Basic programming course: Lesson #5 Control structures. Part 2. Cycles. [ESP-ENG]
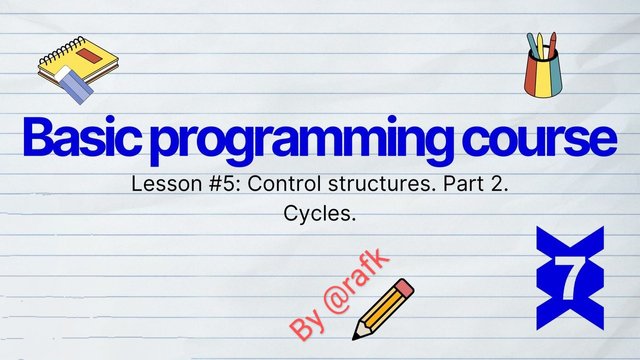
Hi everyone greetings to you. It's been an amazing week of contest. I have learned a lot from the previous lessons based on algorithm. To continue my journey in this week's engagement challenge, I will be putting my entry on control structures again but this time around and cycles.
To begin with, we first of all household, understand how the cycle works, this is going to be done with some base guideline questions from the main post. You can check it here
Before going to cycles, let's talk about counter and accumulators. Counters and accumulators are variables used in programming algorithms to track and accumulate values.
Counters are used to count the number of occurrences of a specific event or condition.
Accumulators are used to store and progressively update a value by adding or subtracting other values.
Both counters and accumulators are essential tools for various programming tasks, such as loop control, frequency analysis, summation, product calculation, and average computation.
Explain the cycles in detail. What they are, how are they used? Explain the difference between the While and Do-While cycle in algorithm?
Cycles or loops, are a programming concept that permits us to execute a block of code multiple times. They're like a loop-to-loop on a roller coaster: you will keep going around and around until you reach your stop🛑.
Types of Cycles
There are three main types of cycles in programming:
For Loops:**
Think of it as a countdown timer. You set a starting point, an ending point, and a way to count down.
Example: Imagine you want to count from 1 to 10. You could use a for loop:
for (int i = 1; i <= 10; i++) {
Console.WriteLine(i);
}
While Loops:
Think of it as a "keep going until" loop. It the cycle goes on repeating as so far as a given condition stays true.
Example: think of a case where you keep asking a user for a number until they enter a positive number:
int number;
do {
Console.WriteLine("enter a positive number:");
number = int.Parse(Console.ReadLine());
} while (number <= 0);
Do-While Loops:
It always runs at least once, then checks the condition to see if it should repeat.
- Example: Imagine you want to make sure a user enters a valid input, even if their first try is wrong:
int number;
do {
Console.WriteLine("input a positive number between 1 and 10:");
number = int.Parse(Console.ReadLine());
} while (number < 1 || number > 10);
The Difference Between While and Do-While
The main difference that exist between while and do-while loops
is when the condition is checked. In the case of a while loop, the condition is checked before the code block is executed. While for a do-while loop, the condition is checked after the code block is executed.
In summary :
- While loop:
"If the condition is true, execute a given task."
- Do-while loop:
"Do this, afterwards if the condition is true, repeat cycle."
Why implement one over the other? This is up to the user on what they're aiming to achieve. If you want to ensure that a block of code is executed at least once, regardless of the condition, a do-while loop is the way to go. If not, a while loop is a sufficient approach.
Investigate how the Switch-case structure is used and give us an example.
Take for instance, you're at a restaurant and the waiter moves over to asks you what you'd like to order. Regardless of your response, he'll bring you a specific dish. This is practically how the switch-case structure works in algorithms.
Mode of operation
The switch-case structure is typically a decision-making statement that allows a program to execute different blocks codes based on the value of an expression. It's particularly applicable in cases where you have multiple possible outcomes and want to limit a series of nested if-else statements.
This is how it works:
Expression Evaluation: The switch-case statement starts by evaluating an expression. The expression can be any of the categories: a number, character, or string.
Case Matching: The evaluated value is then compared to each case label within the switch statement. If a given match is found, the required code block is executed.
Default Case (Optional): If no case matches the evaluated value, the default case (if present) is executed.
Example: A Simple Menu
Let's imagine we want to create a simple menu-driven program where by the user can Select from different options. Here's how we can use a switch-case statement to handle the user's choice in cpp.
#include <iostream>
using namespace std;
int main() {
int choice;
cout << "Menu:\n";
cout << "1. Option 1\n";
cout << "2. Option 2\n";
cout << "3. Option 3\n";
cout << "Enter your choice: ";
cin >> choice;
switch (choice) {
case 1:
cout << "You chose Option 1.\n";
break;
case 2:
cout << "You chose Option 2.\n";
break;
case 3:
cout << "You chose Option 3.\n";
break;
default:
cout << "Invalid choice.\n";
break;
}
return 0;
}
In this example;
- The user is prompted to enter a choice.
- The switch statement will have to evaluates the choice variable.
- Based on the value of choice, the corresponding case is executed.
- If the user in attempt input an invalid choice, the program's default case will be executed.
Key Points:
The break statement is very crucial to prevent "fall-through" to a subsequent cases. Without it, the code will continue to execute until it encounters a break or the end of the switch statement.
The default case is optional but often useful for handling unexpected or invalid inputs.
The switch statement can be incorporated within other switch statements in application of more complex decision-making scenarios.
The switch-case structure is a diverse powerful tool for writing clean and efficient code, especially when dealing with multiple possible outcomes based on a single value. By understanding how it works, we can effectively use it in our programming projects.
Explain what the following code does:
Let's break it down step by step:
Variable Definitions
op, n, ns, a:
These are integer variables.
op
: Stores the user's choice from the menu.
n
: Used to store how many numbers the user wants to sum.
ns:
Stores individual numbers to be summed.
a
: Holds the cumulative sum of the numbers.
exit:
A logic flag (likely a boolean) that controls when to end the program.
Initially:
exit is set to False, meaning the program will continue to run.
a is initialized to 0, so no sum has been performed yet.
Main Program Loop (Repeat...Until exit)
The main loop runs repeatedly until exit is set to True, meaning the program will keep asking for user input and performing tasks based on the input.
User Menu
Inside the loop, the following options are displayed to the user:
Option 1: Sum numbers.
Option 2: Show the result of the sum.
Option 3: End the program.
The program waits for the user to input a choice (op), which is handled by the Switch statement.
Switch Statement (Handling User Choices)
The switch-case structure determines what action to take based on the value of op:
Case 1: Sum Numbers
The user is asked how many numbers they want to sum (this number is stored in n).
A For loop runs n
times, each time asking the user to input a number (ns)
. This number is added to the running total stored in a.
After completing the loop, it prints "Completed"
from which it waits for the user to press any button to continue.
Case 2: Show Results
The current value of a
, which holds the cumulative sum, is displayed to the user.
It then prompts the user to press any button to continue.
Case 3: End Program
This case clears the screen and prints a goodbye message ("Bye =)")
.
The exit flag is set to True, which will stop the main loop and end the program.
Default: Invalid Option
If the user enters a choice that is not in the range 1, 2, or 3
, it prints "Invalid option"
and request the user to try again until the condition is met.
Program End
Once exit becomes True, the Repeat...Until loop terminates, and the program finishes.
In Summary;
The code is a simple interactive program that allows users to:
- Sum a series of numbers.
Display the cumulative sum.
Exit the program.
The user can select what action are to be taken from a menu, and the program repeats the cycle until the user chooses the option to exit.
Write a program in pseudo-code that asks the user for a number greater than 10 (Do-While), then add all the numbers with an accumulator (While) Example: The user enters 15. The program adds up to 1+2+3+4+5+6+7+8+9+10+11+12+13+14+15
Define number, sum, counter as Integer
sum = 0
counter = 1
Do
Print "input a number greater than 10: "
Read number
While number <= 10
While counter <= number Do
sum = sum + counter
counter = counter + 1
EndWhile
Print "The sum of all numbers from 1 to ", number, " is: ", sum
Explanation:
Do-While Loop:
The program Will ask the user to input a number first.
If the number is less than or equal to 10, it keeps asking the user for a valid input (greater than 10).
While Loop:
Once a valid number is inputted, a While loop starts at 1 and runs until the counter reaches the user's entered number.
During each iteration, the current value of the counter is added to the sum, and the counter is increased to by 1.
Output:
After the loop finishes, the program prints the total sum of numbers from 1 to the number entered by the user.
Once complete, this is how the results will look like. This brings us to the end of today's task.
Quite an interesting exercise, I was able to do and learn more about cycles why taking notes on accumulators and counters. With more practice and dedication, I will be able to do further in this lesson.
To conclude, I would like to invite the following person to join me participate in this contest. @chant, @fombae and @simonnwigwe
Cc: @alejos7ven
Credit to: @rafk
Upvoted! Thank you for supporting witness @jswit.