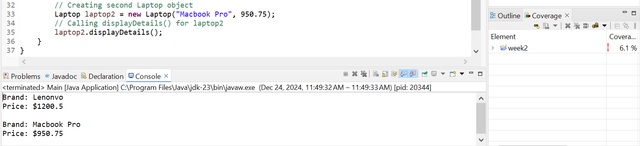
Here is an explanation of the program with functionality:
1. Defining the Laptop
Class:
The program begins by defining a Laptop
class that encapsulates the essential properties of a laptop. This class has two attributes: brand
(a String
) and price
(a double
). These attributes are used to store information about the laptop's brand and price.

2. Constructor for Initialization:
The Laptop
class has a constructor to initialize its attributes. A constructor is a special method that gets called when a new object is being created. Here, this constructor takes two parameters called brand
and price
and assigns them to the class's fields. This would ensure that every Laptop
object is always initialized with particular values.

3. Method to Display Laptop Details:
The Laptop
class also declares a method, displayDetails()
, to print out the details of a laptop in an easy-to-read format. The method calls print out the brand and price of a laptop. It defines this function within the class, ensuring encapsulation and easier reuse in a program.

4. Creating and Using Objects
The program, in the Main
class, demonstrates how to instantiate and use objects of the Laptop
class. The two objects of the Laptop
are instantiated by the use of new
, and their constructor is invoked with the creation of their brand
and price
attributes as being initialized with specified values. Each object calls the displayDetails()
method to display its details.
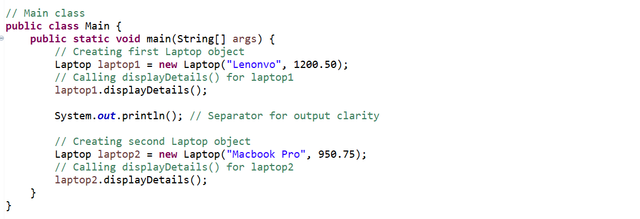
5. Separation and Output:
A blank line is printed between the details of two laptops for better readability of output. This allows distinguishing information to be shown for each object clearly.

After executing the program it shows the output with the detail of the laptops and their brand name and price.
This program shows how object oriented programming principles such as encapsulation and modularity are applied to create and manage real world entities like laptops.
Write a program that demonstrates the creation of a Movie class, its attributes, and methods, along with managing multiple objects in an array.
Here is the Java program that demonstrates the creation of a Movie class, its attributes, and methods, along with managing multiple objects in an array:
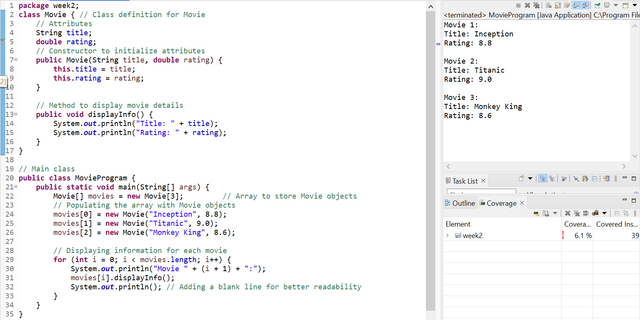
Here is an explanation of the program with functionality:
1. Defining the Movie
Class:
The Movie
class defines the structure and behaviour of a movie object. It includes two attributes:
title
(aString
): Represents the movie's title.rating
(adouble
): Represents the movie's rating.
The constructor initializes these attributes when an object is created, and the displayInfo()
method is used to print the details of a movie.
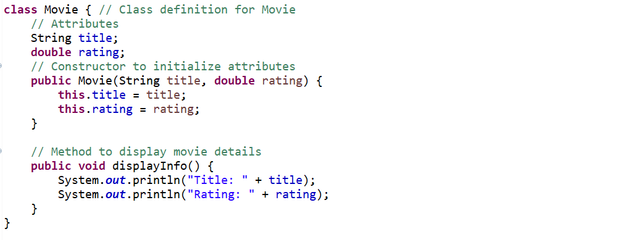
2. Array of Movie Objects:
In the Main
class, an array of type Movie
is created to store three Movie
objects. Arrays allow managing multiple objects of the same type efficiently.

3. Populating the Array:
The array is populated by creating three Movie
objects using the new
keyword and the constructor. Each object is initialized with specific values for title
and rating
. These objects are assigned to individual elements of the array.

4. Displaying Movie Details:
A for
loop iterates through the array, and for each movie object, the displayInfo()
method is called to print its details. To enhance readability, a blank line is added between the outputs of different movies.

This explanation] shows how the program defines a Movie
class, creates multiple objects, stores them in an array, and uses methods to display their information. This modular and structured approach demonstrates the principles of object oriented programming effectively.
Write a program that demonstrates adding methods with calculations by creating a Product class.
Here is the Java program that demonstrates adding methods with calculations by creating a product class.
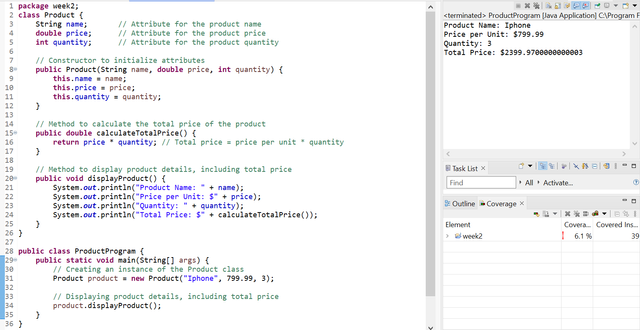
The program illustrates the definition and application of a Product
class, encapsulating attributes, methods, and calculations. It demonstrates how to organize a Java program with object-oriented principles to efficiently handle and process information regarding products.
1. Defining the Product Class
The Product
class is defined to model a product with three essential attributes:
name
: Holds the name of the product such as "Iphone".price
: This is the price for a single unit of the product for example799.99
.quantity
: This is the number of units purchased or available for example3
.
The class makes use of a constructor to initialize these attributes each time a Product
object is created. Therefore every product has its name
, price
, and quantity
defined at the point of its creation.

The this
keyword distinguishes instance variables from method parameters when they share the same name.
2. Calculating the Total Price
The class includes a method calculateTotalPrice()
to compute the total price by multiplying the product's price
by its quantity
. This method encapsulates the calculation logic, making the code modular and reusable.
For example if the product price is $799.99
and quantity is 3
the total amount will be $2399.97
.

This method enables easy modification or extension of the calculation logic in future without affecting any other parts of the program.
3. Displaying Product Details
The displayProduct()
method prints out the product's attributes (name
, price
, and quantity
) and also prints out the total price, which it obtains by calling calculateTotalPrice()
.
This method ensures all relevant product information is printed out in one coherent operation. The use of clear and descriptive System.out.println
statements improves readability.

Encapsulating the display logic within the class improves the separation of concerns and promotes code reusability.
4. Managing Products in the Main Method
The main
method demonstrates how to create and use objects of the Product
class. In this case:
- A
Product
object calledproduct
is created, representing an Iphone priced at$799.99
with a quantity of3
. - The
displayProduct()
method is called on the object to print its information and calculated total price.

The above code instantiates a Product
object in one statement thus ensuring all attributes are defined with useful values.
This program shows how to create a class with attributes and methods that include calculations. It encapsulates logic within methods and organizes code to promote reusability and clarity.
Write a program that manages student records by creating a Student class
Here is the Java program that manages student records by creating a Student class.
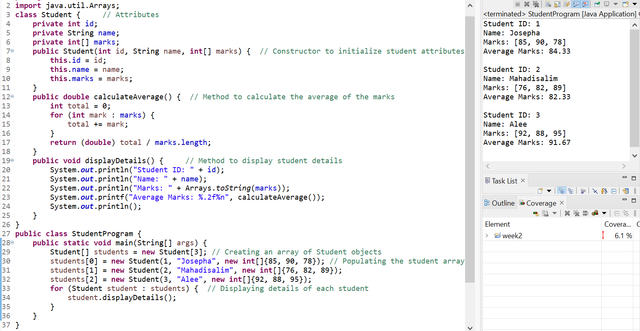
This program shows how to work with student records and manage them using object-oriented principles in Java. It demonstrates how to create a Student
class with attributes, methods, and handling multiple Student
objects stored in an array. Below is a step-by-step breakdown of the program with detailed explanations and code references.
1. Class Design: The Student Class
The Student
class contains all the data and behavior that characterizes a student. It contains:
Attributes
id
: An integer to uniquely identify the student.name
: A string to represent the student's name.marks
: An array of integers containing the marks that the student obtained in various subjects.
Constructor
A constructor initializes the Student
object by assigning values to the properties when the object is instantiated.

The constructor will ensure that at the moment of creation each Student
object possesses valid and relevant data.
2. Method: Average Calculation
The calculateAverage()
function calculates the average of marks stored in the marks
array. It will use a for
loop to iterate over the array, compute the total, and then divide it by the number of elements.
Logic
- Initialize variable
total
for holding sum of marks. - Iterate over
marks
array byfor
loop, where each mark will be added to thetotal
. - Average should be calculated by dividing total by the size of an array.

- It guarantees type safety by directly casting the returned value of
calculateAverage()
intodouble
to ensure computation of average marks. - It can handle arrays of any dimension and hence is reusable in varying situations.
3. Method: Prints Student Details
The displayDetails()
method prints the information of the student, such as Student ID, Name, marks, and average marks calculated by calling the calculateAverage()
method.

Formatted Output
- The
Arrays.toString()
method converts themarks
array into a readable string format. - The
printf
function formats the average to two decimal places, ensuring a professional and clean output.
4. Main Method: Managing Student Objects
In the main
method, we show how to use the Student
class by creating and managing multiple objects.
Steps:
- Create an Array:
An array ofStudent
objects is created to manage records for multiple students. The array size is set to 3 to accommodate three students.

- Fill the Array:
Every element in the array is assigned a newStudent
object with an ID, name, and marks.

- Print Student Information:
Afor-each
loop traverses the array and invokes thedisplayDetails()
method for each student to print their details.

This program simulates a simple student management system that can be extended to include more functionalities such as searching for students, updating marks, or sorting by averages. It is a good foundation for understanding how to manage collections of objects in real-life applications.
Write a program to simulate a simple Library Management System.
The program is designed to simulate a basic Library Management System where users can interact with a collection of books. It consists of three main classes: Book
, Library
, and LibraryManagementSystem
. These classes work together to handle the core functions of the system such as adding books, searching for books, borrowing books, and returning them.
1. Book Class
The Book
class is a simple representation of a book in the library. It encapsulates all the data about each of the books in the form of its ID, title, author, and availability. It also provides methods to interface with these data.
Attributes:

id
: This is a unique identifier for each book. It helps differentiate one book from another in the system.title
: This refers to the book's title. (Example: "Animal Farm").author
: This stores the book author's name. An example: "George Orwell".isAvailable
: Boolean value which will indicate a particular book is available to lend or not. Usually by default, books become available to borrow at the point of their creation.
Constructor:

The constructor initializes a new Book
object with the provided id
, title
, and author
. It also sets isAvailable
to true
by default, meaning the book is initially available for borrowing.
Methods:
- borrowBook():
This method checks if the book is available (isAvailable
istrue
). If it is available, it marks the book as borrowed by settingisAvailable
tofalse
. If the book is not available, it informs the user that the book is currently unavailable.

- returnBook():
This method marks the book as available again when it is returned. It changes theisAvailable
flag back totrue
.

- displayDetails():
This method displays the details of the book, including its ID, title, author, and whether it is available for borrowing.

- Getter methods:
These methods allow theLibrary
class (and any other class) to access the book ID, title, and availability status.
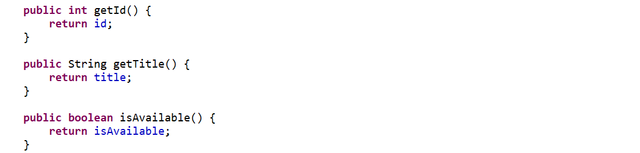
2. Library Class
The Library
class is responsible for managing a collection of books. It stores all the books in an ArrayList
. It also provides methods for adding books, displaying all books, searching for books, and allowing users to borrow or return books.
Attributes:
books
: This is anArrayList
that contains all the books in the library. It gives dynamic storage for any number of books that are added to the library.
Constructor:

The constructor initializes an empty ArrayList
of books which will later hold the books that are added to the library.
Methods:
- addBook(Book book):
This approach adds a book to thebooks
list and prints out a confirmation message that states that a book has indeed been added to the library.

- displayAllBooks()
This will go through all books in thebooks
list, callingdisplayDetails()
on each one to print out details for each book in the library.

- searchByTitle(String title):
This method searches for a book by its title. If a match is found then it displays the details of the book. If no match is found then it prints a message. The message indicates that the book was not found.
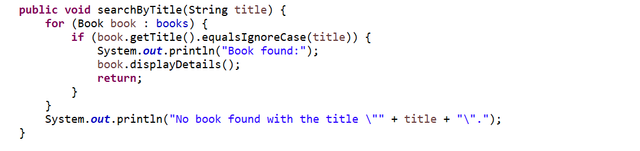
- borrowBook(int id):
This method allows the user to borrow a book by its ID. If the book with the given ID is found then it calls theborrowBook()
method of theBook
class. If the book is not found then it prints a message. The message says that no book was found with the given ID.
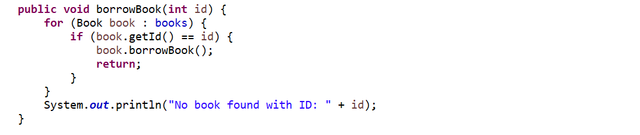
- returnBook(int id):
This method allows the user to return a borrowed book by its ID. If the book with the given ID is found then it calls thereturnBook()
method of theBook
class. If the book is not found then it prints a message. The message indicates that no book with that ID exists in the library.
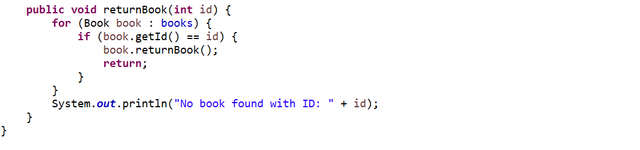
3. LibraryManagementSystem (Main Class)
This is the main class that powers the program. It represents the entry point to the Library Management System. And from this the user interacts with the system via a menu of options. This class contains the main
method. It initializes the library, adds some books and then display a menu. It allows the user to interact with the system.
Main Menu Loop:
The main
method shows a menu of choices in a loop and lets the user perform several actions. The menu provides five choices:
- View all books
- Search for a book by title
- Borrow a book by ID
- Return a book by ID
- Exit the program
This is how the user interaction is structured:
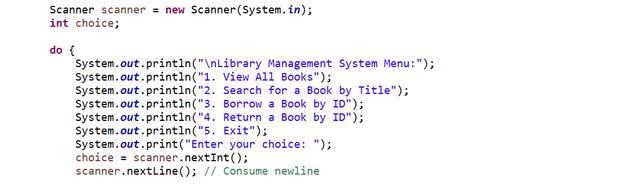
- View All Books: This will call the
displayAllBooks()
method of theLibrary
class that prints all the books present in the library.

- Search a Book for its Title: The following code prompts the user to enter a title and asks
searchByTitle()
to find any books with that title and print them.

- Borrow a Book by ID: Prompts the user for a book ID and calls
borrowBook()
to borrow the book if available.

- Return a Book by ID: It asks the user to input a book ID and calls
returnBook()
to return the borrowed book.

- Exit: Exits the loop and ends the program.

This simple system demonstrates key object-oriented programming concepts such as encapsulation, object manipulation, and method calls, which make it an effective foundation for more complex systems like a real-world library management system.
I invite @wilmer1988, @josepha, @wuddi to join this learning challenge.
Upvoted! Thank you for supporting witness @jswit.