Using Steem-API with Ruby Part 18 — Print Stacked Steem Token
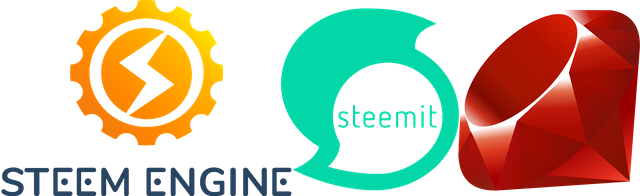
Repositories
SteemRubyTutorial
All examples from this tutorial can be found as fully functional scripts on GitHub:
- SteemRubyTutorial
- radiator sample code: Steem-Print-Balances.rb.
radiator
- Project Name: Radiator
- Repository: https://github.com/inertia186/radiator
- Official Documentation: https://www.rubydoc.info/gems/radiator
- Official Tutorial: https://developers.steem.io/tutorials-ruby/getting_started
Steem Engine

- Project Name: Steem Engine
- Home Page: https://steem-engine.com
- Repository: https://github.com/harpagon210/steem-engine
- Official Documentation: https://github.com/harpagon210/sscjs (JavaScript only)
- Official Tutorial: N/A
What Will I Learn?
This tutorial shows how to interact with the Steem blockchain, Steem database and Steem Engine using Ruby. When accessing Steem Engine using Ruby their only the radiator APIs available.
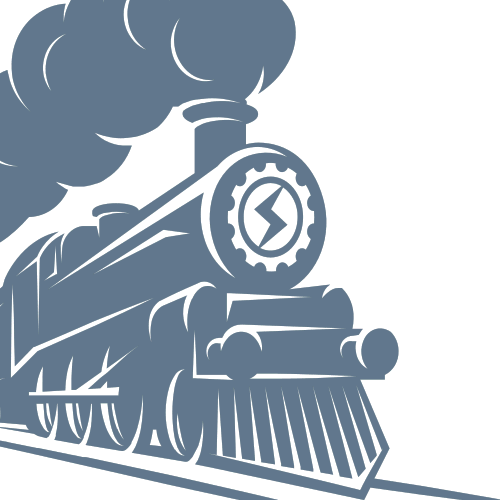
In this particular chapter you learn how extend Steem-Print-Balances.rb from the Print Account Balances improved tutorial so it prints the steem engine token as well.
Requirements
Basic knowledge of Ruby programming is needed. It is necessary to install at least Ruby 2.5 as well as the following ruby gems:
gem install bundler
gem install colorize
gem install contracts
gem install radiator
The tutorial build on top of the following previous chapters:
Difficulty
For reader with programming experience this tutorial is basic level.
Tutorial Contents
The stacked tokens are stored alongside the non stacked token inside the balances
table. Of course not all token are staked so you should check if the token
is stakingEnabled
.
Implementation using radiator
SCC::Balance
Take the staked token into account when calculating steem value of the account's balance.
Contract None => Radiator::Type::Amount
def to_steem
_steem = if @symbol == "STEEMP" then
@balance
elsif token.staking_enabled then
(@balance + @stake) * metric.last_price
else
@balance * metric.last_price
end
return Radiator::Type::Amount.to_amount(
_steem,
Radiator::Type::Amount::STEEM)
end
Add the staked token to the formatted printout.
Contract None => String
def to_ansi_s
_na = "N/A"
begin
_steem = self.to_steem
_sbd = self.to_sbd
_staked = self.token.staking_enabled
_retval = if _staked then
("%1$15.3f %2$s".white +
" %3$15.3f %4$s".white +
" %5$18.5f %7$-12s".blue +
" %6$18.6f %7$-12s".blue) % [
_sbd.to_f,
_sbd.asset,
_steem.to_f,
_steem.asset,
@balance,
@stake,
@symbol]
else
("%1$15.3f %2$s".white +
" %3$15.3f %4$s".white +
" %5$18.5f %7$-12s".blue +
" %6$18s".white) % [
_sbd.to_f,
_sbd.asset,
_steem.to_f,
_steem.asset,
@balance,
_na,
@symbol]
end
rescue KeyError
_retval = (
"%1$15s %2$s".white +
" %3$15s %4$5s".white +
" %5$18.5f %7$-12s".blue +
" %6$18.6f %7$-12s".blue) % [
_na,
_na,
_na,
_na,
@balance,
@stake,
@symbol]
end
return _retval
end
SCC::Token
Add additional attributes describing staked token.
attr_reader :key, :value,
:symbol,
:issuer,
:name,
:metadata,
:precision,
:max_supply,
:supply,
:circulating_supply,
:staking_enabled,
:unstaking_cooldown,
:delegation_enabled,
:undelegation_cooldown,
:loki
Contract Any => nil
def initialize(token)
super(:symbol, token.symbol)
@symbol = token.symbol
@issuer = token.issuer
@name = token.name
@metadata = JSON.parse(token.metadata)
@precision = token.precision
@max_supply = token.maxSupply
@supply = token.supply
@circulating_supply = token.circulatingSupply
@stakingEnabled = token.staking_enabled
@total_staked = token.total_staked
@unstakingCooldown = token.unstaking_cooldown
@delegationEnabled = token.delegation_enabled
@undelegationCooldown = token.undelegation_cooldown
@loki = token["$loki"]
return
end # initialize
Steem-Print-Balances.rb
Update printout and add calculation of accounts steem engine token values.
_scc_balances = SCC::Balance.account account.name
_scc_value = Radiator::Type::Amount.new("0.0 SBD")
_scc_balances.each do |_scc_balance|
token = _scc_balance.token
puts(" %1$-22.22s = %2$s" % [token.name, _scc_balance.to_ansi_s])
# Add token value (in SDB to the account value.
begin
_sbd = _scc_balance.to_sbd
_scc_value = _scc_value + _sbd
_account_value = _account_value + _sbd
rescue KeyError
# do nothing.
end
end
puts((" Account Value (engine) = " + "%1$15.3f %2$s".green) % [
_scc_value.to_f,
_scc_value.asset])
puts((" Account Value = " + "%1$15.3f %2$s".green) % [
_account_value.to_f,
_account_value.asset])
Hint: Follow this link to Github for the complete script with comments and syntax highlighting : Steem-Print-Balances.rb.
The output of the command changes from previous only printing the steem engine token have not been stacked:
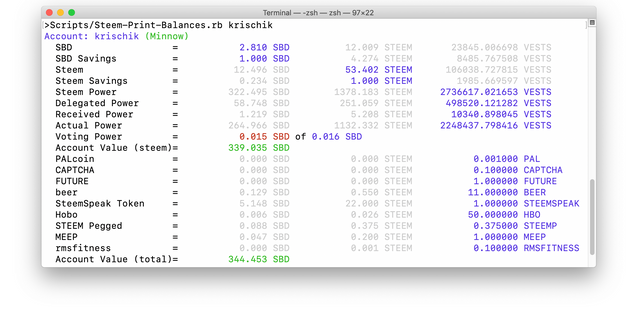
to now printing the values of the staked Steem Engine Token as well:
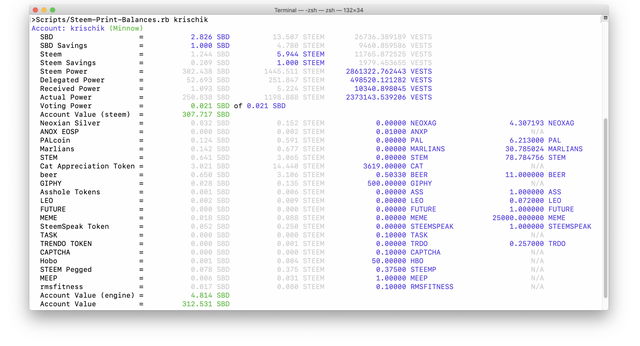
Curriculum
First tutorial
Previous tutorial
Next tutorial
Proof of Work
- GitHub: SteemRubyTutorial Issue #20
Image Source
- Ruby symbol: Wikimedia, CC BY-SA 2.5.
- Steemit logo Wikimedia, CC BY-SA 4.0.
- Steem Engine logo Steem Engine
- Screenshots: @krischik, CC BY-NC-SA 4.0







Hi, @krischik!
You just got a 8.68% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
/ᐠ.。.ᐟ\