SLC S22 Week1 || Getting Started with Java and Eclipse
Assalamualaikum my fellows I hope you will be fine by the grace of Allah. Today I am going to participate in the steemit learning challenge season 22 week 1 by @kouba01 under the umbrella of steemit team. It is about Getting Started with Java and Eclipse. Let us start exploring this week's teaching course.
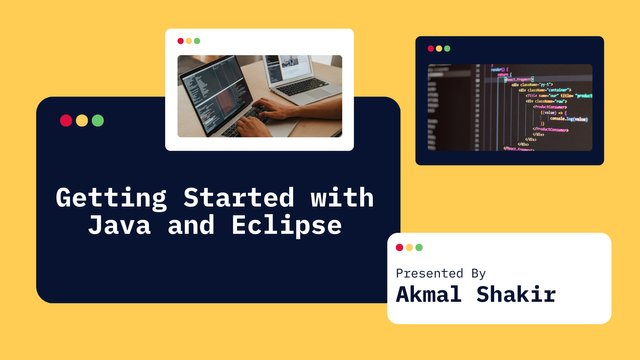.png)
Write a detailed explanation of the differences between JDK, JRE, and JVM by including their roles, functionalities, and interdependence.
The architecture of Java is made up of three main components: JDK (Java Development Kit), JRE (Java Runtime Environment) and JVM (Java Virtual Machine). Each serves a different purpose, yet they are interdependent, and they work together in order to develop and run Java applications.
Java Development Kit (JDK)
The JDK is the tool used by developers to write, compile, and debug Java programs. It acts as a comprehensive development environment that contains everything necessary for developing Java applications. Key components of the JDK include a compiler for converting Java source code into bytecode, tools for debugging and profiling, and additional utilities for packaging applications. Importantly, the JDK also includes the JRE, which enables developers to test and execute their applications. Without the JDK, creating and building Java applications would not be possible.
Java Runtime Environment (JRE)
The JRE is designed to provide an environment in which Java applications can run. It includes the JVM, core libraries, and native code that interfaces with the underlying operating system. The JRE guarantees that all dependencies like Java class libraries and APIs are available for the proper functioning of applications. Although it enables the execution of Java applications, it does not include tools like the compiler, which are needed for development. This makes the JRE suitable for end-users who simply need to run Java applications but are not involved in their creation.
Java Virtual Machine (JVM)
The JVM is the center of Java's "write once, run anywhere" promise. It is a virtual machine responsible for executing the Java bytecode, which is the platform-independent code generated by the JDK's compiler.
The JVM consists of several key components: the class loader, which loads classes into memory; the bytecode verifier, which checks for compliance with Java's security and language rules; and the execution engine, which translates bytecode into machine-specific instructions using either an interpreter or a Just-In-Time (JIT) compiler. The JVM offers an abstraction layer between the Java program and the hardware, so that the same bytecode can run on any system that is equipped with a compatible JVM.
Interdependence of JDK, JRE, and JVM
These components work together seamlessly to enable both the development and execution of Java applications. The JVM executes Java bytecode, while the JRE provides the libraries and environment needed for the JVM to operate. The JDK, in turn, includes the JRE and adds tools for developers, making it the most comprehensive package.
JVM is the runtime executor, JRE is the execution-support environment, and JDK is the toolkit for writing and running Java programs. It ensures flexibility and efficiency by providing a modular structure for the adoption of Java programs on any platform.
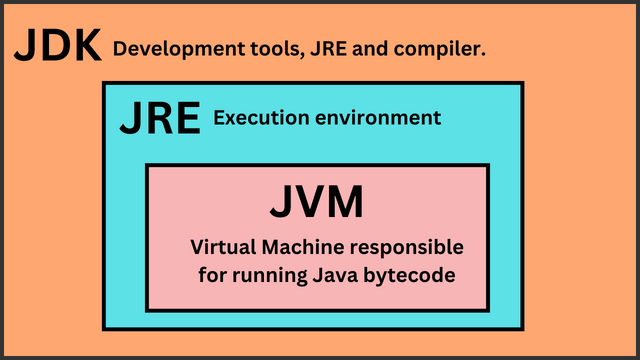
This is the graphical illustration of the JDK, JRE, and JVM where the JDK is the parent and it is holding other components as child in it to complete the development environment.
Install and Set Up Your Java Development Environment and provide step-by-step screenshots of the installation process, Eclipse configuration.
Let us start setting up the java development environment.
JDK Download
- We will go to the Oracle's official website.
The main page looks like as shown above in the picture.
- Then I will move to download the compatible version of the JDK for my system. I am using a Windows system so I have chosen windows JDK installer to download from the given options.
JDK Installation
- After the complete downloading I have double click the setup to install it.
- I clicked the next button and a new interface was there to choose the destination file. I did not touch the destination for the installation because it was already in the C file. So I continued by the default destination.
- After setting up the destination I clicked on the next button. It took a few seconds for the progress and it was completed the installation of JDK.
As I am a window user now I have to configure the environment variables for the JDK because if I do not set its environment variables I cannot access it in the CMD. So it is necessary task.
- I opened the My Computer and then I did right click of the mouse and It gave me some options I selected properties. It opened a new window as you can see below.
- From this I selected Advanced system settings. It opened the settings to to add or remove the environment variables.
- From this window I selected Environment Variables to add it.
- Here to add new environment variable we need to choose New....
- Here we need to add the name and the value of the variable. The value of the variable is actually the path of the JDK file.
I have copied and pasted the path of the JDK directory. If we give wrong path then it will not work. Then I have added it to the environment.
- The variable is added now and we can access it in the CMD to check for its verification.
- Now to verify the installation I have run the command java -version to check the version information if the cmd is able to access the information or not. But it was successful as it gave the required information.
Eclipse Download
- We will go to download Eclipse IDE.
- I have selected Download 2024 - 12 for the downloading. It has redirected me to another page which looks like this.
- After the completion of the download setup I have opened it to install it.
- Now we need to choose the IDE for the Java Development as we are going to interact with java.
- Now we need to add the JVM path and the installation folder path. And after that we need to click on install and the IDE will be installed.
- We need to give the correct JVM path otherwise the installation will not be carried out but initially it detects the path by itself.
- Before the installation they require to accept their user agreement.
- After this the installation took sometime by downloading and installing the required tools and packages for the complete development environment.
- Now we have to set the workspace folder where our work will be saved.
- After selecting this it will start the IDE.
- This is the first look of Eclipse IDE.
Write a program that calculates and displays the sum of the first 100 integers. Define the main method in a class named Sum to handle the entire calculation.
First Program
This is the simple addition program to add the first 100 integers. The entire calculation is handled in the main method.
A
sum
variable to store the sum of the numbers.A loop to find out the sum of the first 100 integers.
sum = sum + i
is storing the and updating sum value with each iteration of the loop.
Second Program
A separate function
calculateSum
to perform all the calculations is written in this program with the same working as in the previous program. I have just put it in the separate function.Then I have called this function in the main method and it is returning the same output as in the previous program.
Translate, Complete, and Debug the Following Program Named Tax.java
Tax.java
I have created a method to calculate the tax with the name
calculateTax
and I have passedincome
as a parameter to this method.I have defined the
tax
variable asdouble
because it can be a floating value. So for the precision it is in the double type.Then there are conditional statements according to the given conditions in the question. Each condition is validating and checking the specific limit of the income and implementing tax to that specific amount of limit.
In the main method I have passed
income = 57000;
to calculate tax for this amount of income.After giving this amount of income the program has calculated the correct amount of tax as expected which is 5550.
Conversion of character
Here is the java program which can convert the characters from the lowercase to uppercase and similarly uppercase to lowercase and it also checks if the given character is a letter or not.
I have created a class
Conversion
and there is aconvert Character
function in which there are the rules to convert the uppercase letter to the lowercase and similarly for the lowercase to uppercase.Character.isLowerCase(c)
checks if a given character is a lowercase or not.Character.isUpperCase(c)
checks if a given character is a uppercase or not.If the given chracter is neither an uppercase nor a lowercase then it returns not a letter.
If we check the output of the program we can observe that it has converted lowercase d to uppercase D and uppercase Q to lowercase q. And when there is a number then it has printed the message it is not a letter.
Array Operations
Here is a complete java program which will perform all the required operations as asked in the question such as finding the largest number of the array, sum of all the numbers of the array and the sorted array.
I have created a class
ArrayOperations
. In the class there are four functions to find out the largest element, sum and sorted array and the main function to handle the program.The first function is checking all the numbers in the array and it is returning the largest number.
The second function is traversing the array and it is finding the sum of all the numbers in the array.
The third function is taking the array and it is using the built in method to sort the arrays. It is sorting the array to the ascending order.
This is the output of the program. I have double tested the program by giving the array filled with numbers and one array without numbers to check the behaviour of the program. The largest element in the array is 123 which is correct and the sum of the array is also 364 which this program has returned correctly. At the end if we see the order of the array it is also in the ascending order.
I invite @wilmer1988, @josepha, @wuddi to join this learning challenge.