SLC S22 Week2 || The Object Approach In JAVA
Hello Everyone
I'm AhsanSharif From Pakistan
Greetings to you all, hope you all are well and enjoying a happy moment of life with steem. I'm also good Alhamdulillah. |
---|
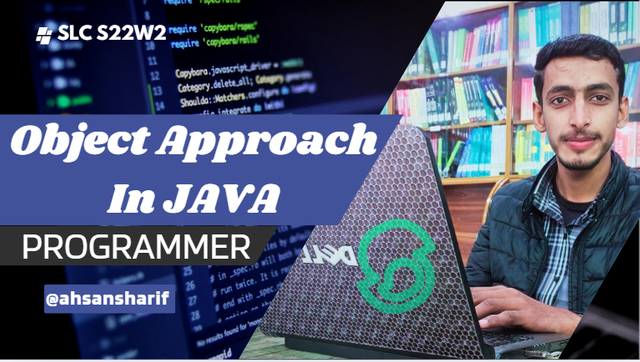
Design On Canva
Task 01
Which of the following statements about Java classes and objects is true?
- What is a class in Java?
- (A) A blueprint for creating objects.
Reason: These classes serve as a template for creating objects in Java. They define the structure and behavior.
- What does the
new
keyword do in Java?
- (B) Creates a new object of a class.
Reason: We use the word new to instantiate any class with another object.
- Which of the following statements is true about attributes in a class?
- (C) Attributes store data for an object.
Reason: The function of attributes is to store specific data for all objects created from the class.
- What is the purpose of a constructor in a class?
- (B) To initialize the fields of a class.
Reason: Constructors The fields of objects are initialized when any instance of the class is created.
Task2
Write a program that demonstrates the creation and use of a class by including attributes, methods, and object instantiation. Specifically, create a
Laptop
class with attributesbrand
(a string) andprice
(a double).Implement a constructor to initialize these attributes and a method
displayDetails()
to print the brand and price of the laptop.In the
main
method, create two instances of theLaptop
class with different values for the attributes and call thedisplayDetails()
method for each object to produce and display the corresponding output.
Considering Task Two, its current coding is as follows:
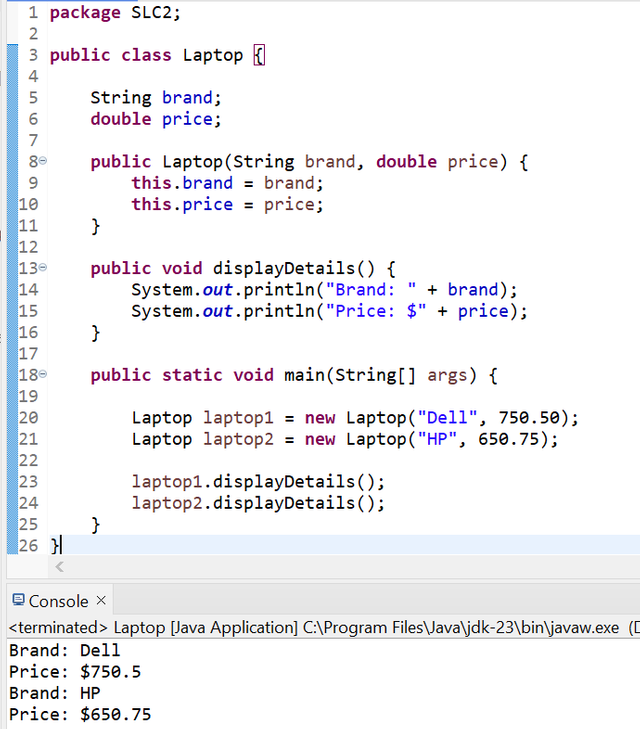
Explanation:
Attributes & Constructor:

This Laptop class has two types of attributes, one is Brand and the other is Price. Brand is a string and Price is a double. Both of these represent the properties of the laptop. In addition, a constructor Laptop contains the string Brand and the double Price. Both of these define what is used to initialize these pages when creating new objects.
Display Method:

In this, we use the displayDetais()
method to print our brand or our price. This allows us to see all the details of our laptop.
Main Method:
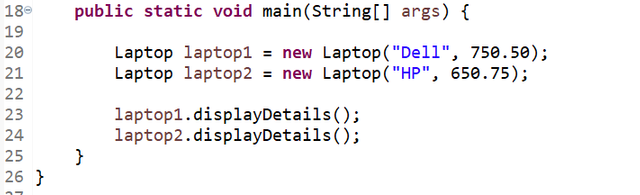
In the main method, we will create two objects using the New
keyword, one will be Laptop One and the other will be Laptop Two. Each will be used with a different brand and different price values.
Similarly, we will print both objects using the DisplayDetails()
method, which will show specific details.
Task3
Write a program that demonstrates the creation of a
Movie
class, its attributes, and methods, along with managing multiple objects in an array. Define theMovie
class with the attributestitle
(a string) andrating
(a double).Implement a constructor to initialize these attributes and a method
displayInfo()
to print the title and rating of the movie.In the
main
method, create an array to store threeMovie
objects, populate the array with details for each movie, and call thedisplayInfo()
method for each object to display their respective details.
Considering Task Three, its current coding is as follows:
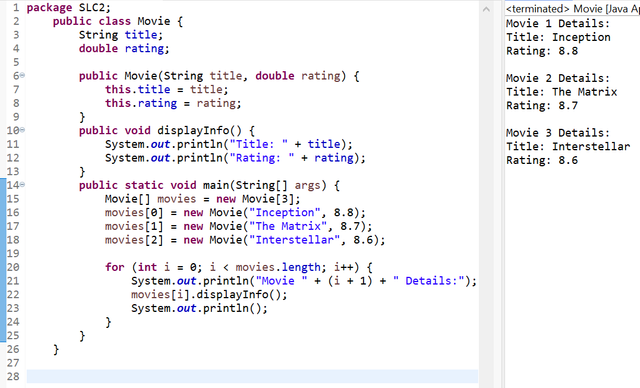
Explanation:
Movie Class:

There are two types of attributes in the Movie class, one is Title which represents the name as a string and the other is Rating which will represent the movie rating as a double apart from that in the constructor we have the movie string Title and Double Rating values used to create the object.
Display Method:

In the Movie class, we have to use the DisplayInfo()
method to print the title and rating of the movie.
Main Method:
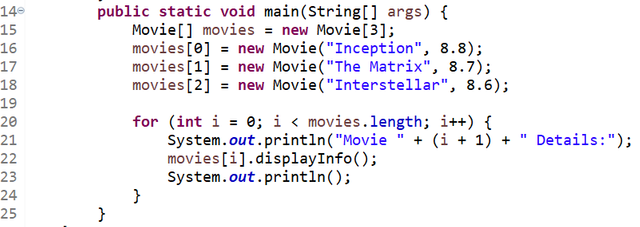
This is an array Movie[ ] movies = new Movie [3];
created to hold the object's movie.
Similarly, using the constructor, our array is filled with three movie objects and they are movies[0] = new Movie("Inception", 8.8);
, movies[1] = new Movie("The Matrix", 8.7);
, and movies[2] = new Movie("Interstellar", 8.6);
.
We use a for loop to iterate the array and then we call DisplayInfo()
which will print our movie objects including the title and rating etc.
Task4
Write a program that demonstrates adding methods with calculations by creating a
Product
class. The class should include the attributesname
(a string),price
(a double), andquantity
(an integer).Implement a constructor to initialize these attributes and a method
calculateTotalPrice()
to compute the total price as the product ofprice
andquantity
. Additionally, create a methoddisplayProduct()
to print the product's details, including the total price.In the
main
method, create an instance of theProduct
class, and call thedisplayProduct()
method to output the product's details and its total price.
Considering Task Four, its current coding is as follows:
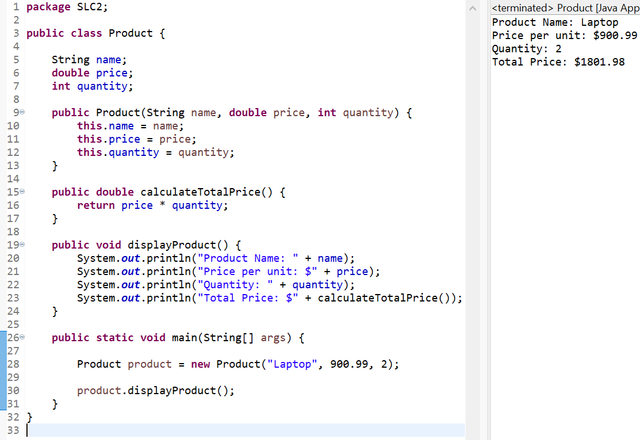
Explanation:
Product Class:

There are a total of three attributes in the Product class: Name, which will represent the name of our product, Price, which will show the unit price of the product, and Quantity, which will show how many units of the product have been purchased.
A constructor is defined to initialize all of these attributes Product(String name, double price, int quantity)
when any new product object is created.
Calculation & Display:
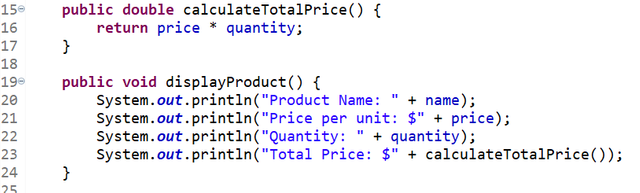
In our program, this method calculateTotalPrice()
calculates the total price by multiplying the number of products and their price.
The displayProduct()
method prints the details of our product, including the name, price, and quantity, using the CalculateTotalPrice()
method.
Main Method:

In this main method, a constructor is used to create an instance of the product class, in which the product name, price, and quantity are set.
After creating the class, we will then display the price and quantity of these products using the displayProduct()
method.
Task5
Write a program that manages student records by creating a
Student
class. The class should have the attributesid
(an integer),name
(a string), andmarks
(an array of integers).Implement a constructor to initialize these attributes and a method
calculateAverage()
to compute and return the average of the marks. Additionally, create a methoddisplayDetails()
to print the student's details, including their average marks.In the
main
method, create an array of threeStudent
objects, populate the array with details for each student, and call thedisplayDetails()
method for each object to display their respective information, including their average marks.
Considering Task Five, its current coding is as follows:
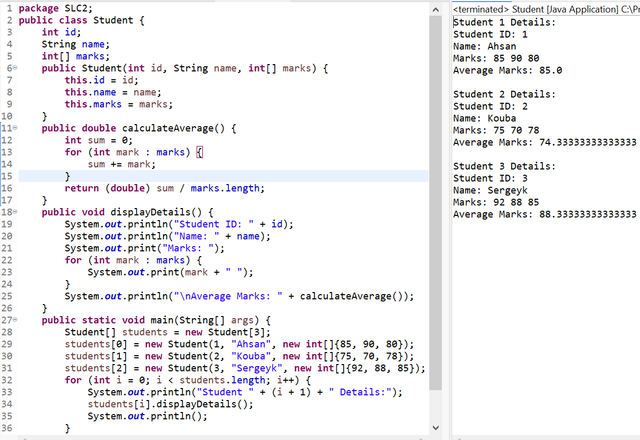
Explanation:
Student Class:

The Student class also has three attributes. The first is ID, which will represent the ID of our students, the second is Name, which will store the student's name, and the third is Marks, which will store the student's marks, in different subjects.
Now we use the constructor to initialize our attributes Student(int id, String name, int[ ] marks) whenever the Student object is created.
Calculate Average & Display:
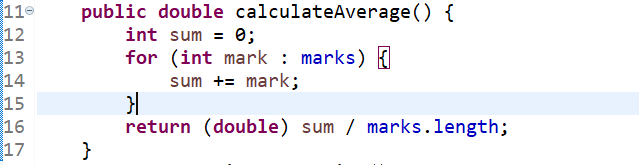
This method calculateAverage()
will find the average of our students' marks. First, it will sum them all. After summing, it will divide them. It will then divide by as many numbers as there are marks in an array.
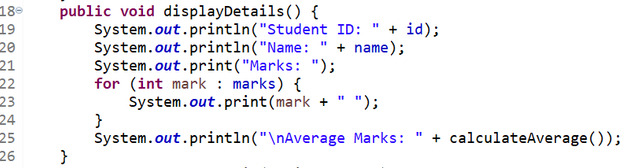
After calculating their average with the help of CalculateAverage()
, the DisplayDetails()
method is being used, which will display the ID name, marks, and average marks of our students.
Main Method:
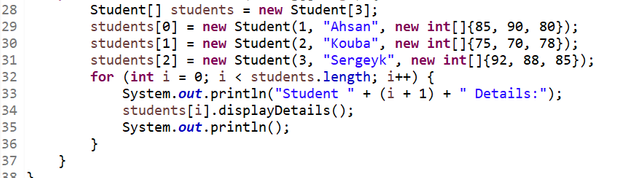
Using the main method, a student array will be created in which the data of three students will be stored. Each element in an array will be assigned a new student with a different ID, name, and marks.
This way, our program will be included in the loop and to display the student from each array, we will call displayDetails()
and it will print our data.
Task6
Write a program to simulate a simple Library Management System. The program should include the following:
Book Class
- Attributes:
id
(int): A unique identifier for each book.title
(String): The book’s title.author
(String): The author of the book.isAvailable
(boolean): Indicates whether the book is available or borrowed.
- Methods:
- A constructor to initialize all attributes.
borrowBook()
: SetsisAvailable
tofalse
if the book is available, otherwise prints that the book is not available.returnBook()
: SetsisAvailable
totrue
.displayDetails()
: Prints the book's details, including its availability.
- Attributes:
Library Class
- Attributes:
books
(ArrayList< Book >): A collection of books in the library.
- Methods:
addBook(Book book)
: Adds a new book to the library.displayAllBooks()
: Displays the details of all books in the library.searchByTitle(String title)
: Searches for a book by its title and displays its details if found.borrowBook(int id)
: Allows a user to borrow a book by its ID if available.returnBook(int id)
: Allows a user to return a book by its ID.
- Attributes:
Main Method
- Create a library with a few initial books.
- Allow the user to perform the following actions:
- View all books.
- Search for a book by title.
- Borrow a book by ID.
- Return a book by ID.
Book.java
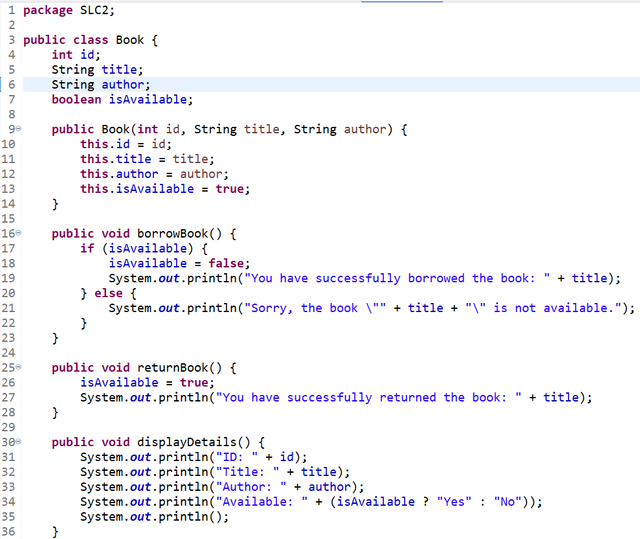
Book.java:
The Book class includes our ID, Title, Author, and IsAvailable attributes. In it, we have the method that defines how we can borrow and return books, and display the details of our books.
Library.java:
The purpose of the Library class is that it manages our book objects, which provides us with methods by which we can add a book to the library, display it, search it by its title, we can borrow it by its ID, or return the book by its ID.
LibraryManagementSystem.java:
This is the main class from where our program runs. It creates this library instance for us and provides a user interface through which it allows the user to view all the books in this library search for them by their title borrow this book by ID or return that book from there through ID.
It has a while loop that will continuously display our menu to the user until they select exit.
Code
Output
Thank you so much guys for staying here. I would like to invite @josepha, @abdullahw2, and @rumaisha to join this challenge.
Cc:
@kouba01
Presentasi anda sangat bagus dan tertrukstur.
Semoga anda sukses dan beruntung.
Thank you so much dear