Programming Lessons: Let's learn a little of C# - Lesson 02
Hello Guys
Tow days ago I started a new topic. I wanna teach how to program a little. As you probably know I'm a software developer so I wanna bring some of the stuff that I do daily. With this topic I do not wanna do something hard to understand but the basic concepts of programming.
We'll start with a simple topics and the language that we'll use is C#.
About C#
C# is a simple, modern, general-purpose, object-oriented programming language developed by Microsoft within its .NET initiative led by Anders Hejlsberg. This tutorial covers basic C# programming and various advanced concepts related to C# programming language.
First of all, if you wanna try the examples that I'll provide you can use this site to program online C#. Alternatively, you can install the free version on Visual Studio, the best tool to program C# here.
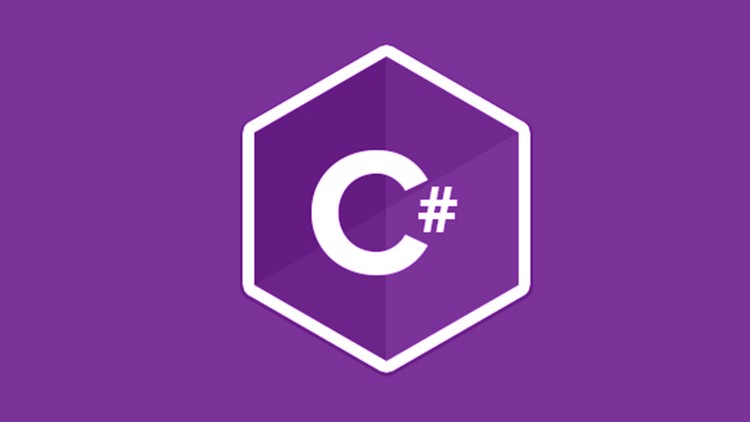
Lesson 02: Object-Oriented Programming Language
C# is an object-oriented programming language. In Object-Oriented Programming methodology, a program consists of several objects that interact with each other. The actions that an object may take are called methods. So, we create an object (a class) and inside of a class we create the variables and the methods to externalize the actions.
For example, let us consider the classic Rectangle object. It has attributes such as Length and Width. Let us look at implementation of a Rectangle class and discuss C# basic syntax:
Code
// Rectangle Class
using System;
namespace RectangleApplication
{
class Rectangle
{
// Variables
double length;
double width;
// First method - Details of length and width
public void AcceptDetails()
{
length = 5;
width = 4;
}
// Match calculation to produce the area of the rectangle. This method return a type Double*
public double CalculateArea()
{
return length * width;
}
// Code to display the Length, Width and the result of Area
public void Display()
{
Console.WriteLine("Rectangle Length: {0}", length);
Console.WriteLine("Rectangle Width: {0}", width);
Console.WriteLine("Rectangle Area: {0}", CalculateArea());
}
}
*More information here about this literal
// Main Class
class RectangleEx
{
static void Main(string[] args)
{
// Instantiate the object
Rectangle rectangle = new Rectangle();
// Call the method AcceptDetails
rectangle.AcceptDetails();
// Call the method display
rectangle.Display();
Console.ReadLine();
}
}
Console
> Rectangle Length: 5
> Rectangle Width: 4
> Rectangle Area: 20
Press a key to continue...
Hope you enjoy the first lesson :)
@criptomaster
Congratulations @criptomaster! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Not bad for a second post under my tag. Here's some advice that I hope you take seriously: cite your sources. I can assume the code is specifically yours, correct? If not, someone will end up fact-checking and marking you for plagiarism. Further to that, those indented explanations came from somewhere, so you need to give credit to the sources that you use before you are flagged.
Please take this into consideration if you continue to use this tag. I will overlook for the 3rd Post since it was already up before my comments.
Aside from that, good choice for the next lesson... what would they use it for?