Domain Steem with JavaScript: Lesson #4 - Broadcasting operations part 1: Transferring, and posting.
Created with canva
We have already learned the essentials to be able to read and interpret the information in the blockchain, now we go to the fun part of the matter, we are going to start writing transactions directly from our code, which will allow us to start creating applications capable of reacting without us being present.
To perform operations in Steem we will require a library such as Steem JS or DSteem, since POST queries do not support this type of transactions, so from here we will work only with libraries.
Broadcasting operations
To perform operations the Steem JS library has methods for the most common, you can see the guide here:
For this occasion I have created an account that I will dedicate exclusively to tests, and thus not fill my main account with spam since we are going to perform several actions, this account is @eight888 and will only have that purpose, once the course is over the account will be inactive and will only be used again in case of future activities for educational or practical purposes.
As you can see, it is a totally clean account, so the first thing we need to do is add SP to be able to perform operations. Today all the operations that we are going to carry out will be with code to practice to the maximum, we are going to create the script to make delegations.
To make a delegation we need to take into account a number of things:
1- The API must receive the amount to delegate in vesting_shares, but we are not going to enter quantities in this unit since we know it is working with SP, so we need to obtain the amount of STEEM PER MILLION VESTING to perform the conversion, for this we have a function that returns this data and calculates the desired value automatically:
2- The formula we saw earlier is used to convert vesting_shares to SP, so we must invert it to obtain the desired result, it would look like this:
SP * 1000000 / per_mvests
For this we create a function
Having this, we can use the method steem.broadcast.delegateVestingShares
to make the delegation, we will need the account that you are going to delegate with your ACTIVE KEY, the account that you are going to receive and the amount in SP, we will ask the user for this data. After having obtained this information we transform the amount of SP to vesting shares, and finally we execute the operation.
We have installed the readline-sync library in node to be able to ask the user for data from the console.
It is important to mention that the amount in VESTING SHARES must have 6 decimals, for this you can use the .toFixed(6) method to correctly format the amount. It is also important to add the VESTS symbol.
Once the operation is executed, we check our account and we already have the delegated SP:
We have fulfilled our task, now we can use this new account to perform operations.
Creating a post
To create a post we will use the method steem.broadcast.comment
, this method will require the following data:
- parent_author: This value is left blank, it is only used if you are going to comment on a post from someone else, if you create a post of 0, you only have an empty string.
- parent_permlink: Again if you are creating a post of 0, in parent_permlink you must place the tag of the community where you will publish or the first tag of your post if it will be on your blog.
- author: the name of the account to publish (private posting key required)
- permlink: it is the unique link to your publication, you must create an algorithm to create a unique and random permlink preferably, the permlink is what goes in the url right after your user.
- title: the title of the post.
- body: the content of the post.
- json_metadata: some important metadata, this section includes tags, and information such as from which app the post was published.
This is the code:
let steem = require("steem");
const readlineSync = require('readline-sync');
(async ()=> {
let tags = ["steem", "development", "steemjs"];
let post = {
author:"eight888",
title:"Hello world! This is a post for the Steem JS Course",
body:`
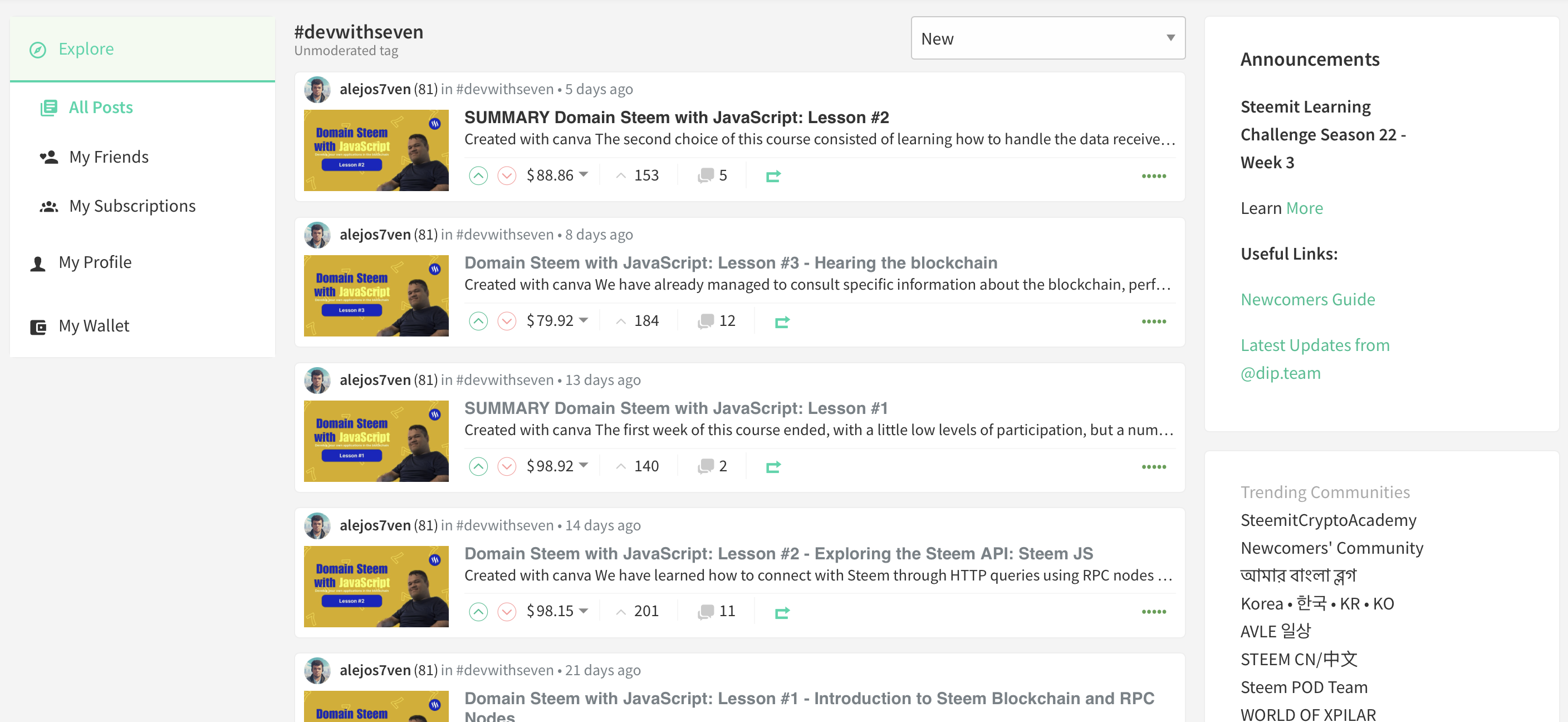%202.34.02%E2%80%AFp.%C2%A0m..png)
Hello!
This is a post created using Steem JS code for the Steem JS course. In this lesson you are learning how to broadcast operations. You can follow the course here:
|Thumb|Post|
|---|---|
|<img src="https://steemitimages.com/200x400/https://cdn.steemitimages.com/DQmTUTzrPhDHPvmqwLwnU5tuR7P3aNfEcPfnacf6HD42rWU/d.jpg">|[Domain Steem with JavaScript: Lesson #1 - Introduction to Steem Blockchain and RPC Nodes](https://steemit.com/@alejos7ven/domain-steem-with-javascript-lesson-1-introduction-to-steem-blockchain-and-rpc-nodes)|
|<img src="https://steemitimages.com/200x400/https://cdn.steemitimages.com/DQmcbBwPMzodPpfRG1EdGXSHP17MddVhLmtsWV1pTVaeZ7a/2.jpg">|[Domain Steem with JavaScript: Lesson #2 - Exploring the Steem API: Steem JS](https://steemit.com/@alejos7ven/domain-steem-with-javascript-lesson-2-exploring-the-steem-api-steem-js)|
|<img src="https://steemitimages.com/200x400/https://cdn.steemitimages.com/DQmRckq64fXSRXfHjSHzZZ2jJaS6AVjasJ2retqYaiFQMbP/3.jpg">|[Domain Steem with JavaScript: Lesson #3 - Hearing the blockchain](https://steemit.com/@alejos7ven/domain-steem-with-javascript-lesson-3-hearing-the-blockchain)|
I hope you are enjoying it,
Let's rock with Steem!`,
parent_author:"",
parent_permlink:tags[0],
permlink: "steemjs-course-" + new Date().getDate() +"-id-" + Math.random().toFixed(4).substring(2),
json_metadata:JSON.stringify({ tags:tags, app:'steemjscourse/1.0' })
}
let wif = readlineSync.question("Are you sure do you want to create this post? Enter your posting key ");
steem.broadcast.comment(wif,
post.parent_author,
post.parent_permlink,
post.author,
post.permlink,
post.title,
post.body,
post.json_metadata, function(err, result) {
console.log(err, result);
});
})()
Created an arrangement with all the necessary variables, create a unique permlink using specific words of the course, the date, and random numbers, in the body add the text of a simple post and in the metadata indicate that it was created with JS for the course.
After entering the posting key the post was created:
Thumb | Author | Post |
---|---|---|
![]() | @eight888 | Hello world! This is a post for the Steem JS Course |
And finally, if we see the transaction we will see that it was created by our code, and not by Steemit.
- Create a bot that detects when you vote for someone else's publication, when detecting your vote creates an automatic comment on that publication you voted for (the app that created your comment should be called your username and version 1.0, example
app:'alejos7ven/1.0'
) [5 PTS] - Use SteemWorld, Ecosynthesizer, or any other explorer to show the transactions you have created, leave link just like me. [2 PTS]
- Transfer 0.001 STEEM to @eight888 using Steem JS, add in the memo the title of this lesson. [3 PTS]
Rules
- The content must be #steemexclusive.
- The article must contain the tag #steemjs-s22w4.
- Plagiarism is not allowed.
- The link of your task must be added in the comments of this publication.
- The course will be open for 7 days from 00:00 UTC on January 6. After the deadline, users will be able to continue participating without applying for prizes with the aim of allowing more people in time to take advantage of this content.
This post has been upvoted by @italygame witness curation trail
If you like our work and want to support us, please consider to approve our witness
Come and visit Italy Community
Great explanation on broadcasting operations in Steem using JavaScript! Your detailed breakdown of the transfer and posting processes makes it accessible, even for those new to blockchain programming. I have a question: do you recommend any de additional libraries or tools for debugging these operations during testing? Following your lessons has been incredibly insightful; thank you for sharing!
I don’t know any library to do it from know, maybe some student of this course will be the hero to do something like this.
Thanks for the support!
Hi @alejos7ven, first, thank you for this lesson and this challenge. I have a question about the third question in the homework:
"Transfer 0.001 STEEM to @eight888 using Steem JS, and add the title of this lesson in the memo. [3 PTS]"
You didn’t show us how to make a transfer, only delegation, so we would like to know how to do that. I don't have any STEEM; I only have STEEM Power. It takes me a week to power down, and that will overlap with the end of this challenge. So, is there any solution? Maybe you could ask us to do a transfer or delegation instead, not only a transfer?
You can do the transfer by studying the method "transfer" in the documentation, it’s easy because you don’t have to transform any value, just add 3 decimals and the symbol STEEM/SBD
The idea is you to learn how to broadcast operations by your own using different methods, cause it is common that you need to do an specific operation and you have to learn how to use a new method.
If you don’t have Steem, you could replace this task with accountWitnessVote method, try removing and giving again a witness vote.
Let me know if you need help with something,
0.45 SBD,
0.26 STEEM,
1.86 SP
thank you for the advice! I'll review the "transfer" method in the documentation to understand how it works. Note that there is no need to change the value. Just add 3 decimal places and a sign (STEEM/SBD).
Since I don't have STEEM, I'll use the accountWitnessVote method instead. I really appreciate the flexibility of working for those who don't have STEEM. Thank you for supporting this process!
Best regards
You created exclusive and quality content
We wish you a happy new year 2025
Team 01 - Steemit Explorers Team
@graceleon
Здорово, что начал появляться такой полезный контент! Друг, ты крутой! Успехов тебе!
Thank you!☺️
Check out my entry: Mastering Steem Blockchain Operations with JavaScript. Also, the post is shared on Twitter: Twitter Link.
Могу ли я задать несколько вопросов по уроку в телеграмм?
Yes
Как мне найти вас в телеграмм?
Alejos7ven
Hello @alejos7ven! 👋
I really enjoyed your post and I have voted for it.
This comment was generated by my bot for the completion of my task. 🚀
App: mohammadfaisal/1.0
Here is my entry: https://steemit.com/steemjs-s22w4/@mohammadfaisal/domain-steem-with-javascript-lesson-4-broadcasting-operations-part-1-transferring-and-posting
Saludos amigo.
Una pequeña consulta. El readline no me permite leer los datos, me sale este error
Sera debido a que tengo una version de node.js 13.14.0 que es muy obsoleta. Ya hice el proceso de instalar el readline.
creo que es un error de node en si, desinstalalo y vuelvelo a instalar