SLC S21 Week3 || Mastering Records and Record Arrays with Python
Hello everyone! I hope you will be good. Today I am here to participate in the contest of @kouba01 about Mastering Records and Record Arrays with Python. It is really an interesting and knowledgeable contest. There is a lot to explore. If you want to join then:
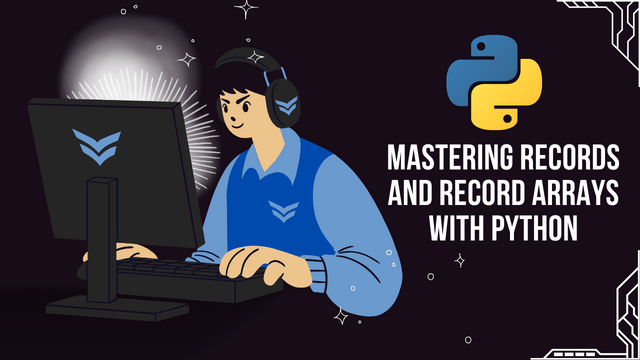.png)
Advanced Employee Records Management
Develop a Python program to manage employee records using dataclass. The program should support adding, updating, and displaying employee information, along with calculating average performance scores and identifying top performers.
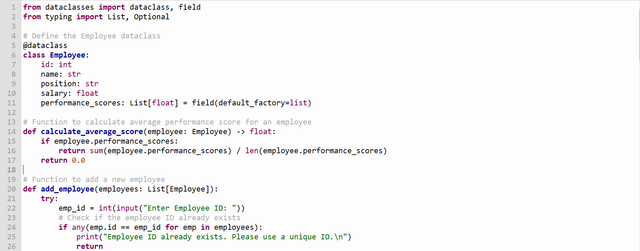
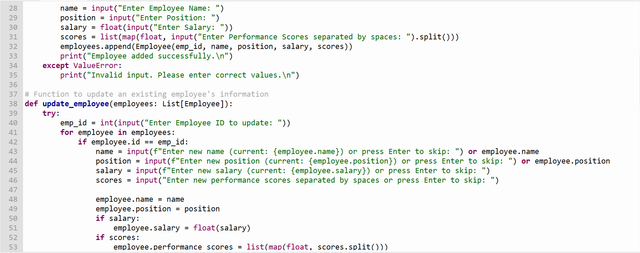
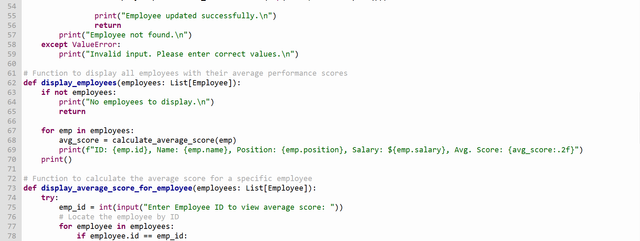
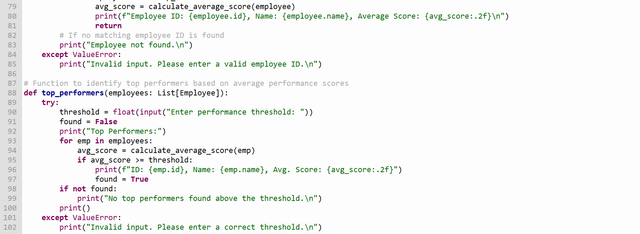
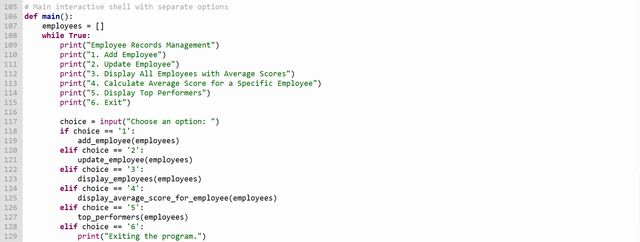

It is the complete python program to manage the records of the employee using dataclass
. The explanation of the code is given below:

from dataclasses import dataclass, field
: Imports the dataclass
decorator and field
function from Python's dataclasses
module. The dataclass
decorator simplifies creating classes that store data, while field
helps specify default values.
from typing import List, Optional
: Imports List
and Optional
from the typing
module, enabling type annotations for lists and optionally provided values.
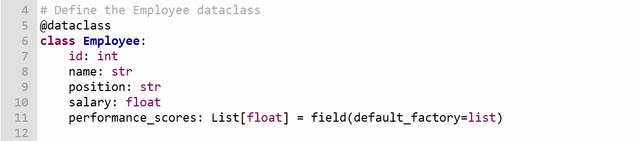
@dataclass
: Decorates the Employee
class, allowing it to automatically generate common methods like __init__
and __repr__
.
class Employee
: Defines a class named Employee
to represent employee data.
id: int
: Specifies that each employee has an integer id
.
name: str
: Specifies a name
field for the employee's name.
position: str
: Specifies a position
field for the employee's job title.
salary: float
: Specifies a salary
field as a floating-point number.
performance_scores: List[float] = field(default_factory=list)
: Defines performance_scores
as a list of floats, with default_factory=list
initializing it as an empty list if not specified.

def calculate_average_score
: Defines a function that calculates the average score for an employee.
if employee.performance_scores:
: Checks if the employee has any performance scores.
return sum(employee.performance_scores) / len(employee.performance_scores)
: Calculates and returns the average by dividing the sum by the count of scores.
return 0.0
: If there are no scores, it returns 0.0
as the average.
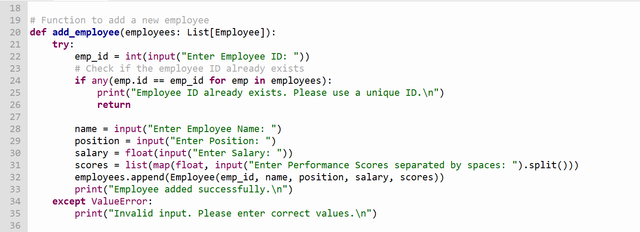
def add_employee
: Defines a function to add a new employee to the list of employees.
emp_id = int(input("Enter Employee ID: "))
: Prompts the user to input an employee ID and converts it to an integer.
if any(emp.id == emp_id for emp in employees):
: Checks if the provided ID already exists in the employee list.
print
and return
: If the ID exists, prints a message and exits the function.
Prompts the user to input values for name
, position
, salary
, and performance_scores
.
employees.append(...)
: Creates an Employee
instance with provided details and adds it to the list.
print
: Confirms successful addition.
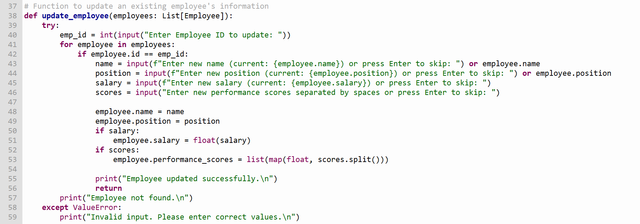
def update_employee
: Defines a function to update an employee’s details.
emp_id = int(input("Enter Employee ID to update: "))
: Prompts for the employee’s ID to update.
for employee in employees:
: Iterates over the list of employees.
if employee.id == emp_id:
: Checks if the current employee’s ID matches the input.
User input lines: Prompts for each attribute, allowing the user to press Enter to skip updating.
Attribute updates: Updates the employee’s attributes if input is provided.
print
and return
: Confirms success and exits. If no match is found, a message indicates this.

display_employees
: Lists all employees with average scores.
for emp in employees:
: Loops through employees, calculates, and displays each employee’s details.
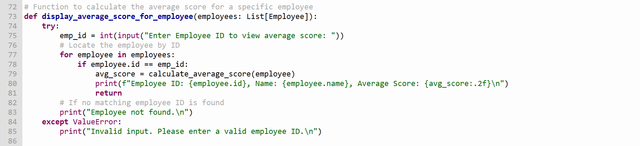
display_average_score_for_employee
: Finds and displays a specific employee’s average score based on their ID.
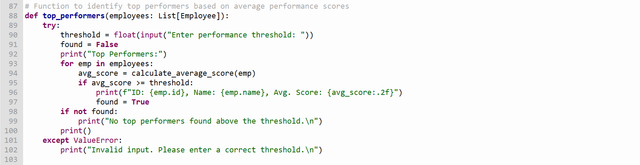
top_performers
: Identifies and lists employees with average scores at or above a given threshold.
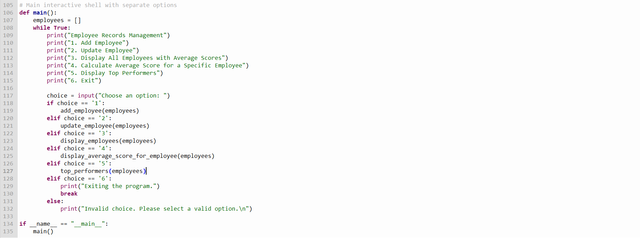
main
: Provides an interactive menu for managing employee records, with a loop for handling user input until the program exits. Each choice calls the respective function.
Comprehensive Student Grades Analysis
Utilize NumPy's structured arrays to store and analyze student grades, including calculating averages, identifying top students, and analyzing subject-wise performance.
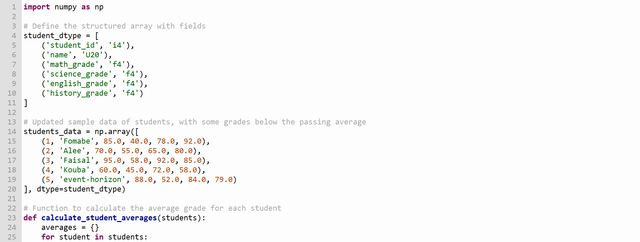
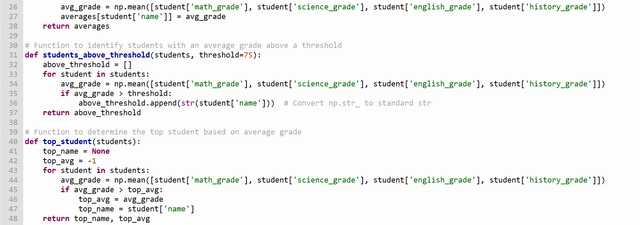
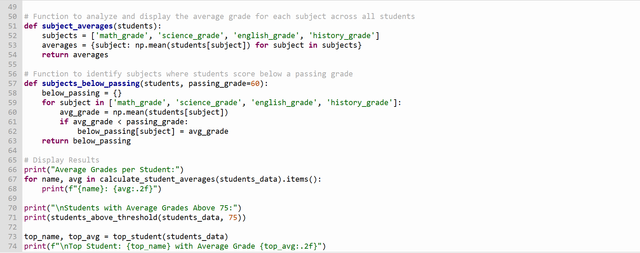

Importing NumPy

This imports the NumPy library, which provides functions to create and manipulate arrays efficiently.
Structured Array

This student_dtype
variable defines the structure (data type) for each student record, with:
student_id
as a 4-byte integer (i4
)name
as a 20-character Unicode string (U20
)- Each grade field (
math_grade
,science_grade
, etc.) as a 4-byte float (f4
).
Initialize Sample Data

This students_data
array holds sample student data with IDs, names, and grades in four subjects. The structured array format allows each entry to be accessed by name (e.g., student['math_grade']
).
Calculate Average Grades for Each Student

This function iterates over each student, calculates the average of their grades, and stores it in a dictionary (averages
) with the student’s name as the key.
Identify Students Above a Threshold

This function identifies students whose average grade is above a specified threshold
. It collects their names in a list and returns it.
Determine the Top Student by Average Grade

This function finds the student with the highest average grade:
- It initializes
top_name
asNone
andtop_avg
as-1
to ensure any valid average grade will be higher. - For each student, it calculates the average grade.
- If the average grade is higher than the current
top_avg
, it updatestop_avg
andtop_name
with the current student's data. - Finally, it returns the name and average grade of the top student.
Calculate Average Grades for Each Subject

This function calculates the average grade for each subject across all students:
- It iterates over each subject, computes the mean for that subject, and stores it in a dictionary.
- This dictionary,
averages
, maps each subject name to its average grade across all students.
Identify Subjects Below a Passing Grade

This function finds subjects with average grades below a specified passing_grade
:
- It iterates through each subject, calculates its average grade, and checks if it is below
passing_grade
. - If a subject’s average is below this threshold, it adds the subject and its average grade to the
below_passing
dictionary. - This dictionary is then returned, showing which subjects need improvement.
Display Results
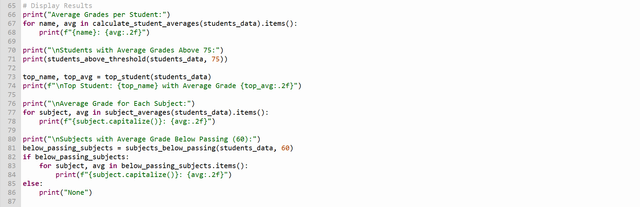
This final section prints the results of each analysis:
- Average Grades per Student: Calls
calculate_student_averages
and prints each student’s average grade. - Students with Average Grades Above 75: Calls
students_above_threshold
to list students with averages above 75. - Top Student: Calls
top_student
to display the student with the highest average. - Average Grade for Each Subject: Calls
subject_averages
to show average grades by subject. - Subjects Below Passing Grade: Calls
subjects_below_passing
and displays subjects that have an average grade below 60. If no subject falls below this threshold, it prints "None".
This code structure efficiently organizes student data and performs multiple types of analyses, making it easy to expand or adjust thresholds and criteria as needed.
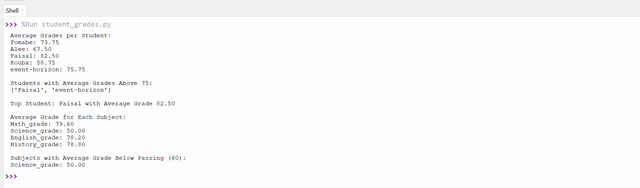
This is the output of the program after compiling all the code.
Enhanced Inventory Management System
Develop an inventory management system using namedtuple to track products, including functionalities for low stock alerts and identifying high-value products.
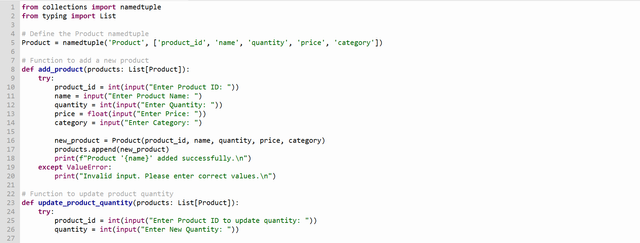
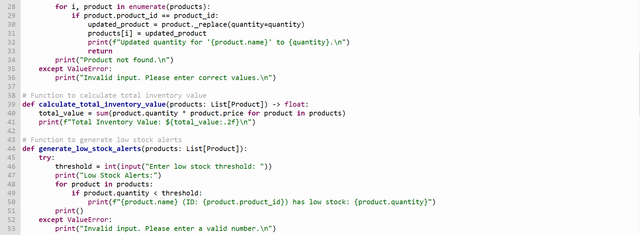
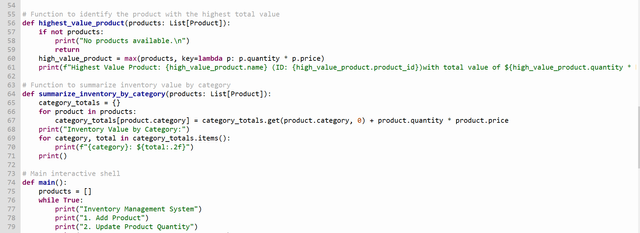
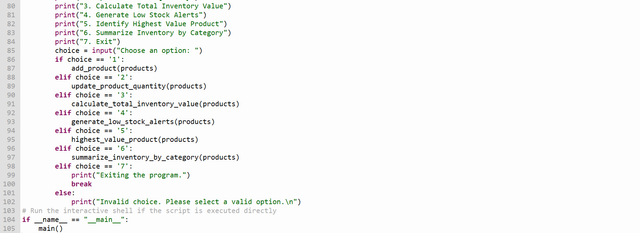
Here’s a complete breakdown of the inventory management code while explaining each function and part of the code in detail:
Imports and Named Tuple Definition

from collections import namedtuple
: Importsnamedtuple
from Python’scollections
module, allowing us to create a simple class-like structure for storing related data.from typing import List
: ImportsList
fromtyping
to annotate the type of list we use in functions.

- Defines a
Product
named tuple with fields:product_id
,name
,quantity
,price
, andcategory
. Each instance ofProduct
will store these details for a specific item in the inventory.
Function Definitions
Each function manages a specific part of the inventory system, allowing for modular operations that can be accessed individually through the main interactive shell.
Function: add_product
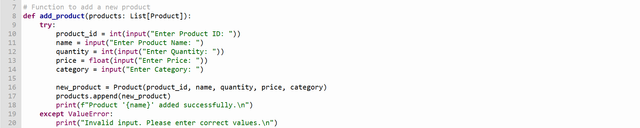
- Purpose: Allows the user to add a new product to the inventory list.
- Steps:
- Prompts for product details (
product_id
,name
,quantity
,price
,category
) and converts input to the appropriate data type. - Creates a new
Product
instance and appends it to theproducts
list. - Handles
ValueError
exceptions to ensure valid data types (e.g., integers for IDs and quantities, floats for prices).
- Prompts for product details (
Function: update_product_quantity
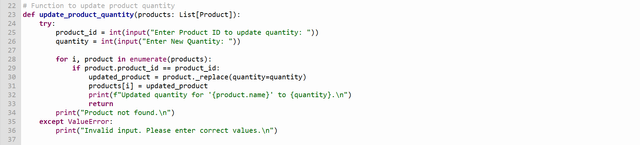
- Purpose: Updates the quantity of a specified product.
- Steps:
- Prompts for the
product_id
and newquantity
. - Searches for the product by
product_id
in theproducts
list. - If found, updates the product’s quantity using
_replace()
(a method fornamedtuple
instances to return a modified copy). - If not found, prints "Product not found." Handles
ValueError
exceptions for input.
- Prompts for the
Function: calculate_total_inventory_value

- Purpose: Calculates and displays the total monetary value of the inventory.
- Steps:
- Multiplies each product’s
quantity
by itsprice
and sums the values for all products. - Prints the total inventory value, formatted to two decimal places.
- Multiplies each product’s
Function: generate_low_stock_alerts

- Purpose: Provides alerts for products that have a quantity below a specified threshold.
- Steps:
- Prompts for a
threshold
and iterates overproducts
. - If a product’s
quantity
is below the threshold, it is listed as low stock. - Catches
ValueError
exceptions for input validation.
- Prompts for a
Function: highest_value_product

- Purpose: Finds and displays the product with the highest total value (
quantity × price
). - Steps:
- If the
products
list is empty, it informs the user and returns. - Uses
max()
with a lambda function (p.quantity * p.price
) to find the product with the highest value.
- If the
Function: summarize_inventory_by_category

- Purpose: Calculates and displays the total value of products grouped by category.
- Steps:
- Iterates over
products
, accumulating each category’s total value incategory_totals
. - Prints each category with its total value, formatted to two decimal places.
- Iterates over
Main Interactive Shell
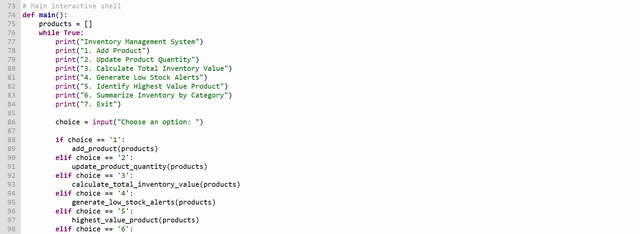

- Purpose: Provides a menu-driven interface to perform different inventory operations.
- Steps:
- Initializes an empty
products
list. - Displays the menu and prompts the user to choose an option.
- Based on the choice, calls the corresponding function to add a product, update a quantity, calculate total value, generate low stock alerts, find the highest value product, summarize by category, or exit the program.
- Initializes an empty
Advanced Customer Orders Processing
Create a program to process customer orders using NumPy's structured arrays, including functionalities for calculating order totals, identifying large orders, and analyzing customer purchasing patterns.
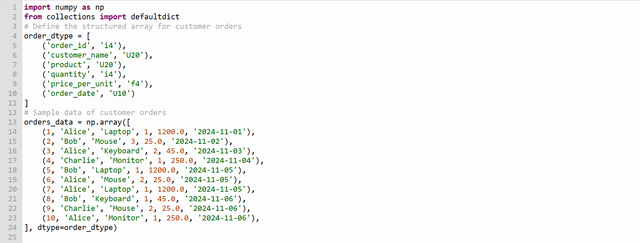
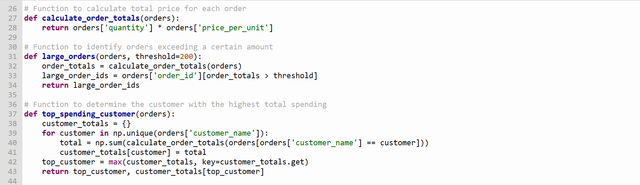
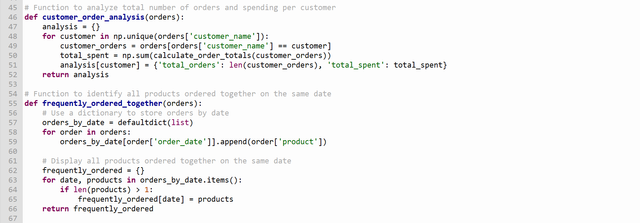
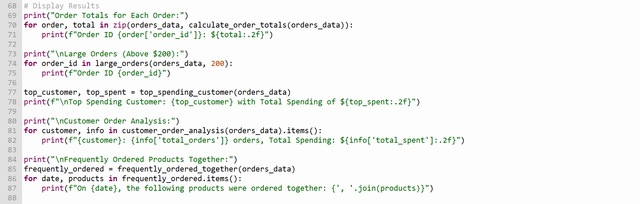
Here is the complete breakdown of the python program to process the customer orders using NumPy's structured arrays.
Imports

numpy
(imported asnp
): This library is used for numerical operations, especially with arrays, and is used here for structured arrays and numerical calculations.defaultdict
fromcollections
: This is a dictionary subclass that provides a default value for non-existent keys, useful here for grouping products by date.
Define the Structured Array for Orders

- This defines the structure of each order record, specifying the fields and data types:
order_id
: integer (4 bytes, ori4
).customer_name
: Unicode string with a max length of 20 characters (U20
).product
: Unicode string up to 20 characters.quantity
: integer for the quantity of each product.price_per_unit
: float (4 bytes).order_date
: Unicode string with a max length of 10 characters (format: 'YYYY-MM-DD').
Sample Data of Customer Orders

- This
orders_data
array uses theorder_dtype
structure to store each order as a record. It contains sample orders with details such asorder_id
,customer_name
,product
,quantity
,price_per_unit
, andorder_date
.
Functions
Calculate Total Price for Each Order
- This function computes the total cost per order by multiplying the
quantity
andprice_per_unit
for each order in the array.
- This function computes the total cost per order by multiplying the
Identify Large Orders (Above a Threshold)
- This function identifies orders with a total value greater than a specified threshold (default of $200). It calculates totals using
calculate_order_totals
and then filters orders where the total exceeds the threshold, returning theorder_id
s.
- This function identifies orders with a total value greater than a specified threshold (default of $200). It calculates totals using
Determine the Customer with the Highest Total Spending
- This function first creates a dictionary
customer_totals
to track total spending per customer. For each unique customer, it calculates the sum of order totals where thecustomer_name
matches. The customer with the highest total spending is then identified and returned.
- This function first creates a dictionary
Analyze Total Orders and Spending Per Customer
- This function provides a summary of total orders and spending per customer by grouping each customer's orders, calculating their total spending, and counting their orders. The result is stored in
analysis
, with each customer's name as a key.
- This function provides a summary of total orders and spending per customer by grouping each customer's orders, calculating their total spending, and counting their orders. The result is stored in
Identify Products Ordered Together on the Same Date
- This function groups products by their
order_date
using adefaultdict
to build a list of products ordered on each date. - If more than one product was ordered on the same date, the date and the products are added to the
frequently_ordered
dictionary. This dictionary is returned, showing which products were ordered together on specific dates.
- This function groups products by their
Display Results

- This loop displays the total price for each order by iterating through
orders_data
and calculated totals.

- This displays the
order_id
of each order with a total price above $200.

- This section displays the customer with the highest spending.

- This outputs a summary of the number of orders and total spending per customer.

- This displays the products ordered together on each date with multiple items, formatted to show the date and products ordered.
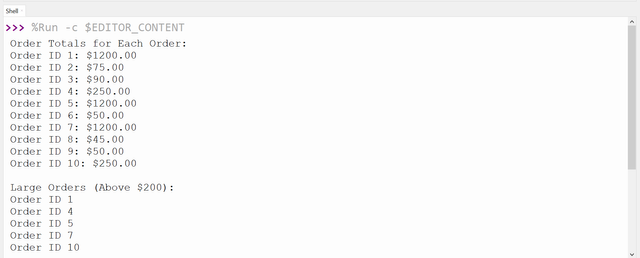
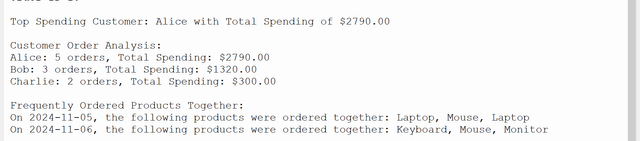
The program provides a complete system for tracking and analyzing orders, including identifying high value customers, orders exceeding a price threshold and products frequently ordered together. It uses numpy
for array management and collections.defaultdict
for grouping making it both efficient and adaptable.
In-Depth Weather Data Analysis
Analyze weather data using dataclass and NumPy, including functionalities for calculating averages, identifying trends, and detecting anomalies.
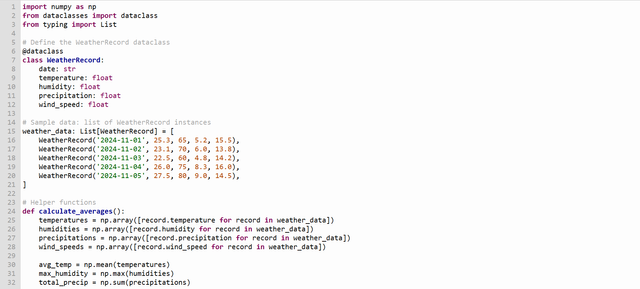
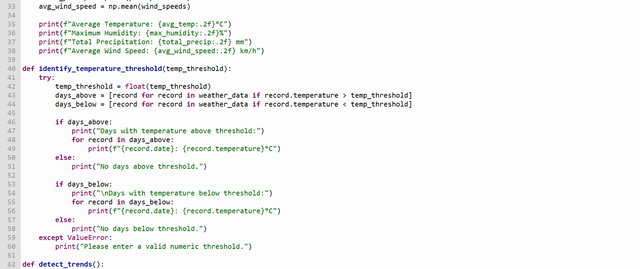
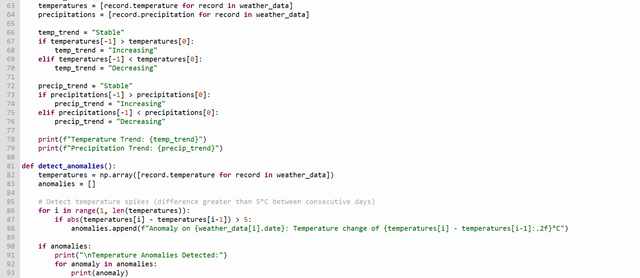
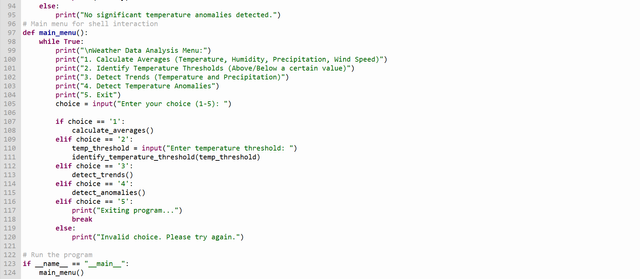
Here’s a complete breakdown of the program to analyze weather data using dataclass
and NumPy
.
Imports and Setup

numpy
: Used for numerical operations, particularly calculating averages, maximums, and summing lists.dataclasses.dataclass
: Used to create aWeatherRecord
class that holds structured weather data.typing.List
: Specifies a list ofWeatherRecord
instances.
WeatherRecord
Dataclass

WeatherRecord
: This is a dataclass that stores weather data for each day, including the date, temperature, humidity, precipitation, and wind speed.
Sample Data

weather_data
: A list ofWeatherRecord
instances representing sample weather data.
Helper Functions
calculate_averages
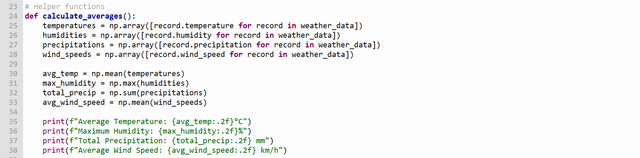
- This function calculates:
- Average temperature
- Maximum humidity
- Total precipitation
- Average wind speed
- It uses numpy functions like
np.mean
,np.max
, andnp.sum
.
identify_temperature_threshold
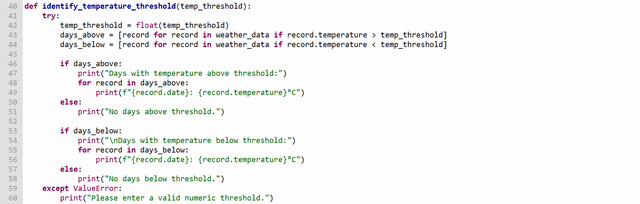
- This function accepts a
temp_threshold
, identifies days where the temperature is above or below it, and prints these dates.
detect_trends
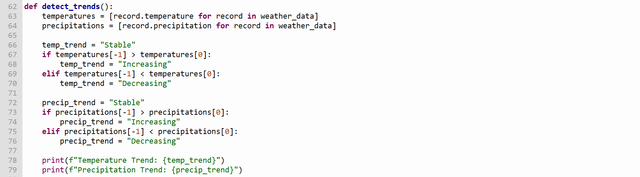
- This function checks if temperature and precipitation values are increasing, decreasing, or stable based on the first and last values in the dataset.
detect_anomalies
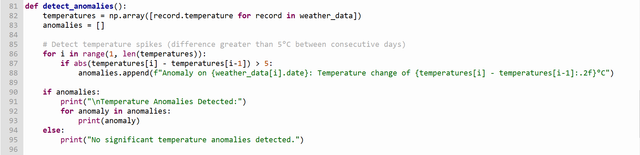
- This function detects temperature spikes (changes greater than 5°C between consecutive days) and lists these as anomalies.
main_menu
Function
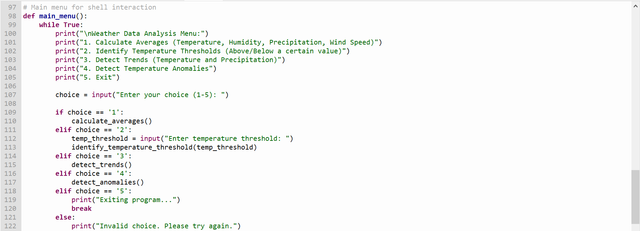
The main menu prompts the user with five options:
- Calculate averages
- Identify temperature thresholds
- Detect trends
- Detect temperature anomalies
- Exit
Depending on the choice, the relevant function is called, and the user can view or analyze the weather data interactively.
Running the Program

main_menu
runs as the program’s entry point, enabling interactive analysis of weather data based on the provided options.
Congratulations, your post has been upvoted by @scilwa, which is a curating account for @R2cornell's Discord Community. We can also be found on our hive community & peakd as well as on my Discord Server
Felicitaciones, su publication ha sido votado por @scilwa. También puedo ser encontrado en nuestra comunidad de colmena y Peakd así como en mi servidor de discordia