Programming Lessons: Let's learn a little of C# - Lesson 03
Hello Guys
Some days ago I started a new topic. I wanna teach how to program a little. As you probably know I'm a software developer so I wanna bring some of the stuff that I do daily. With this topic I do not wanna do something hard to understand but the basic concepts of programming.
We'll start with a simple topics and the language that we'll use is C#.
About C#
C# is a simple, modern, general-purpose, object-oriented programming language developed by Microsoft within its .NET initiative led by Anders Hejlsberg. This tutorial covers basic C# programming and various advanced concepts related to C# programming language.
First of all, if you wanna try the examples that I'll provide you can use this site to program online C#. Alternatively, you can install the free version on Visual Studio, the best tool to program C# here.
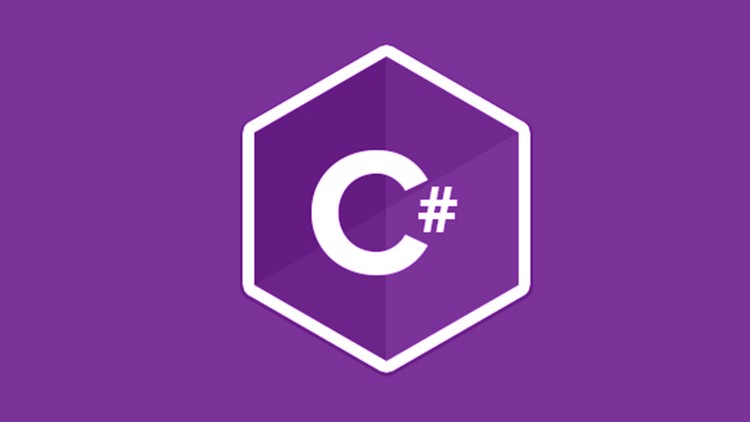
Lesson 03: Data Type
In C# the basic kind of variables are:
- Value types
- Reference types
Value Type
Value type variables can be assigned a value directly (Derived from System.ValueType). The value types directly contain data. Some examples are int, char, and float, which stores numbers, alphabets, and floating point numbers, respectively. When you declare an int type, the system allocates memory to store the value.
source
Type | Represents | Range |
---|---|---|
bool | Boolean value | True or False |
byte | 8-bit unsigned integer | 0 to 255 |
char | 16-bit Unicode character | U +0000 to U +ffff |
decimal | 128-bit precise decimal values with 28-29 significant digits | (-7.9 x 1028 to 7.9 x 1028) / 100 to 28 |
double | 64-bit double-precision floating point type | (+/-)5.0 x 10-324 to (+/-)1.7 x 10308 |
float | 32-bit single-precision floating point type | -3.4 x 1038 to + 3.4 x 1038 |
int | 32-bit signed integer type | -2,147,483,648 to 2,147,483,647 |
long | 64-bit signed integer type | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
sbyte | 8-bit signed integer type | -128 to 127 |
short | 16-bit signed integer type | -32,768 to 32,767 |
uint | 32-bit unsigned integer type | 0 to 4,294,967,295 |
ulong | 64-bit unsigned integer type | 0 to 18,446,744,073,709,551,615 |
ushort | 16-bit unsigned integer type | 0 to 65,535 |
Code
// Initializing Value Types
int myInt;
// Invoke default constructor for int type.
myInt = new int();
// Assign an initial value, 0 in this example.
myInt = 0;
You can find more information here.
Reference Type
The reference types do not contain the actual data stored in a variable, but they contain a reference to the variables. In other words, they refer to a memory location. You can find more information here.
C# also provides the following built-in reference types:
- dynamic
- object
- string
String Type
The string type is one of the most used when programming.
"The string type represents a sequence of zero or more Unicode characters. string is an alias for String in the .NET Framework. Although string is a reference type, the equality operators (== and !=) are defined to compare the values of string objects, not references. This makes testing for string equality more intuitive." source
string s1 = "Hello ";
string s2 = "World.";
// Concatenate s1 and s2. This actually creates a new string object and stores it in s1, releasing the reference to the original object.
s1 += s2;
System.Console.WriteLine(s1);
// Output: Hello World.
You can find more information here.
Hope you enjoy the first lesson @criptomaster
Nice post
Pleases vote mee too
Thanks :)
Very good post today my friend, keep up for success <3
Thanks :)
I challenge you to find all sources and cite them. Come back and edit your post with those sources (including code definitions, quotes, and/or code samples that you've used) and then respond to this comment to let me know that you have done so.
If you can do that, I'll drop another 100% upvote and resteem for the Post. I will hold off upvoting the post until I hear back from you.
Challenge accepted!
The text "You can find more information here." have the text that I'm using in the post. As you may know, I'm not inventing anything ... I'm sharing some information. The code that I present is very standard. I check some points to put the source as you asked :)
Fell free to comment and ressentem if you want :)
Thank you for doing that. In the end, there are a number of readers that will flag your Posts if you do not give citations in some form. It's a hard lesson to learn sometimes, especially if you have a whale downvote you for plagiarism because you didn't cite your sources. I've seen people that had $20 posts that were flagged down to nothing, so it's something to keep in mind.
I like the lessons and I think it will provide more visibility to the tag.
Thanks :) I'll pay more attention to that in the next posts :)